filmov
tv
Dynamic Arrays 🌱
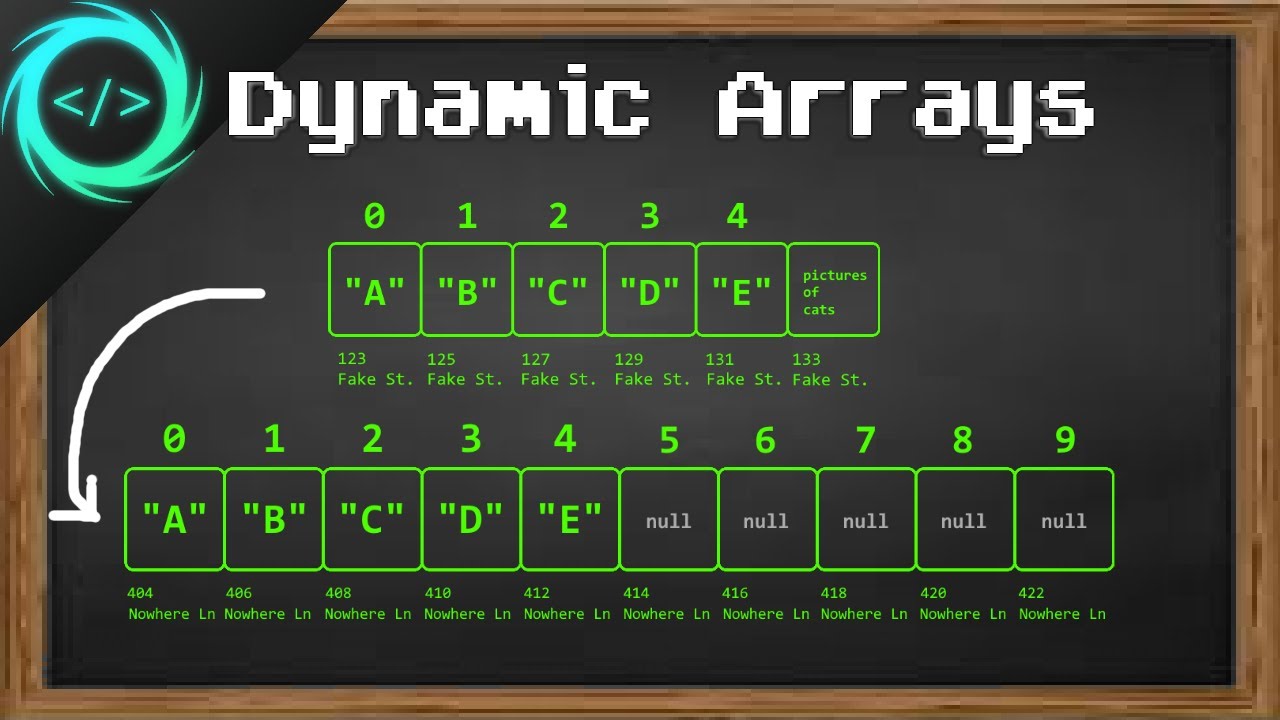
Показать описание
Dynamic arrays arraylists tutorial example explained
Part 1 (00:00:00) theory
Part 2 (00:05:00) practice
#dynamic #arrays #arraylists
Part 1 (00:00:00) theory
Part 2 (00:05:00) practice
#dynamic #arrays #arraylists
Excel Dynamic Arrays (How they will change EVERYTHING!)
Dynamic Arrays 🌱
Design a Dynamic Array (Resizable Array)
C++ POINTERS (2020) - How to create/change arrays at runtime? (Dynamic arrays) PROGRAMMING TUTORIAL
Using dynamic arrays in a Table : 4 methods | Excel Off The Grid
The Simple and Elegant Idea behind Efficient Dynamic Arrays
What if you had to invent a dynamic array?
2. Data Structures and Dynamic Arrays
Introduction to Dynamic arrays part - 1 || System verilog complete course ||
Why Dynamic Arrays Are Moving in Memory?
Dynamic Arrays in C
Referencing Dynamic Arrays with Tables
Dynamic Arrays in C++ (std::vector)
C++ Programming Tutorials - 30 - C++ Dynamic Arrays - Eric Liang
C++ Dynamic Arrays (Dynamically Allocated Array)
What is a Dynamic Array Formula in Excel?
Dynamic Arrays of Objects (Data Structures course, step-by-step)
Excel Dynamic Arrays and How to use them...
Basics of Dynamic Memory Allocation
Two Excel Dynamic Array Functions: UNIQUE and SORT
Static Arrays vs. Dynamic Arrays
How to use Dynamic Arrays in Excel and other Advanced Formulas
#️⃣ Dynamic Arrays in Excel - This Changes Everything!
How to use dynamically allocated arrays
Комментарии