filmov
tv
Memoization: The TRUE Way To Optimize Your Code In Python
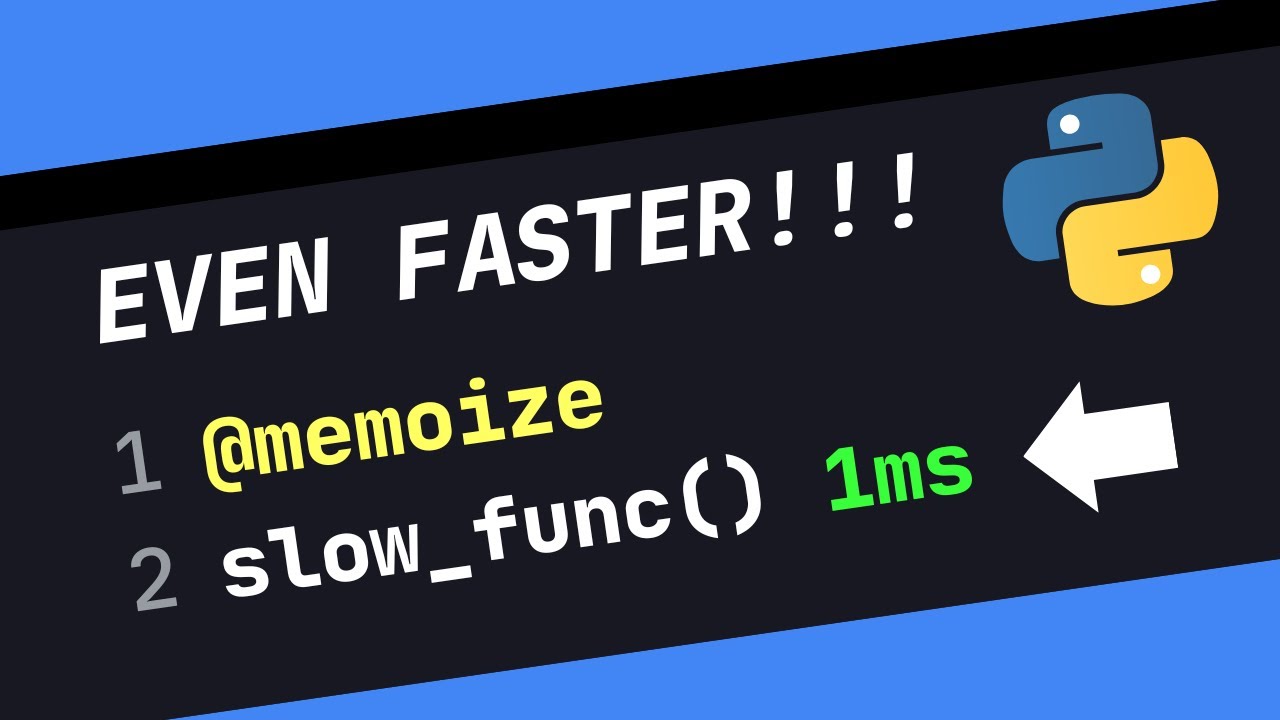
Показать описание
Learn how you can optimize your code using memoization, a form of caching computations that have already been made in recursive functions. Incredibly useful and can really optimize slow functions.
▶ Become job-ready with Python:
▶ Follow me on Instagram:
▶ Become job-ready with Python:
▶ Follow me on Instagram:
Memoization: The TRUE Way To Optimize Your Code In Python
Algorithms: Memoization and Dynamic Programming
Memoization in Python in 5 Minutes
Implementing Memoization for Recursive Fibonacci in Python // #Shorts
Using memoization in Python
26 - Memoization in Python: Speed Up Your Code with Caching - #shorts #python #programming
Python lru_cache stores the function result and saves time on repetitive execution #Shorts
What is Memoization?
Memo Tables and the Magic of Higher Order Functions in Python!
Dynamic Programming - Top Down Memoization & Bottom Up Tabulation - DSA Course in Python Lecture...
memoization
maximize efficiency with #memoization #python #coding #programming #shorts
Unique Paths 2 LeetCode Question | Top Down Memoization Solution
4 Principle of Optimality - Dynamic Programming introduction
Dynamic Programming - Learn to Solve Algorithmic Problems & Coding Challenges
5 Simple Steps for Solving Dynamic Programming Problems
React memoization explained in the right way | UseMemo and React.memo Everything You Need to Know
Boost JavaScript Performance with Memoization: Real-Life Job Interview Success Story!
Scientific Python : 019 : Memoization in detail
True Wisdom comes from understanding, not memorization.” - Naval
How to Memorize Fast and Easily
The Correct Way to Measure time of code Execution in Python - You were doing it wrong all along!
State vs. Memoization: Finding the Right Balance with Hooks w/Sumit Ridhal | HackBuddy
10 Mind Tricks to Learn Anything Fast!
Комментарии