filmov
tv
Correctly Remove a Node from a Linked List in C
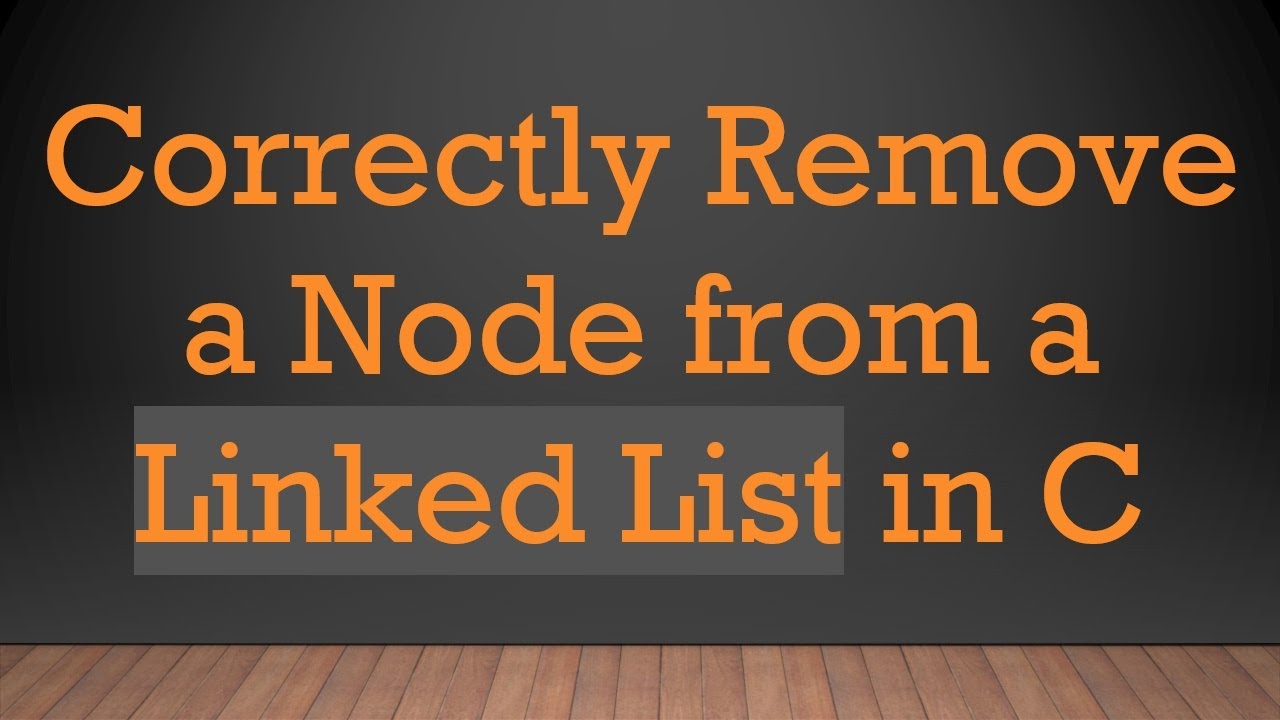
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Learn how to correctly remove a node from a singly linked list in C after performing a binary operation, with detailed steps and code examples.
---
Correctly Remove a Node from a Linked List in C
Working with linked lists is a fundamental aspect of programming in C, especially in lower-level data manipulation. One of the key operations you'll often need to perform is removing a node from a singly linked list. This guide will walk you through how to correctly remove a node after performing a binary operation in C, ensuring that your linked list remains well-structured and error-free.
Understanding the Basics
A singly linked list is a data structure consisting of nodes, where each node contains a value and a pointer to the next node. Here is a basic structure for a node in C:
[[See Video to Reveal this Text or Code Snippet]]
For this guide, let's assume you have already performed a binary operation and are now ready to remove a specific node from your list.
Steps to Correctly Remove a Node
Traverse the List
The first step is to traverse the linked list to find the node you want to delete. Traversal involves iterating through the list until you find the target node.
[[See Video to Reveal this Text or Code Snippet]]
Modify Pointers
When a node is found, adjust the next pointers of the surrounding nodes to bypass the node to be deleted.
Free Memory
It's crucial to free the memory allocated to the node to avoid memory leaks. Use the free() function to deallocate memory.
Example Function
Below is a complete example, incorporating all the steps:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
In summary, removing a node from a singly linked list in C requires careful traversal, pointer modification, and memory management. By correctly implementing these steps, you can ensure robust and efficient linked list operations.
---
Summary: Learn how to correctly remove a node from a singly linked list in C after performing a binary operation, with detailed steps and code examples.
---
Correctly Remove a Node from a Linked List in C
Working with linked lists is a fundamental aspect of programming in C, especially in lower-level data manipulation. One of the key operations you'll often need to perform is removing a node from a singly linked list. This guide will walk you through how to correctly remove a node after performing a binary operation in C, ensuring that your linked list remains well-structured and error-free.
Understanding the Basics
A singly linked list is a data structure consisting of nodes, where each node contains a value and a pointer to the next node. Here is a basic structure for a node in C:
[[See Video to Reveal this Text or Code Snippet]]
For this guide, let's assume you have already performed a binary operation and are now ready to remove a specific node from your list.
Steps to Correctly Remove a Node
Traverse the List
The first step is to traverse the linked list to find the node you want to delete. Traversal involves iterating through the list until you find the target node.
[[See Video to Reveal this Text or Code Snippet]]
Modify Pointers
When a node is found, adjust the next pointers of the surrounding nodes to bypass the node to be deleted.
Free Memory
It's crucial to free the memory allocated to the node to avoid memory leaks. Use the free() function to deallocate memory.
Example Function
Below is a complete example, incorporating all the steps:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
In summary, removing a node from a singly linked list in C requires careful traversal, pointer modification, and memory management. By correctly implementing these steps, you can ensure robust and efficient linked list operations.