filmov
tv
Simplifying code with defaultdict in python
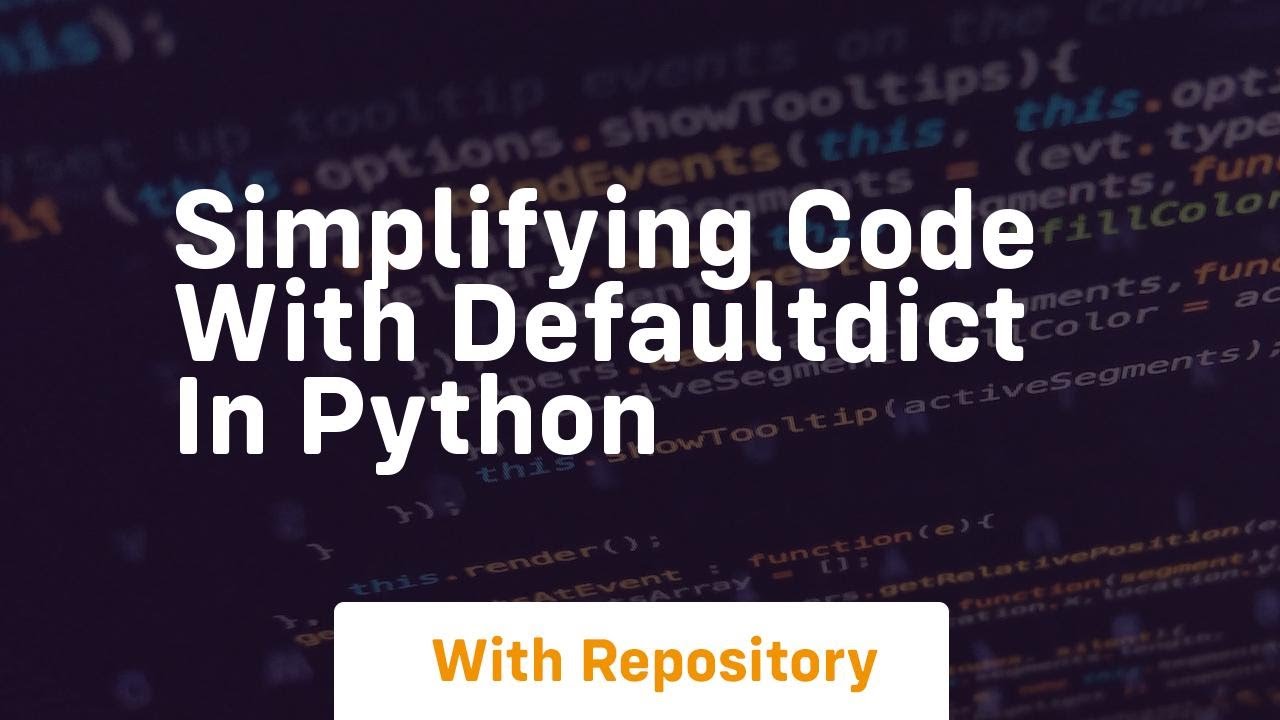
Показать описание
sure! the `defaultdict` is a subclass of the built-in `dict` class in python, which provides a convenient way to handle missing keys. using `defaultdict`, you can avoid key errors by providing a default value for non-existing keys, which simplifies your code significantly, especially when dealing with collections or counting items.
### what is `defaultdict`?
a `defaultdict` requires a factory function as its argument, which provides the default value for the dictionary when a key is accessed that does not exist. common factory functions include `list`, `int`, and `set`.
### usage scenarios
1. **counting occurrences of elements**: instead of initializing a dictionary with keys and values manually, you can use `defaultdict(int)` to count occurrences.
2. **grouping items**: use `defaultdict(list)` to group items together easily.
3. **setting default values**: use `defaultdict(str)` or any other type to ensure that all keys have a default value.
### example: counting occurrences
here's an example that demonstrates how to count the occurrences of each character in a string using `defaultdict`.
### output
### example: grouping items
here’s another example where we group items by their lengths using `defaultdict(list)`.
### output
### advantages of using `defaultdict`
1. **cleaner code**: reduces the need for checking if a key exists before updating its value.
2. **less boilerplate**: avoids repetitive code for initializing dictionary keys.
3. **improved readability**: makes the intention of the code clearer.
### conclusion
the `defaultdict` is a powerful tool in python that can help simplify your code when dealing with dictionaries. by providing default values, it reduces the chance of key errors and makes your code cleaner and easier to understand. whether you are counting items or grouping elements, `defaultdict` can significantly improve your coding experience.
...
#python code formatter
#python code online
#python code tester
#python code examples
#python code checker
python code formatter
python code online
python code tester
python code examples
python code checker
python code
python code generator
python coder
python code compiler
python code runner
python defaultdict vs dict
python defaultdict 0
python defaultdict to dict
python defaultdict
python defaultdict(set)
python defaultdict typing
python defaultdict lambda
python defaultdict default value
### what is `defaultdict`?
a `defaultdict` requires a factory function as its argument, which provides the default value for the dictionary when a key is accessed that does not exist. common factory functions include `list`, `int`, and `set`.
### usage scenarios
1. **counting occurrences of elements**: instead of initializing a dictionary with keys and values manually, you can use `defaultdict(int)` to count occurrences.
2. **grouping items**: use `defaultdict(list)` to group items together easily.
3. **setting default values**: use `defaultdict(str)` or any other type to ensure that all keys have a default value.
### example: counting occurrences
here's an example that demonstrates how to count the occurrences of each character in a string using `defaultdict`.
### output
### example: grouping items
here’s another example where we group items by their lengths using `defaultdict(list)`.
### output
### advantages of using `defaultdict`
1. **cleaner code**: reduces the need for checking if a key exists before updating its value.
2. **less boilerplate**: avoids repetitive code for initializing dictionary keys.
3. **improved readability**: makes the intention of the code clearer.
### conclusion
the `defaultdict` is a powerful tool in python that can help simplify your code when dealing with dictionaries. by providing default values, it reduces the chance of key errors and makes your code cleaner and easier to understand. whether you are counting items or grouping elements, `defaultdict` can significantly improve your coding experience.
...
#python code formatter
#python code online
#python code tester
#python code examples
#python code checker
python code formatter
python code online
python code tester
python code examples
python code checker
python code
python code generator
python coder
python code compiler
python code runner
python defaultdict vs dict
python defaultdict 0
python defaultdict to dict
python defaultdict
python defaultdict(set)
python defaultdict typing
python defaultdict lambda
python defaultdict default value