filmov
tv
Top 7 Algorithms for Coding Interviews Explained SIMPLY
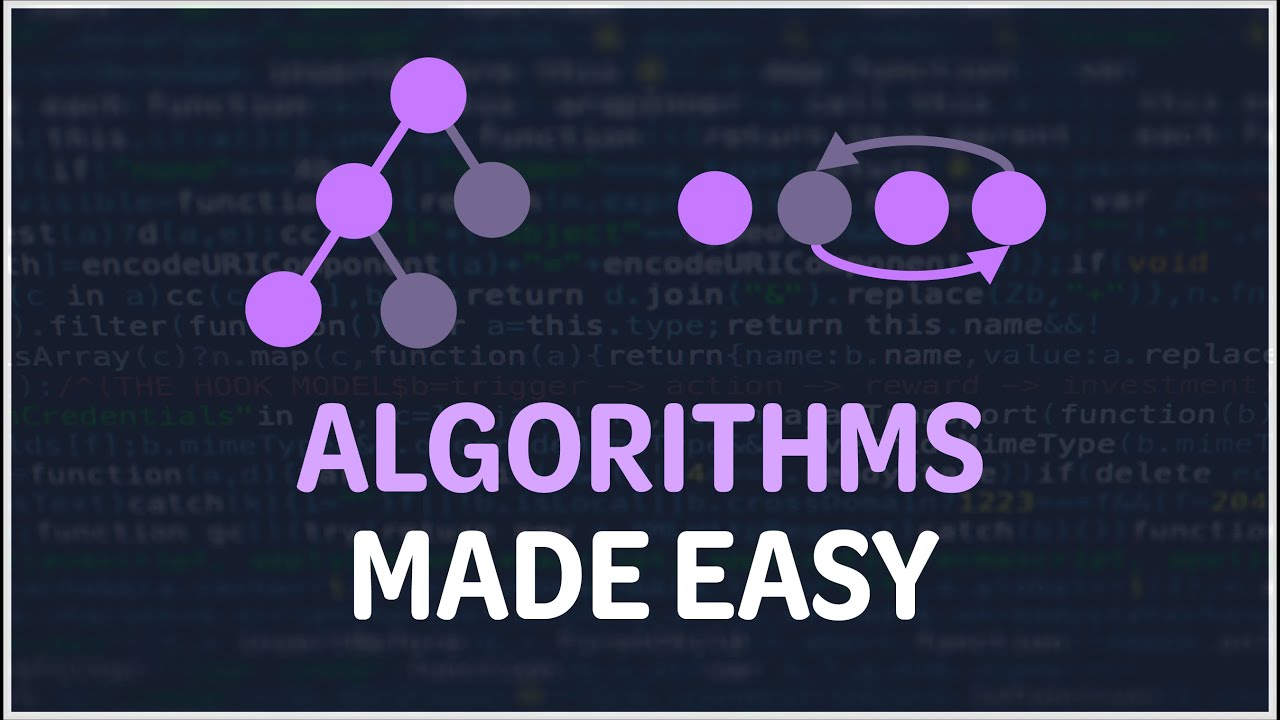
Показать описание
Today we'll be covering the 7 most important algorithms you need to ace your coding interviews and land a job as a software engineer. We'll be showing visualizations, common uses, and time complexity. Hope you enjoy!
📘 Chapters
0:00 - Intro
0:57 - Binary Search
3:43 - Depth-First Search
6:39 - Breadth-First Search
9:13 - Insertion Sort
10:57 - Merge Sort
12:52 - Quick Sort
15:57 - Greedy
🔗 Resources
🎵 Music
Music Courtsey of Epidemic Sound
Wizzle - Guustavv
Slapperoni Pizza - Jobii
Unfettered - Jobii
At My Own Pace - Sarah, the Illstrumentalist
Deadlines - Dylan Sitts
Sleepy + Hungry - baegel
Tea Tree - Jobii
Taargus - Jobii
Pick Up Your Phone Please - baegel
Brontosaurus - Dylan Sitts
#coding #softwareengineer #algorithm
📘 Chapters
0:00 - Intro
0:57 - Binary Search
3:43 - Depth-First Search
6:39 - Breadth-First Search
9:13 - Insertion Sort
10:57 - Merge Sort
12:52 - Quick Sort
15:57 - Greedy
🔗 Resources
🎵 Music
Music Courtsey of Epidemic Sound
Wizzle - Guustavv
Slapperoni Pizza - Jobii
Unfettered - Jobii
At My Own Pace - Sarah, the Illstrumentalist
Deadlines - Dylan Sitts
Sleepy + Hungry - baegel
Tea Tree - Jobii
Taargus - Jobii
Pick Up Your Phone Please - baegel
Brontosaurus - Dylan Sitts
#coding #softwareengineer #algorithm
Top 7 Algorithms for Coding Interviews Explained SIMPLY
I gave 127 interviews. Top 5 Algorithms they asked me.
Top 6 Coding Interview Concepts (Data Structures & Algorithms)
Top 5 Algorithms for Coding Interviews
Top 5 Most Common Graph Algorithms for Coding Interviews
Top 7 algorithms for Interviews
Top 7 Algorithms for Coding Interviews | 98% Chance in Interviews | DSA interview questions answer 🔥...
Top 5 algorithms for interviews
COMP20003 Algorithms and Data Structures Revision Workshop
Top 10 Javascript Algorithms to Prepare for Coding Interviews
The Best Book To Learn Algorithms From For Computer Science
My Top 3 Tips for Learning Data Structures & Algorithms
Top Algorithms every Programmer should know #javascripttutorial #learntocode
10 Common Coding Interview Problems - Solved!
Here's the Top 9 Algorithms for Coding Interviews asked 98% of the time | Check the description
How I'd Learn Data Structures & Algorithms For Free
How I mastered Data Structures and Algorithms #dsa #codinginterview #leetcode
The BEST Advice For Learning Data Structures and Algorithms Ft. Sandeep Jain, Founder of GFG
Fastest way to learn Data Structures and Algorithms
9 Most Important Algorithms For Coding Interviews. #algorithm #coding #interview
Top Algorithms for The Coding Interview(for Software Engineers)|Coding Interview Tips |Coding Ninjas
Top 10 Algorithms For Coding Interviews | Important Algorithms For Software Engineers | Simplilearn
The 10 Most Important Concepts For Coding Interviews (algorithms and data structures)
Top 6 Load Balancing Algorithms Every Developer Should Know
Комментарии