filmov
tv
Handling json arrays returned from asp net web services with jquery
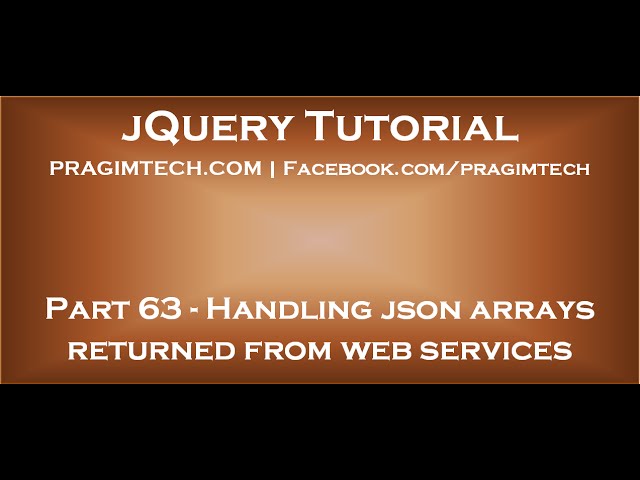
Показать описание
Link for all dot net and sql server video tutorial playlists
Link for slides, code samples and text version of the video
Healthy diet is very important both for the body and mind. If you like Aarvi Kitchen recipes, please support by sharing, subscribing and liking our YouTube channel. Hope you can help.
When we click Get All Employees button, we want to retrieve all the Employees from the database table and display on the page using jQuery.
This is continuation to Part 62, please watch Part 62 before proceeding.
using System;
using System.Collections.Generic;
using System.Configuration;
using System.Data.SqlClient;
using System.Web.Script.Serialization;
using System.Web.Script.Services;
using System.Web.Services;
namespace Demo
{
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
[System.Web.Script.Services.ScriptService]
public class EmployeeService : System.Web.Services.WebService
{
[WebMethod]
public void GetAllEmployees()
{
List<Employee> listEmployees = new List<Employee>();
string cs = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(cs))
{
SqlCommand cmd = new SqlCommand("Select * from tblEmployee", con);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
Employee employee = new Employee();
employee.ID = Convert.ToInt32(rdr["Id"]);
employee.Name = rdr["Name"].ToString();
employee.Gender = rdr["Gender"].ToString();
employee.Salary = Convert.ToInt32(rdr["Salary"]);
listEmployees.Add(employee);
}
}
JavaScriptSerializer js = new JavaScriptSerializer();
Context.Response.Write(js.Serialize(listEmployees));
}
}
}
Replace < with LESSTHAN symbol and > with GREATERTHAN symbol
<html>
<head>
<script type="text/javascript">
$(document).ready(function () {
$('#btnGetAllEmployees').click(function () {
$.ajax({
dataType: "json",
method: 'post',
success: function (data) {
var employeeTable = $('#tblEmployee tbody');
$(data).each(function (index, emp) {
+ emp.Name + '</td><td>' + emp.Gender
+ '</td><td>' + emp.Salary + '</td></tr>');
});
},
error: function (err) {
alert(err);
}
});
});
});
</script>
</head>
<body style="font-family:Arial">
<input type="button" id="btnGetAllEmployees" value="Get All Employees" />
<br /><br />
<table id="tblEmployee" border="1" style="border-collapse:collapse">
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Gender</th>
<th>Salary</th>
</tr>
</thead>
<tbody></tbody>
</table>
</body>
</html>
Link for slides, code samples and text version of the video
Healthy diet is very important both for the body and mind. If you like Aarvi Kitchen recipes, please support by sharing, subscribing and liking our YouTube channel. Hope you can help.
When we click Get All Employees button, we want to retrieve all the Employees from the database table and display on the page using jQuery.
This is continuation to Part 62, please watch Part 62 before proceeding.
using System;
using System.Collections.Generic;
using System.Configuration;
using System.Data.SqlClient;
using System.Web.Script.Serialization;
using System.Web.Script.Services;
using System.Web.Services;
namespace Demo
{
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
[System.Web.Script.Services.ScriptService]
public class EmployeeService : System.Web.Services.WebService
{
[WebMethod]
public void GetAllEmployees()
{
List<Employee> listEmployees = new List<Employee>();
string cs = ConfigurationManager.ConnectionStrings["DBCS"].ConnectionString;
using (SqlConnection con = new SqlConnection(cs))
{
SqlCommand cmd = new SqlCommand("Select * from tblEmployee", con);
con.Open();
SqlDataReader rdr = cmd.ExecuteReader();
while (rdr.Read())
{
Employee employee = new Employee();
employee.ID = Convert.ToInt32(rdr["Id"]);
employee.Name = rdr["Name"].ToString();
employee.Gender = rdr["Gender"].ToString();
employee.Salary = Convert.ToInt32(rdr["Salary"]);
listEmployees.Add(employee);
}
}
JavaScriptSerializer js = new JavaScriptSerializer();
Context.Response.Write(js.Serialize(listEmployees));
}
}
}
Replace < with LESSTHAN symbol and > with GREATERTHAN symbol
<html>
<head>
<script type="text/javascript">
$(document).ready(function () {
$('#btnGetAllEmployees').click(function () {
$.ajax({
dataType: "json",
method: 'post',
success: function (data) {
var employeeTable = $('#tblEmployee tbody');
$(data).each(function (index, emp) {
+ emp.Name + '</td><td>' + emp.Gender
+ '</td><td>' + emp.Salary + '</td></tr>');
});
},
error: function (err) {
alert(err);
}
});
});
});
</script>
</head>
<body style="font-family:Arial">
<input type="button" id="btnGetAllEmployees" value="Get All Employees" />
<br /><br />
<table id="tblEmployee" border="1" style="border-collapse:collapse">
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Gender</th>
<th>Salary</th>
</tr>
</thead>
<tbody></tbody>
</table>
</body>
</html>
Комментарии