filmov
tv
Advanced C++/Graphics Tutorial 15: ResourceManager, TextureCache!
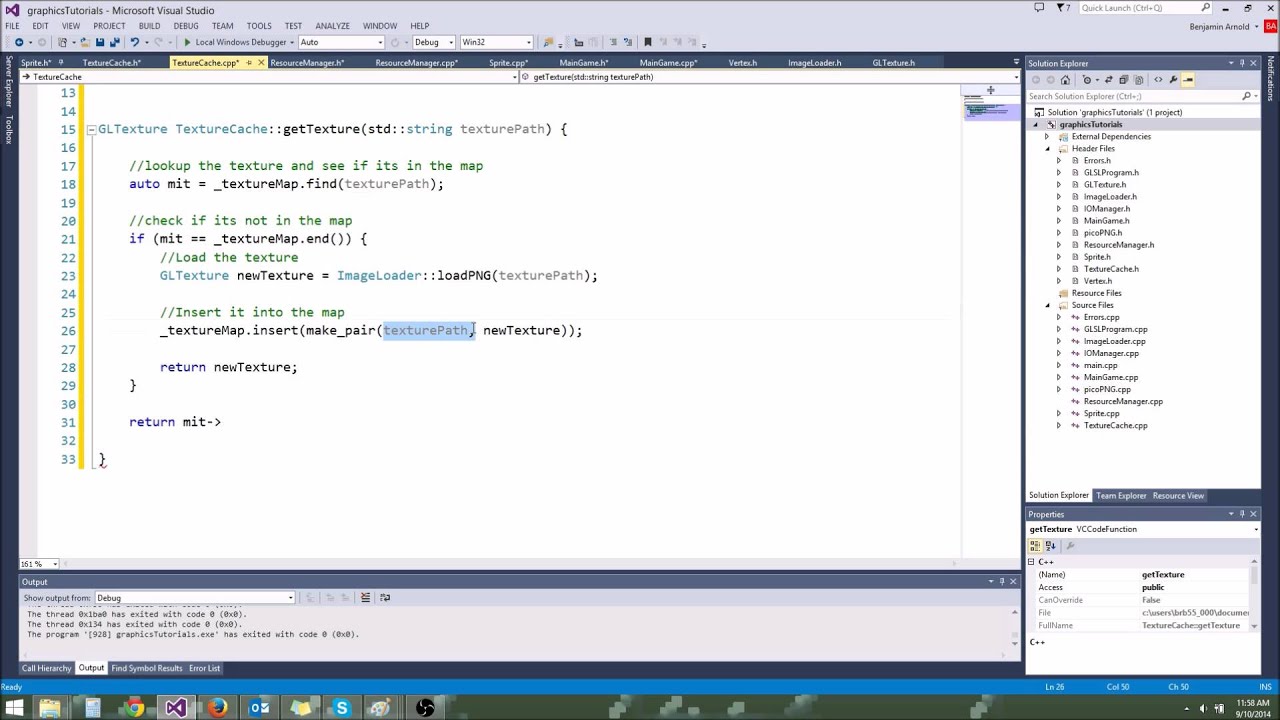
Показать описание
Now we get to implement texture caching! We have a lot more optimizations to learn so that our programs can run as fast as possible, but I want to do a challenge episode soon! So I may wait a bit before teaching you some of the optimizations.
Advanced C++/Graphics Tutorial 15: ResourceManager, TextureCache!
Advanced C++/Graphics Tutorial 30: Optimized Spritebatch!
Advanced C++/Graphics Tutorial 45: Finished IMainGame + IGameScreen
Advanced C++/Graphics Tutorial 16: FPS Counter and Limiter!
Advanced C++/Graphics Tutorial 42: Spatial Partition Pt. 2
Advanced C++/Graphics Tutorial 18: Custom Library, Bengine
Advanced C++/Graphics Tutorial 11: IOManager, more ifstream
Advanced C++/Graphics Tutorial 44: IMainGame + IGameScreen architecture
Advanced C++/Graphics Tutorial 35: for each loop
Advanced C++/Graphics Tutorial 12: lodePNG, GLTexture
Advanced C++/Graphics Tutorial 39: 2D Rotations!
Advanced C++/Graphics Tutorial 38: Function Pointers, Lambdas
Advanced C++/Graphics Tutorial 22 pt 2: Finished SpriteBatch, emplace_back
Resource Manager Demo
Advanced C++/Graphics Tutorial 19: Namespaces, Window
C++, SDL2 Game Engine # How I Builded my Texture Manager class
Advanced C++/Graphics Tutorial 58: LevelReaderWriter
Comment yes for more body language videos! #selfhelp #personaldevelopment #selfimprovement
Advanced C++/Graphics Tutorial 14: Maps, Binary Search, Caching!
Jamie- Programmer & Resource Manager
Advanced C++/Graphics Tutorial 56: CEGUI Event Handling review, new widgets.
Advanced C++/Graphics Tutorial 26: Vectors and Projectiles!
OpenGL/ C++ FPS Tutorial part 10: Project organization and basic ResourceManager class
Code Review: Resource Manager in C++ for Games
Комментарии