filmov
tv
Python API Development Course: Part 1
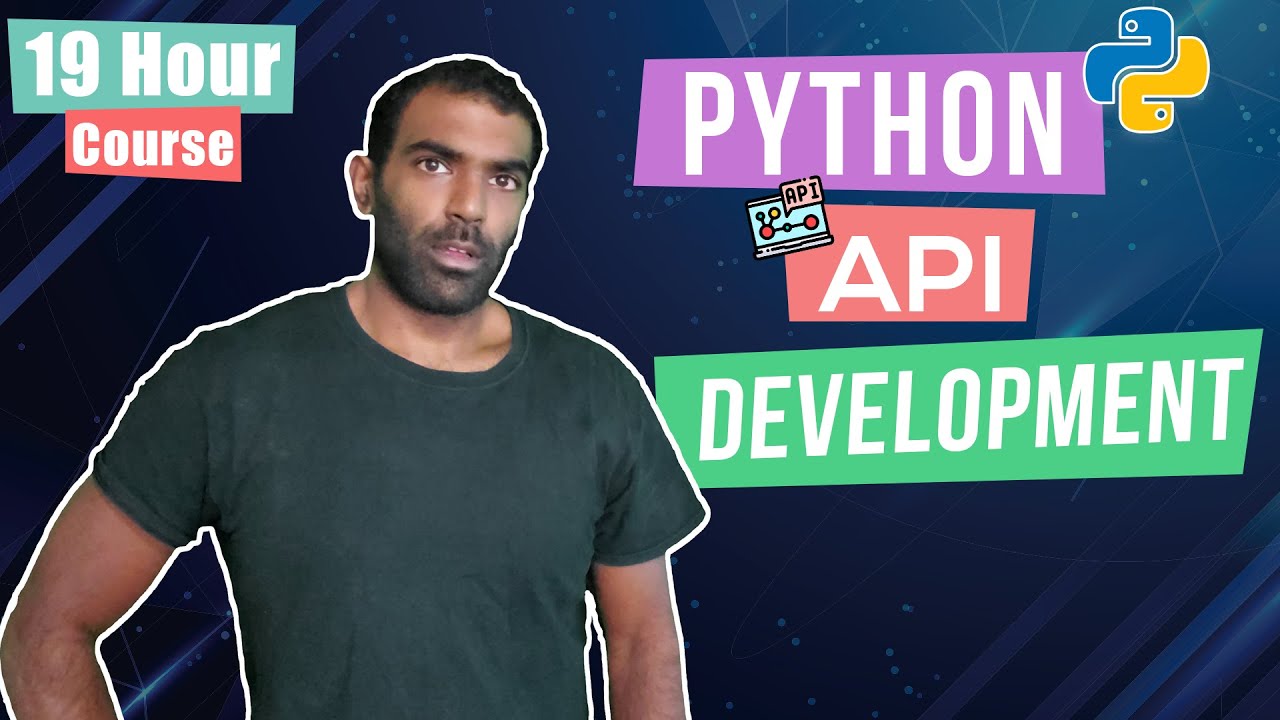
Показать описание
Part 2:
Playlist:
Github Repo:
Welcome to the greatest python API development course of all time(ehh probably not). In this course we are going to build a full fledge api in python. The api that we're building is for a social media type applications where, users can create/read/delete/update posts, as well as like other users posts and of course we will setup user registration and authentication. We will be building this api using the FastAPI library.
We're going to learn the fundamentals of API design including things like routes, serialization/deserialization, schema validation, models.
But the fun doesn't stop there, we're also going to do a deepdive into SQL databases. You do not need to have any prior experience with sql as we will be learning everything from scratch. I will show how to integrate our API with the database using both raw sql queries as well as with the SqlAlchemy ORM. I've chosen Postgres as our database, but everything we learn will be applicable to really any sql based database.
After that We'll move on to Testing our application using the pytest library. We'll setup a test database and perform a good number of integration tests.
I've including 2 deployment scenarios where we deploy on an ubuntu machine as well as to heroku and i've even included a section on how to dockerize our application.
Finally we'll build out a CI/CD pipeline using github actions so that we don't have to do everything manually.
This course is 19 hrs long but youtube has decided they want to get in the way of peoples education and put a hardcap on the length of uploadable videos, even though i've seen other people recently upload over 24hr videos. So i had to chop it up into 2 seperate videos. Here's the link to the playlist so that you can see Part 2 for some more python epicness.
If you like this video please subscribe to this channel. Don't let me go hungry! 🎁
▬▬▬▬▬▬ Support this garbage Channel 🍒 ▬▬▬▬▬▬
▬▬▬▬▬▬ Stalk me on Social Media 😲 ▬▬▬▬▬▬
Playlist:
Github Repo:
Welcome to the greatest python API development course of all time(ehh probably not). In this course we are going to build a full fledge api in python. The api that we're building is for a social media type applications where, users can create/read/delete/update posts, as well as like other users posts and of course we will setup user registration and authentication. We will be building this api using the FastAPI library.
We're going to learn the fundamentals of API design including things like routes, serialization/deserialization, schema validation, models.
But the fun doesn't stop there, we're also going to do a deepdive into SQL databases. You do not need to have any prior experience with sql as we will be learning everything from scratch. I will show how to integrate our API with the database using both raw sql queries as well as with the SqlAlchemy ORM. I've chosen Postgres as our database, but everything we learn will be applicable to really any sql based database.
After that We'll move on to Testing our application using the pytest library. We'll setup a test database and perform a good number of integration tests.
I've including 2 deployment scenarios where we deploy on an ubuntu machine as well as to heroku and i've even included a section on how to dockerize our application.
Finally we'll build out a CI/CD pipeline using github actions so that we don't have to do everything manually.
This course is 19 hrs long but youtube has decided they want to get in the way of peoples education and put a hardcap on the length of uploadable videos, even though i've seen other people recently upload over 24hr videos. So i had to chop it up into 2 seperate videos. Here's the link to the playlist so that you can see Part 2 for some more python epicness.
If you like this video please subscribe to this channel. Don't let me go hungry! 🎁
▬▬▬▬▬▬ Support this garbage Channel 🍒 ▬▬▬▬▬▬
▬▬▬▬▬▬ Stalk me on Social Media 😲 ▬▬▬▬▬▬
Комментарии