filmov
tv
ValueError invalid literal for int with base 10 NewFilmLikes in Python
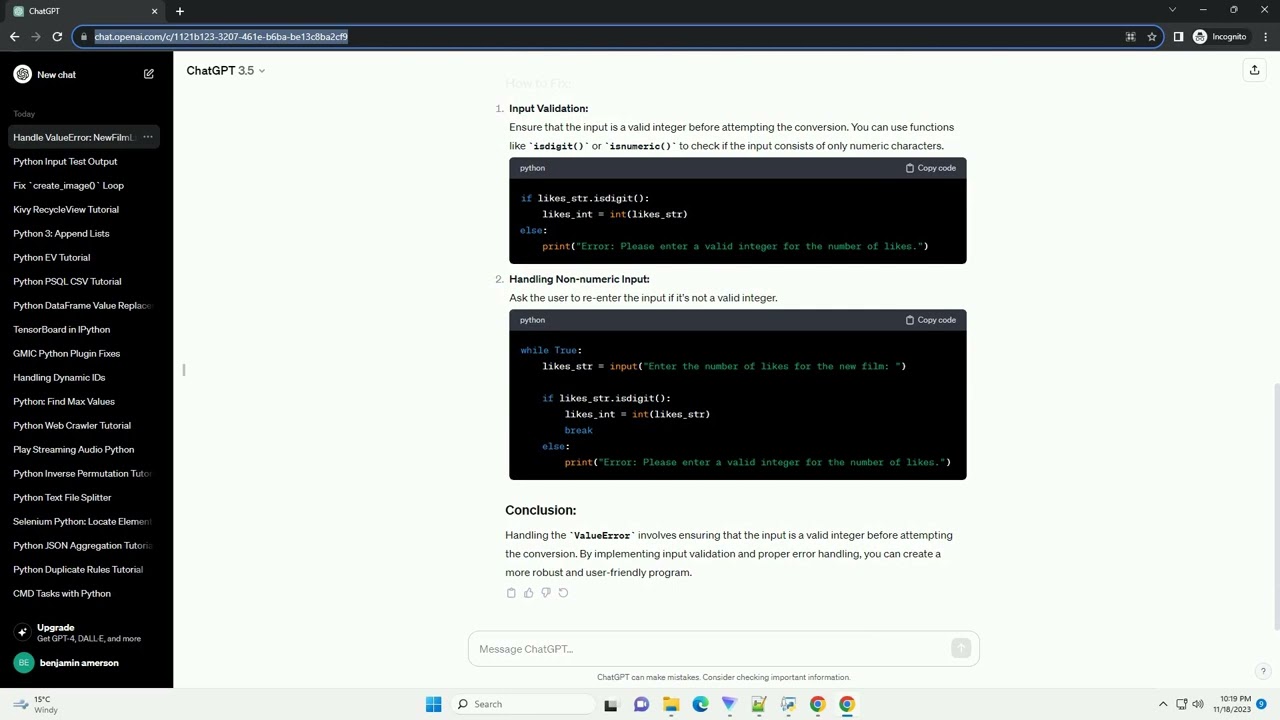
Показать описание
Title: Handling ValueError: Invalid literal for int() with base 10 in Python
Introduction:
When working with Python, you might encounter a ValueError with the message "invalid literal for int() with base 10." This error occurs when you try to convert a string to an integer using the int() function, but the string is not a valid integer representation.
In this tutorial, we'll explore the reasons behind this error and discuss how to handle it gracefully with code examples.
Common Causes of the Error:
Non-Numeric Input:
The int() function expects a string containing a valid numeric representation, such as '123' or '-45'. If the string contains non-numeric characters, the conversion will fail.
Unexpected Input Format:
Ensure that the input string doesn't include any leading or trailing whitespaces, as they can also lead to this error. Additionally, check for unexpected characters within the string.
Code Example:
Let's examine a simple example that triggers the ValueError:
Explanation:
Handling the Error:
To handle this error, you can use a try-except block to catch the ValueError and take appropriate action, such as providing a default value or displaying a user-friendly error message.
In this revised example, the function provides a clear error message when an invalid input is encountered.
Conclusion:
Handling ValueError: invalid literal for int() with base 10 is crucial when working with user inputs or external data. By using a try-except block, you can gracefully handle invalid conversions and improve the robustness of your Python programs.
ChatGPT
Certainly! Let's dive into understanding and handling the ValueError: invalid literal for int() with base 10: 'NewFilmLikes' in Python.
The error message indicates that there's an attempt to convert a string ('NewFilmLikes') to an integer using the int() function, but the string doesn't represent a valid integer in base 10. This usually occurs when you're expecting a numeric input, but the provided value is not a number.
This error often arises when reading input from a user or a file, and you expect a numerical value, but the input provided is a string that cannot be converted to an integer.
Let's consider a simple example where the user is asked to input the number of likes for a new film:
Input Validation:
Ensure that the input is a valid integer before attempting the conversion. You can use functions like isdigit() or isnumeric() to check if the input consists of only numeric characters.
Handling Non-numeric
Introduction:
When working with Python, you might encounter a ValueError with the message "invalid literal for int() with base 10." This error occurs when you try to convert a string to an integer using the int() function, but the string is not a valid integer representation.
In this tutorial, we'll explore the reasons behind this error and discuss how to handle it gracefully with code examples.
Common Causes of the Error:
Non-Numeric Input:
The int() function expects a string containing a valid numeric representation, such as '123' or '-45'. If the string contains non-numeric characters, the conversion will fail.
Unexpected Input Format:
Ensure that the input string doesn't include any leading or trailing whitespaces, as they can also lead to this error. Additionally, check for unexpected characters within the string.
Code Example:
Let's examine a simple example that triggers the ValueError:
Explanation:
Handling the Error:
To handle this error, you can use a try-except block to catch the ValueError and take appropriate action, such as providing a default value or displaying a user-friendly error message.
In this revised example, the function provides a clear error message when an invalid input is encountered.
Conclusion:
Handling ValueError: invalid literal for int() with base 10 is crucial when working with user inputs or external data. By using a try-except block, you can gracefully handle invalid conversions and improve the robustness of your Python programs.
ChatGPT
Certainly! Let's dive into understanding and handling the ValueError: invalid literal for int() with base 10: 'NewFilmLikes' in Python.
The error message indicates that there's an attempt to convert a string ('NewFilmLikes') to an integer using the int() function, but the string doesn't represent a valid integer in base 10. This usually occurs when you're expecting a numeric input, but the provided value is not a number.
This error often arises when reading input from a user or a file, and you expect a numerical value, but the input provided is a string that cannot be converted to an integer.
Let's consider a simple example where the user is asked to input the number of likes for a new film:
Input Validation:
Ensure that the input is a valid integer before attempting the conversion. You can use functions like isdigit() or isnumeric() to check if the input consists of only numeric characters.
Handling Non-numeric