filmov
tv
Understanding the defaultdict of defaultdict in Python
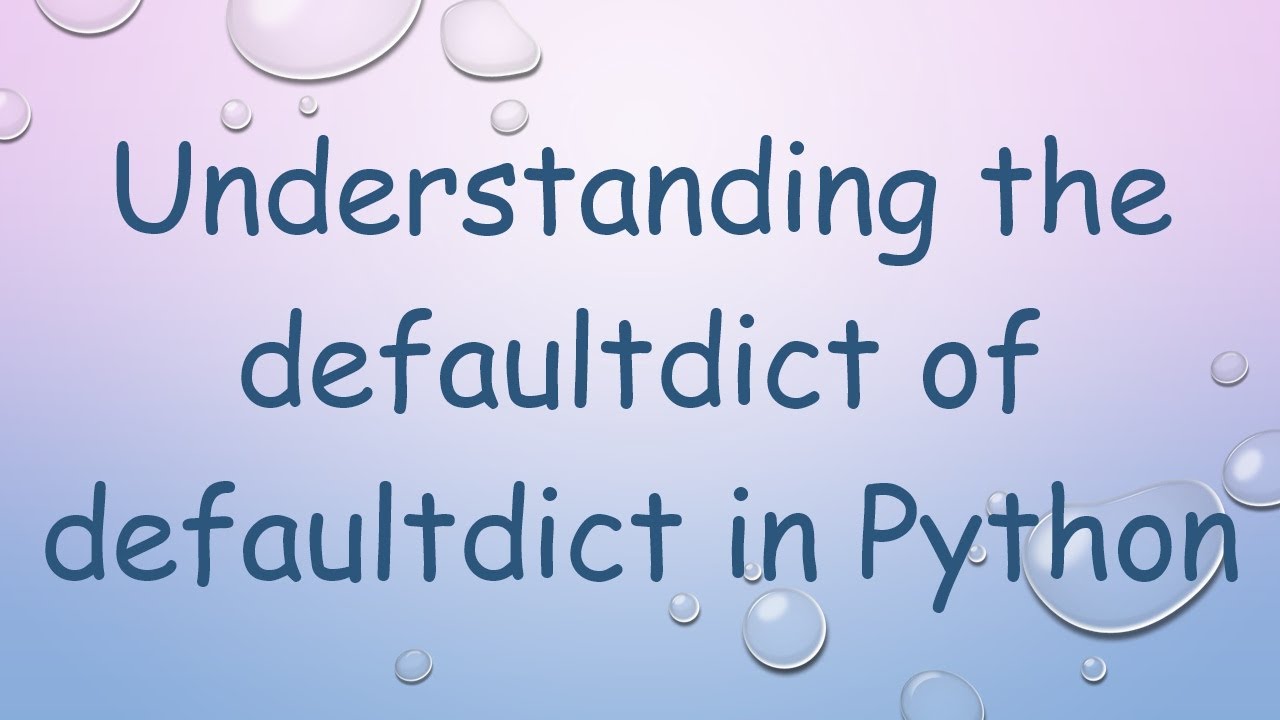
Показать описание
Learn about the defaultdict of defaultdict in Python, its benefits, and how to use it effectively to simplify nested dictionary operations.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
In Python, dictionaries are a fundamental data structure used to store key-value pairs. However, working with nested dictionaries can sometimes be cumbersome, especially when initializing or updating them. The defaultdict from the collections module offers a convenient solution to this problem, particularly when using a defaultdict of defaultdicts.
What is a defaultdict?
A defaultdict is a subclass of the built-in dict class. It overrides one method and adds one writable instance variable. The overridden method is __missing__, which provides a default value for a nonexistent key. The default_factory attribute is used to specify the default type or value.
Creating a defaultdict of defaultdict
When working with nested dictionaries, you often need to ensure that each nested dictionary is initialized properly. Using a defaultdict of defaultdict can simplify this process.
Basic Example
Here's a simple example to illustrate the concept:
[[See Video to Reveal this Text or Code Snippet]]
In this example, nested_dict is a defaultdict where each value is another defaultdict with int as the default factory. This means that any missing key will automatically be initialized to a defaultdict(int), and any missing subkey in those nested dictionaries will default to 0.
Benefits
Automatic Initialization: One of the biggest advantages is the automatic initialization of nested dictionaries. You don't need to check if a key exists before adding or updating values.
Cleaner Code: Your code becomes cleaner and more readable without the need for multiple if statements or manual dictionary initializations.
Reduced Errors: By automating the initialization, you reduce the risk of KeyError exceptions, making your code more robust.
Advanced Usage
You can nest defaultdict to any level of complexity required by your application. For instance, you might need a defaultdict of defaultdict of defaultdict:
[[See Video to Reveal this Text or Code Snippet]]
In this scenario, three_level_dict is a 3-level nested defaultdict, where the innermost dictionary defaults to an integer.
Conclusion
The defaultdict of defaultdict pattern in Python is a powerful tool for handling nested dictionaries efficiently. It simplifies the initialization process, enhances code readability, and reduces the potential for errors. Whether you're dealing with simple or complex nested data structures, leveraging defaultdict can significantly improve your code's maintainability and robustness.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
In Python, dictionaries are a fundamental data structure used to store key-value pairs. However, working with nested dictionaries can sometimes be cumbersome, especially when initializing or updating them. The defaultdict from the collections module offers a convenient solution to this problem, particularly when using a defaultdict of defaultdicts.
What is a defaultdict?
A defaultdict is a subclass of the built-in dict class. It overrides one method and adds one writable instance variable. The overridden method is __missing__, which provides a default value for a nonexistent key. The default_factory attribute is used to specify the default type or value.
Creating a defaultdict of defaultdict
When working with nested dictionaries, you often need to ensure that each nested dictionary is initialized properly. Using a defaultdict of defaultdict can simplify this process.
Basic Example
Here's a simple example to illustrate the concept:
[[See Video to Reveal this Text or Code Snippet]]
In this example, nested_dict is a defaultdict where each value is another defaultdict with int as the default factory. This means that any missing key will automatically be initialized to a defaultdict(int), and any missing subkey in those nested dictionaries will default to 0.
Benefits
Automatic Initialization: One of the biggest advantages is the automatic initialization of nested dictionaries. You don't need to check if a key exists before adding or updating values.
Cleaner Code: Your code becomes cleaner and more readable without the need for multiple if statements or manual dictionary initializations.
Reduced Errors: By automating the initialization, you reduce the risk of KeyError exceptions, making your code more robust.
Advanced Usage
You can nest defaultdict to any level of complexity required by your application. For instance, you might need a defaultdict of defaultdict of defaultdict:
[[See Video to Reveal this Text or Code Snippet]]
In this scenario, three_level_dict is a 3-level nested defaultdict, where the innermost dictionary defaults to an integer.
Conclusion
The defaultdict of defaultdict pattern in Python is a powerful tool for handling nested dictionaries efficiently. It simplifies the initialization process, enhances code readability, and reduces the potential for errors. Whether you're dealing with simple or complex nested data structures, leveraging defaultdict can significantly improve your code's maintainability and robustness.