filmov
tv
Algorithms: Solve 'Ice Cream Parlor' Using Binary Search
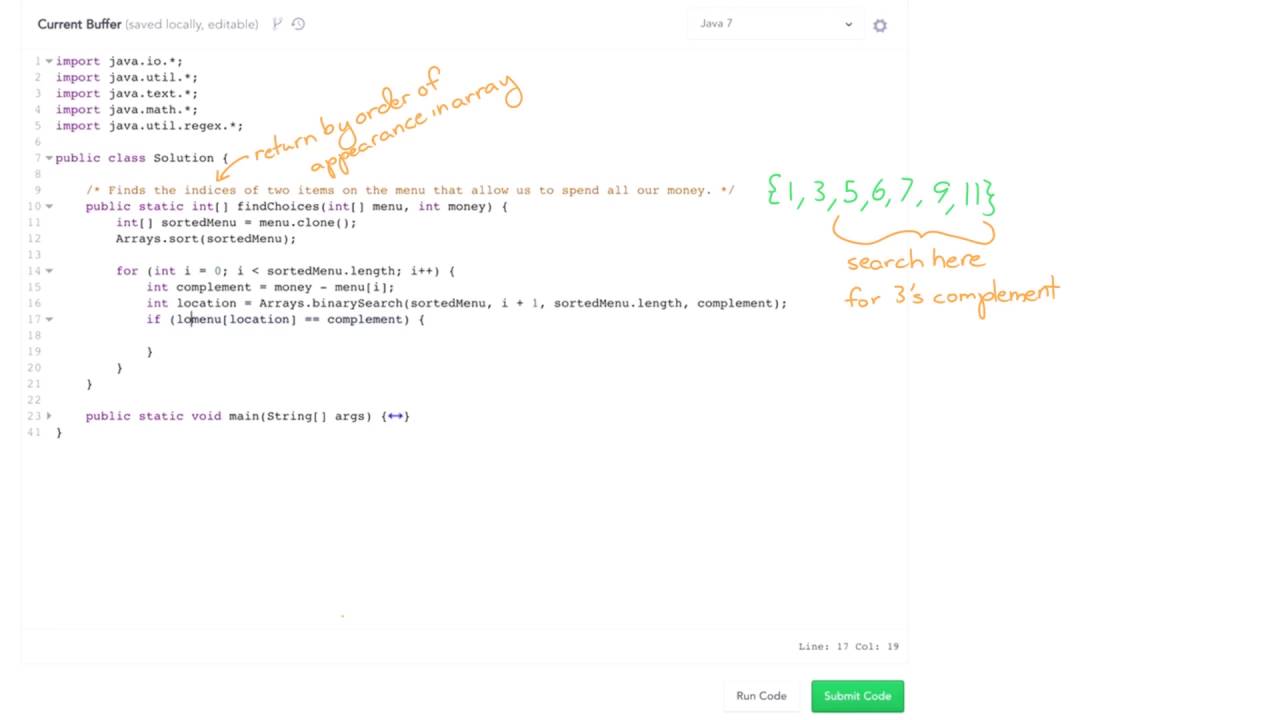
Показать описание
Learn how to solve the 'Ice Cream Parlor' using binary search algorithm. This video is a part of HackerRank's Cracking The Coding Interview Tutorial with Gayle Laakmann McDowell.
Algorithms: Solve 'Ice Cream Parlor' Using Binary Search
Algorithms Solve 'Ice Cream Parlor' Using Binary Search
Java Search Algorithm Ice Cream Parlor
Ice cream parlor
Ice Cream Parlor HackerRank solution | Two Sum LeetCode Solution
Ice cream parlor hackerrank solution in C @ BE A GEEK | Hindi |
ICE CREAM PARLOR HACKERRANK | SOLUTION | C++ | HINGLISH
Icecream Parlor | HackerRank Problem Solving | Ep-13 | Tamil | code io
Algorithms: Solve 'Connected Cells' Using DFS
Debugging 101: Replace print() with icecream ic()
Algorithms: Solve 'Lonley Integer' Using Bit Manipulation
Algorithms: Solve 'Coin Change' Using Memoization and DP
Kaiser's Ice Cream Parlor hires workers to produce milk shakes. The market for milk shakes is p...
Algorithms: Recursion
Genius test! 🤯 (10 seconds to solve!) #shorts #riddle #puzzle #games #braingames
Can you solve the 4 foods puzzle?
HOW TO SOLVE RUBIKS CUBE IN ONLY 2 MOVES
Algorithms: Bit Manipulation
How Neuralink Works 🧠
How Michael Reeves Learned To Code
This Hurt My Soul Ice Fishing
These GIANT Lawn Worms will give you nightmares. With @theunblockersaus
Ice Cream Sales Prediction with Linear Regression | Machine Learning Algorithm
11 years later ❤️ @shrads
Комментарии