filmov
tv
The Best Methods to Prepend Elements in a JavaScript Array
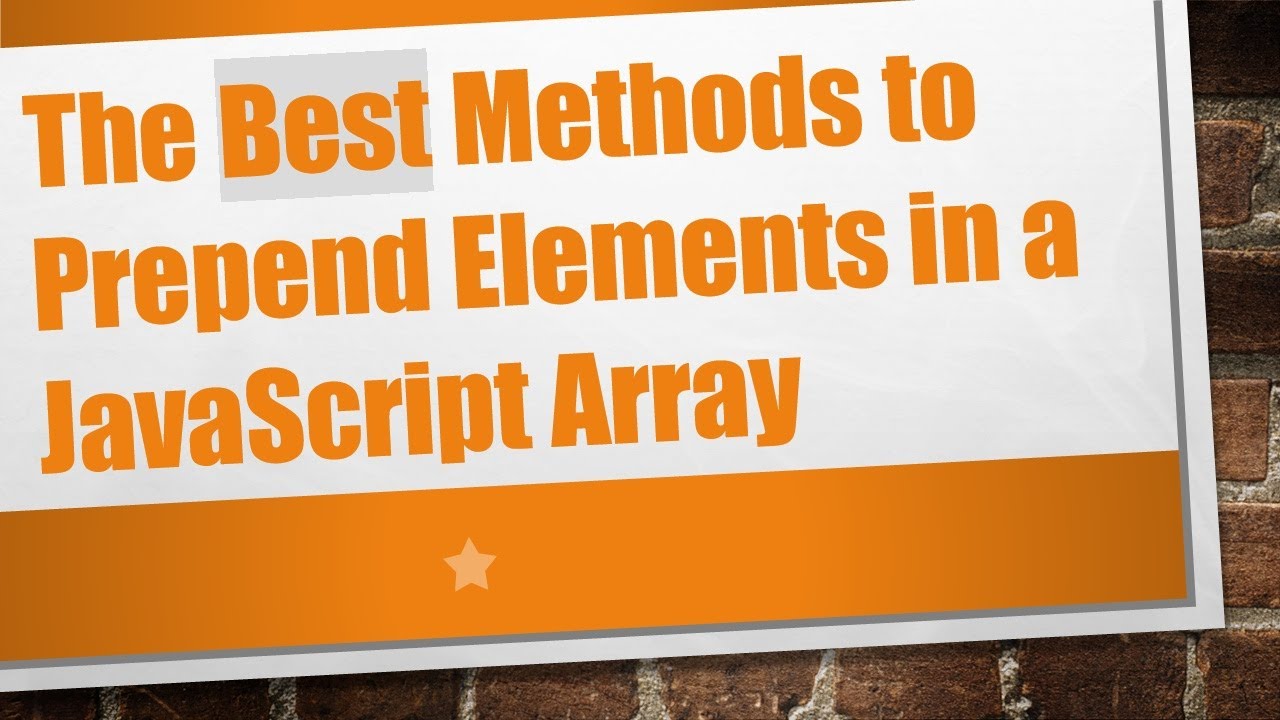
Показать описание
Unlock the secrets of efficiently prepending elements to arrays in JavaScript with and without cloning. Explore simple methods to enhance your code performance!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Best way to prepend an element to an array with and without cloning the original list
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
The Best Methods to Prepend Elements in a JavaScript Array
When working with arrays in JavaScript, you might find yourself in need of prepending an element to the beginning of an array. This is a common task but can pose challenges depending on whether you're allowing for the array to be modified directly or whether you need to clone the original array. In this post, we'll explore the most effective ways to achieve this, highlighting the pros and cons of each method and how they impact performance.
Understanding the Problem
Let’s start by understanding what it means to prepend an element to an array. Prepending refers to adding an item to the start of an array. As the array grows larger, the method of adding elements can significantly affect performance, especially in more complex applications where maintaining immutability is necessary.
The Challenge
Imagine we have an array of messages, and we want to add a new message at the start of this array. The challenge is twofold:
How do we do this efficiently?
How do we ensure the original array remains unchanged if required (for example, in frameworks that don't allow state mutations)?
Methods to Prepend Elements
We'll explore several methods to prepend elements, both without cloning the original list and with cloning to ensure the original array remains immutable. We’ll present the performance of each method as we go along.
Without Cloning the Original List
Using Spread Syntax
[[See Video to Reveal this Text or Code Snippet]]
Performance: 531 ops/s (99.5% slower than the fastest method)
Description: This syntax is concise, but performance suffers with large arrays.
Using concat()
[[See Video to Reveal this Text or Code Snippet]]
Performance: 5.6K ops/s (94.71% slower)
Description: This method is reasonably fast and creates a new array.
Using unshift()
[[See Video to Reveal this Text or Code Snippet]]
Performance: 105K ops/s (Fastest)
Description: This method modifies the array directly but is very efficient.
Using reverse()
[[See Video to Reveal this Text or Code Snippet]]
Performance: 4.9K ops/s (95.3% slower)
Description: This is a convoluted method and generally not recommended due to performance overhead.
With Cloning the Original List
For scenarios where state immutability is a must, we look at methods that clone the array before manipulating it.
Spread Syntax with Clone
[[See Video to Reveal this Text or Code Snippet]]
Performance: 472 ops/s (84.57% slower)
Using concat() with Clone
[[See Video to Reveal this Text or Code Snippet]]
Performance: 3.1K ops/s (Fastest among cloning methods)
Using unshift() on Clone
[[See Video to Reveal this Text or Code Snippet]]
Performance: 2.5K ops/s (19.7% slower)
Using reverse() on Clone
[[See Video to Reveal this Text or Code Snippet]]
Performance: 1.2K ops/s (61.14% slower)
Conclusion: Optimal Solution
Based on the performance of these methods, it is clear that using concat() appears to be the fastest while still allowing you to maintain the immutability of your data structure. In scenarios where direct mutation is acceptable, using unshift() is incredibly efficient but changes the original array.
In summary, consider the context you are coding in and whether or not you need to maintain the original state of your array. By knowing all available methods, you can choose the optimal one for your specific needs.
Whether you're building a simple application or developing a more complex state management solution, understanding how to prepend elements in an efficient manner will vastly improve your JavaScript coding experience.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Best way to prepend an element to an array with and without cloning the original list
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
The Best Methods to Prepend Elements in a JavaScript Array
When working with arrays in JavaScript, you might find yourself in need of prepending an element to the beginning of an array. This is a common task but can pose challenges depending on whether you're allowing for the array to be modified directly or whether you need to clone the original array. In this post, we'll explore the most effective ways to achieve this, highlighting the pros and cons of each method and how they impact performance.
Understanding the Problem
Let’s start by understanding what it means to prepend an element to an array. Prepending refers to adding an item to the start of an array. As the array grows larger, the method of adding elements can significantly affect performance, especially in more complex applications where maintaining immutability is necessary.
The Challenge
Imagine we have an array of messages, and we want to add a new message at the start of this array. The challenge is twofold:
How do we do this efficiently?
How do we ensure the original array remains unchanged if required (for example, in frameworks that don't allow state mutations)?
Methods to Prepend Elements
We'll explore several methods to prepend elements, both without cloning the original list and with cloning to ensure the original array remains immutable. We’ll present the performance of each method as we go along.
Without Cloning the Original List
Using Spread Syntax
[[See Video to Reveal this Text or Code Snippet]]
Performance: 531 ops/s (99.5% slower than the fastest method)
Description: This syntax is concise, but performance suffers with large arrays.
Using concat()
[[See Video to Reveal this Text or Code Snippet]]
Performance: 5.6K ops/s (94.71% slower)
Description: This method is reasonably fast and creates a new array.
Using unshift()
[[See Video to Reveal this Text or Code Snippet]]
Performance: 105K ops/s (Fastest)
Description: This method modifies the array directly but is very efficient.
Using reverse()
[[See Video to Reveal this Text or Code Snippet]]
Performance: 4.9K ops/s (95.3% slower)
Description: This is a convoluted method and generally not recommended due to performance overhead.
With Cloning the Original List
For scenarios where state immutability is a must, we look at methods that clone the array before manipulating it.
Spread Syntax with Clone
[[See Video to Reveal this Text or Code Snippet]]
Performance: 472 ops/s (84.57% slower)
Using concat() with Clone
[[See Video to Reveal this Text or Code Snippet]]
Performance: 3.1K ops/s (Fastest among cloning methods)
Using unshift() on Clone
[[See Video to Reveal this Text or Code Snippet]]
Performance: 2.5K ops/s (19.7% slower)
Using reverse() on Clone
[[See Video to Reveal this Text or Code Snippet]]
Performance: 1.2K ops/s (61.14% slower)
Conclusion: Optimal Solution
Based on the performance of these methods, it is clear that using concat() appears to be the fastest while still allowing you to maintain the immutability of your data structure. In scenarios where direct mutation is acceptable, using unshift() is incredibly efficient but changes the original array.
In summary, consider the context you are coding in and whether or not you need to maintain the original state of your array. By knowing all available methods, you can choose the optimal one for your specific needs.
Whether you're building a simple application or developing a more complex state management solution, understanding how to prepend elements in an efficient manner will vastly improve your JavaScript coding experience.