filmov
tv
Memorize this Array Time Complexity Cheat Sheet!
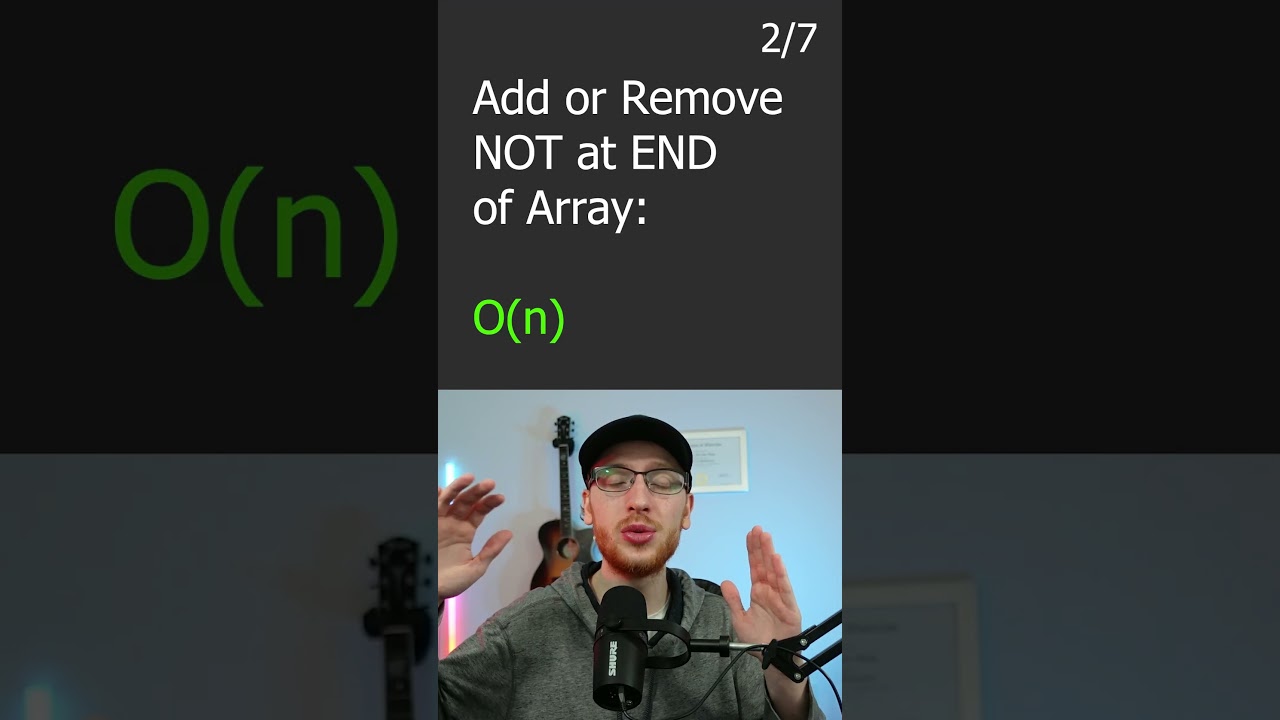
Показать описание
dynamic programming, leetcode, coding interview question, data structures, data structures and algorithms, faang
Memorize this Array Time Complexity Cheat Sheet!
Array Data Structure Tutorial - Array Time Complexity
Calculating Time Complexity | Data Structures and Algorithms| GeeksforGeeks
LeetCode is a JOKE with This ONE WEIRD TRICK
Fastest way to learn Data Structures and Algorithms
LeetCode was HARD until I Learned these 15 Patterns
8 patterns to solve 80% Leetcode problems
3 Tips I’ve learned after 2000 hours of Leetcode
DSA In Java || Sorting Algorithms in Java || Coders Arcade
Quick Guide: Time Complexity Analysis in Under 1 Minute!
Time & Space Complexity Cheatsheet for Sorting Algorithms
How to Start Leetcode (as a beginner)
Big-O Notation - For Coding Interviews
Sorting Algorithms Explained Visually
L-1.6: Time Complexities of all Searching and Sorting Algorithms in 10 minute | GATE & other Exa...
Learn Big O notation in 6 minutes 📈
I gave 127 interviews. Top 5 Algorithms they asked me.
Students in first year.. 😂 | #shorts #jennyslectures #jayantikhatrilamba
Struggle with Time Complexity? Watch this.
How I Remember ALGORITHMS | PLACEMENT PREPARATION
Memoization: The TRUE Way To Optimize Your Code In Python
My Brain after 569 Leetcode Problems
Time Complexity for Coding Interviews | Big O Notation Explained | Data Structures & Algorithms
1.11 Best Worst and Average Case Analysis
Комментарии