filmov
tv
Understanding C++ Vector (Dynamic Arrays)
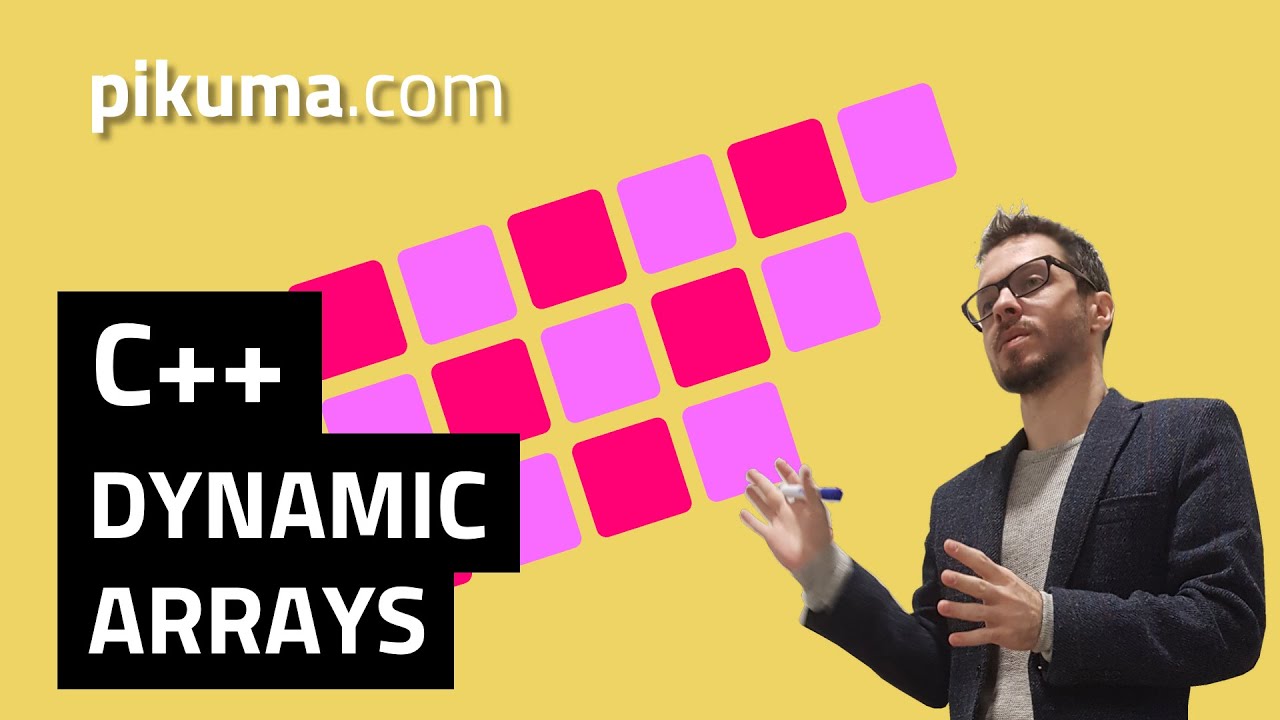
Показать описание
In this video, we'll take a closer look at C++ vectors (STL implementation of a dynamic array).
We'll learn how C++ vectors are implemented, and code a very simple custom class with the absolute minimum needed from a dynamic array data structure.
std::vector is a sequence container that encapsulates dynamic size arrays in C++. The elements are stored contiguously, which means that elements can be accessed not only through iterators but also using offsets to regular pointers to elements.
The storage of the vector is handled automatically, being expanded as needed. Vectors usually occupy more space than static arrays, because more memory is allocated to handle future growth. This way a vector does not need to reallocate each time an element is inserted, but only when the additional memory is exhausted.
Chapters:
00:00:00 Introduction to C++ vector
00:01:40 C++ static arrays
00:08:11 Using the STL vector class
00:15:02 A short discussion on abstraction
00:16:47 Creating our own 'toy' Vector class
00:19:10 Vector methods and algorithm complexity
00:24:11 Vector using generics with C++ templates
00:28:28 Private members of our Vector class
00:32:33 Vector function prototypes and signatures
00:43:10 Constructors and destructors
00:48:13 Square-brackets operator overloading
00:48:59 Getter methods
00:49:54 Copy constructor
00:51:43 PushBack method
01:00:04 PopBack method
01:02:35 Clear method
01:03:06 Erase method
01:06:01 Assignment operator overloading
01:08:49 Insert method (proposed exercise)
01:10:05 Conclusion & next steps
For comprehensive courses on computer science, retro programming, and mathematics, visit:
Don't forget to subscribe to receive updates and news about new courses and tutorials:
Enjoy!
We'll learn how C++ vectors are implemented, and code a very simple custom class with the absolute minimum needed from a dynamic array data structure.
std::vector is a sequence container that encapsulates dynamic size arrays in C++. The elements are stored contiguously, which means that elements can be accessed not only through iterators but also using offsets to regular pointers to elements.
The storage of the vector is handled automatically, being expanded as needed. Vectors usually occupy more space than static arrays, because more memory is allocated to handle future growth. This way a vector does not need to reallocate each time an element is inserted, but only when the additional memory is exhausted.
Chapters:
00:00:00 Introduction to C++ vector
00:01:40 C++ static arrays
00:08:11 Using the STL vector class
00:15:02 A short discussion on abstraction
00:16:47 Creating our own 'toy' Vector class
00:19:10 Vector methods and algorithm complexity
00:24:11 Vector using generics with C++ templates
00:28:28 Private members of our Vector class
00:32:33 Vector function prototypes and signatures
00:43:10 Constructors and destructors
00:48:13 Square-brackets operator overloading
00:48:59 Getter methods
00:49:54 Copy constructor
00:51:43 PushBack method
01:00:04 PopBack method
01:02:35 Clear method
01:03:06 Erase method
01:06:01 Assignment operator overloading
01:08:49 Insert method (proposed exercise)
01:10:05 Conclusion & next steps
For comprehensive courses on computer science, retro programming, and mathematics, visit:
Don't forget to subscribe to receive updates and news about new courses and tutorials:
Enjoy!
Комментарии