filmov
tv
Programming Interview 16: Find Largest subsequent sum in array in O(N) time
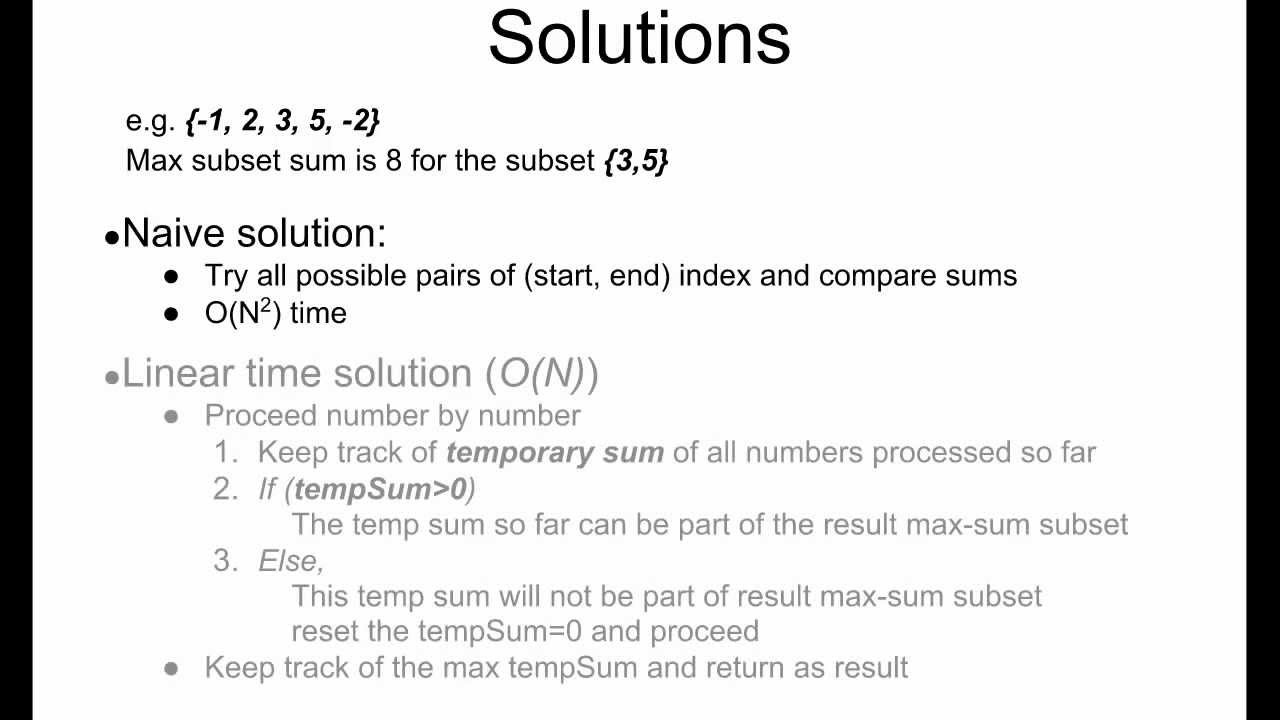
Показать описание
Step by step to crack Programming Interview questions 16: Find the maximum sum of a consecutive subset in an array in O(N) time
Example:
(-1, 2, 3, 5, -2) Max subset sum is 8 for the subset (3,5)
Solution:
1. Proceed number by number
2. Keep track of temporary sum of all numbers processed so far
2.1. If (tempSum is larger than 0)
The temp sum so far can be part of the result max-sum subset
2.2. Else,
This temp sum will not be part of result max-sum subset
reset the tempSum to 0 and proceed
3. Keep track of the max tempSum and return as result
Example:
(-1, 2, 3, 5, -2) Max subset sum is 8 for the subset (3,5)
Solution:
1. Proceed number by number
2. Keep track of temporary sum of all numbers processed so far
2.1. If (tempSum is larger than 0)
The temp sum so far can be part of the result max-sum subset
2.2. Else,
This temp sum will not be part of result max-sum subset
reset the tempSum to 0 and proceed
3. Keep track of the max tempSum and return as result
Programming Interview 16: Find Largest subsequent sum in array in O(N) time
Coding Interview 07: Find Largest sum of digits of prime numbers in series
Microsoft Coding Interview Question - Valid Perfect Square - Leetcode 367
Dynamic Programming Interview Question #1 - Find Sets Of Numbers That Add Up To 16
Top 6 Coding Interview Concepts (Data Structures & Algorithms)
3Sum Closest - LeetCode 16 - Coding Interview Questions
Java Coding Interview Hack: Find the Second Largest Element! 🥈✨
Coding Interview for Data Engineer: find two max number in given array
3 Coding Interview Mistakes
Top 60 Programming interview questions with answer - CRACK INTERVIEW
Coding rounds in Google Interview?
Apple Coding Interview Question! | Leetcode 1207 - Unique Number of Occurrences
Top 10 mistakes candidates make in the coding Interviews!
Top 6 Coding Interview Patterns
Top 5 Mistakes to Avoid in Coding Interviews
Amazon Coding Interview Question - K Closest Points to the Origin
Build 16 Medium/Hard JavaScript projects for live coding Interview rounds (Course Preview)
Python Programming Interview Question - Find Longest Word
uc berkeley students tell their #softwareengineer #intern #salary #coding #interview #tech #shorts
Coding Interview Problem: Largest Rectangle in a Histogram
C Programming-Predict the Output-16#interview#coding#placement#programming#c#preparation#it#java#job
Top 5 Dynamic Programming Patterns for Coding Interviews - For Beginners
CODING INTERVIEW STEP BY STEP GUIDE | FOLLOW THIS ALGORITHM FOR SUCCESS IN A TECHNICAL INTERVIEW
Google Coding Interview With A Competitive Programmer
Комментарии