filmov
tv
Design Patterns - Command Pattern Explanation and Implementation in C++
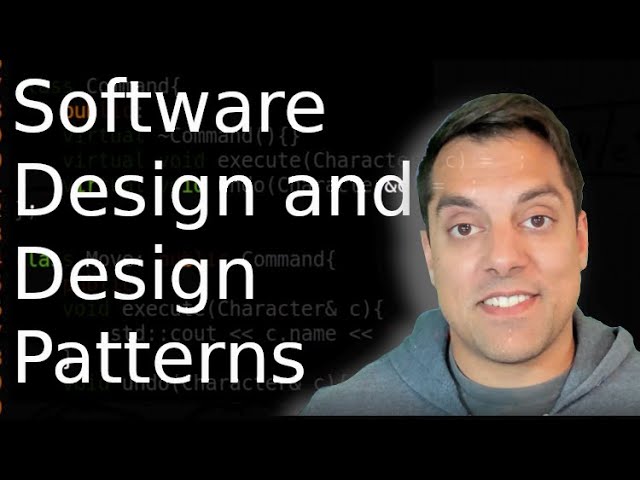
Показать описание
►Lesson Description: In this lesson I teach you one of the most powerful behavioral design patterns, the command pattern. This pattern models objects to act as a command, and it is one of the most convenient ways to store a series of 'commands' or actions that happen throughout your program. I will show you a use case of using the pattern to store commands as a queue, and this pattern could be used to elegantly implement and undo/redo system.
00:00 What are Design patterns
00:30 Behavioral Design Patterns
1:00 Command Pattern
1:30 Command Patterns uses
1:59 Design Pattern Resources
2:50 Conceptual Understanding of command pattern
4:00 A game controller example
4:40 Utilizing inheritance for is-a relationship
5:20 Key idea of what a command does
5:50 C++ explanation of virtual
6:30 Enqueing commands in some data structure
7:40 Undo commands in our history
9:10 Starting our command pattern in C++
10:15 Creating our command class
10:45 Creating our interface member functions
11:25 A simple use case for our command pattern
12:40 Creating a Move, which is a type of Command
13:50 Showing how pure virtual functions must be implemented
15:00 Creating a character
15:45 Passing our character as an argument
17:02 Preparing our character to move
18:25 Adding some output to see what is going on
20:00 Our characters first command
20:40 Storing multiple commands in a queue
23:00 Managing lifetime of our commands with pointers
24:40 Undoing our commands
25:30 How do we know it is working?
26:00 Storing position data for our character in move
29:35 Verifying our execute and undo work
31:30 Using a different data structure to perform undo
32:12 Final code walk through
34:10 Closing
►Please like and subscribe to help the channel!
Комментарии