filmov
tv
Data Structures: Solve 'Find the Running Median' Using Heaps
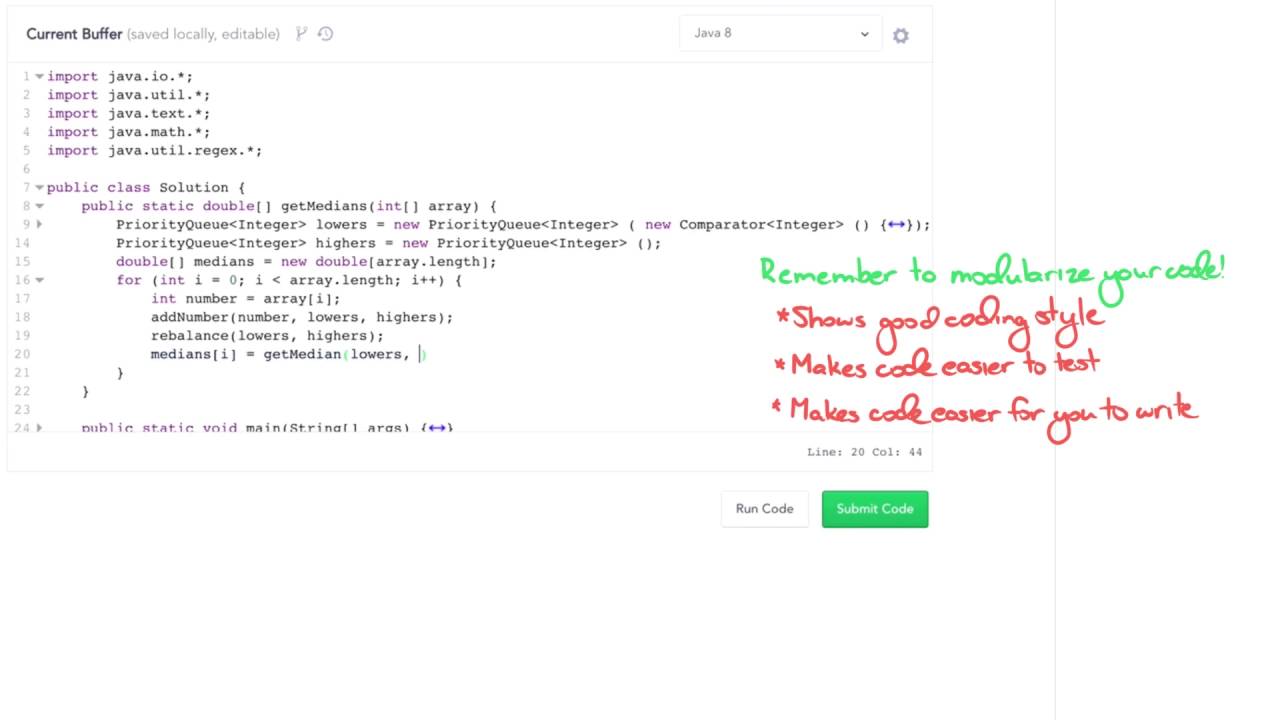
Показать описание
Learn how to solve 'Finding the Running Median' using heaps. This video is a part of HackerRank's Cracking The Coding Interview Tutorial with Gayle Laakmann McDowell.
Data Structures: Solve 'Find the Running Median' Using Heaps
Data Structures: Solve 'Contacts' Using Tries
Top 6 Coding Interview Concepts (Data Structures & Algorithms)
Union Find in 5 minutes — Data Structures & Algorithms
I gave 127 interviews. Top 5 Algorithms they asked me.
Stack Data Structure Tutorial – Solve Coding Challenges
Introduction to Linked Lists (Data Structures & Algorithms #5)
Asymptotic Analysis (Solved Problem 1)
Master Python Data Structures & Algorithms in 1 Hour
Top 5 Most Common Graph Algorithms for Coding Interviews
Introduction to Big O Notation and Time Complexity (Data Structures & Algorithms #7)
How to Solve Problems with Data Structures and Algorithms (Think Like a Programmer)
Top 7 Algorithms for Coding Interviews Explained SIMPLY
How I Got Good at Coding Interviews
Data Structures Easy to Advanced Course - Full Tutorial from a Google Engineer
Calculating Time Complexity | Data Structures and Algorithms| GeeksforGeeks
Data Structures & Algorithms #1 - What Are Data Structures?
Algorithms and Data Structures Tutorial - Full Course for Beginners
Binary Tree Algorithms for Technical Interviews - Full Course
Binary Search examples | Successful search | Design & Algorithms | Lec-13 | Bhanu Priya
Graph Algorithms for Technical Interviews - Full Course
Data Structures and Algorithms for Beginners
5.1 Graph Traversals - BFS & DFS -Breadth First Search and Depth First Search
Big O notation - Data Structures & Algorithms Tutorial #2 | Measuring time complexity
Комментарии