filmov
tv
Programming Interview 49: Print Longest Increasing Subsequence (LIS) using Dynamic Programming
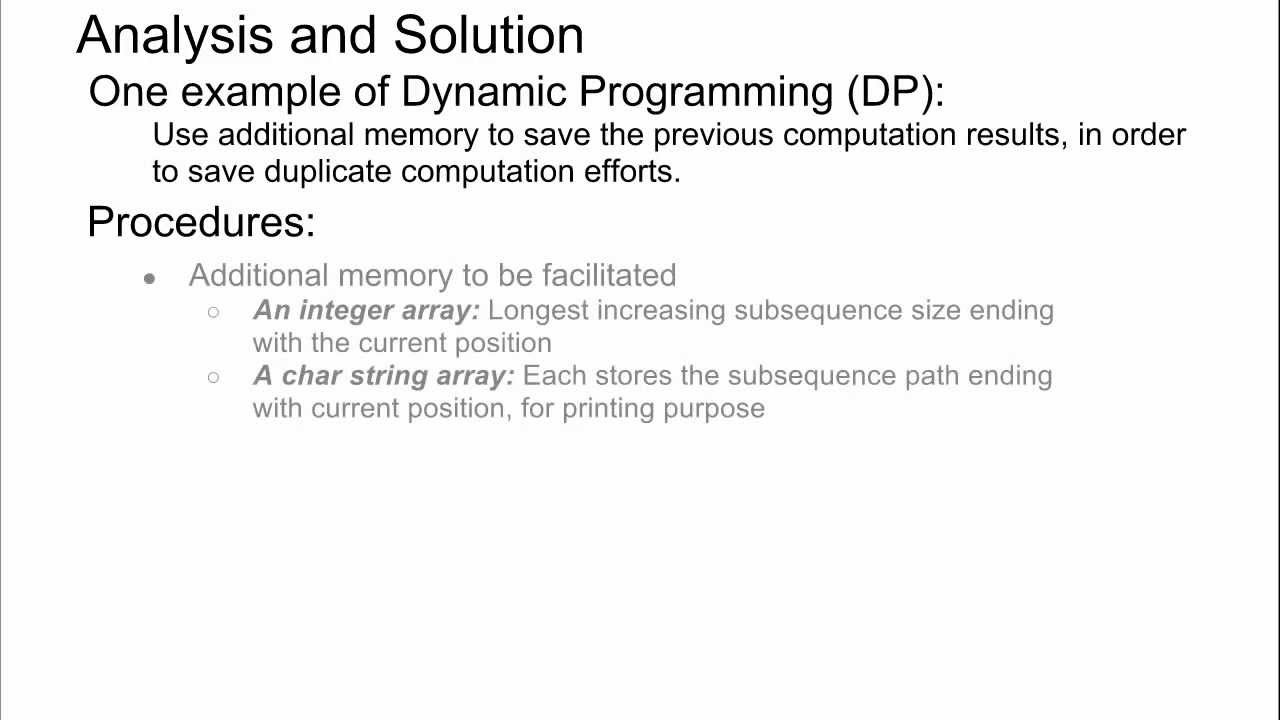
Показать описание
Step by step to crack Programming Interview question 49: Print Longest Increasing Subsequence (LIS) using Dynamic Programming
e.g. (2,6,4,5,1,3), the LIS = (2,4,5)
Please notice: The increasing subsequence does not have to be contiguous.
Analysis:
1. One example of Dynamic Programming (DP):
1.1. Use additional memory to save the previous computation results, in order to save duplicate computation efforts.
Procedures:
1. Additional memory to be facilitated
1.1. An integer array: Longest increasing subsequence size ending with the current position
1.2. A char string array: Each stores the subsequence path ending
2. For each new position to be processed
2.1. Check all previous positions to see how to append the current position to make the increasing subsequence length the maximal
2.2. The current position value should be larger than previous one
2.3. The previous_Size+1 is larger than current size
Please note: this O(N^2) time-complexity DP solution is not the best time-efficient solution. Google the O(nLogn) solution yourself.
e.g. (2,6,4,5,1,3), the LIS = (2,4,5)
Please notice: The increasing subsequence does not have to be contiguous.
Analysis:
1. One example of Dynamic Programming (DP):
1.1. Use additional memory to save the previous computation results, in order to save duplicate computation efforts.
Procedures:
1. Additional memory to be facilitated
1.1. An integer array: Longest increasing subsequence size ending with the current position
1.2. A char string array: Each stores the subsequence path ending
2. For each new position to be processed
2.1. Check all previous positions to see how to append the current position to make the increasing subsequence length the maximal
2.2. The current position value should be larger than previous one
2.3. The previous_Size+1 is larger than current size
Please note: this O(N^2) time-complexity DP solution is not the best time-efficient solution. Google the O(nLogn) solution yourself.
Programming Interview 49: Print Longest Increasing Subsequence (LIS) using Dynamic Programming
Group Anagrams - Categorize Strings by Count - Leetcode 49
Interview Problem (Dynamic Programming - Longest Increasing Subsequence) Amazon, Google, Facebook
Programming Interview Question: Longest Common Subsequence Dynamic Programming
Programming Interview Question: Longest Increasing Subsequence nlogn
Programming Interview 16: Find Largest subsequent sum in array in O(N) time
Programming Interview 48: Compute Largest Contiguous Subsequent Product (LSP) in Linear Time
Coding Interview Question - Python - Find Longest Substring In A String
Selenium Interview || Java Program to find the longest string in the array of strings
Programming Interview : Dynamic Programming :LIS, Longest Increasing Subsequence
Group anagrams | Leetcode #49
Java Interview Questions ||Longest Substring Without Repeating Characters| #programmingclass #java
Coding Interview Tutorial 32: Longest Continuous Increasing Subsequence [LeetCode]
Java Program to find longest substring without repetition| Amazon coding interview questions
Find longest length number in a string Java coding interview question
Programming Interview 39: Find Largest Rectangle Size in a Histogram in linear time
Longest Increasing Subsequence NLogN || Code in C# || Coding Interview Questions
Longest Increasing Subsequence (Dynamic Programming)
[GOOGLE INTERVIEW QUESTION] Largest Component Size Common Factor | LeetCode | DSU Solution Explained
Longest Consecutive Sequence (LeetCode 128) | Full solution quick and easy explanation | Interviews
23 Printing Longest common subsequence
How to find largest number in an array using Python| Python interview questions
'Why I Fire People Every Day' - Warren Buffett
Programming Interview 36: Find (kth largest) Kth item from array after sorting in ascending order
Комментарии