filmov
tv
Arbitrary Rectangle Collision Detection & Resolution - Complete!
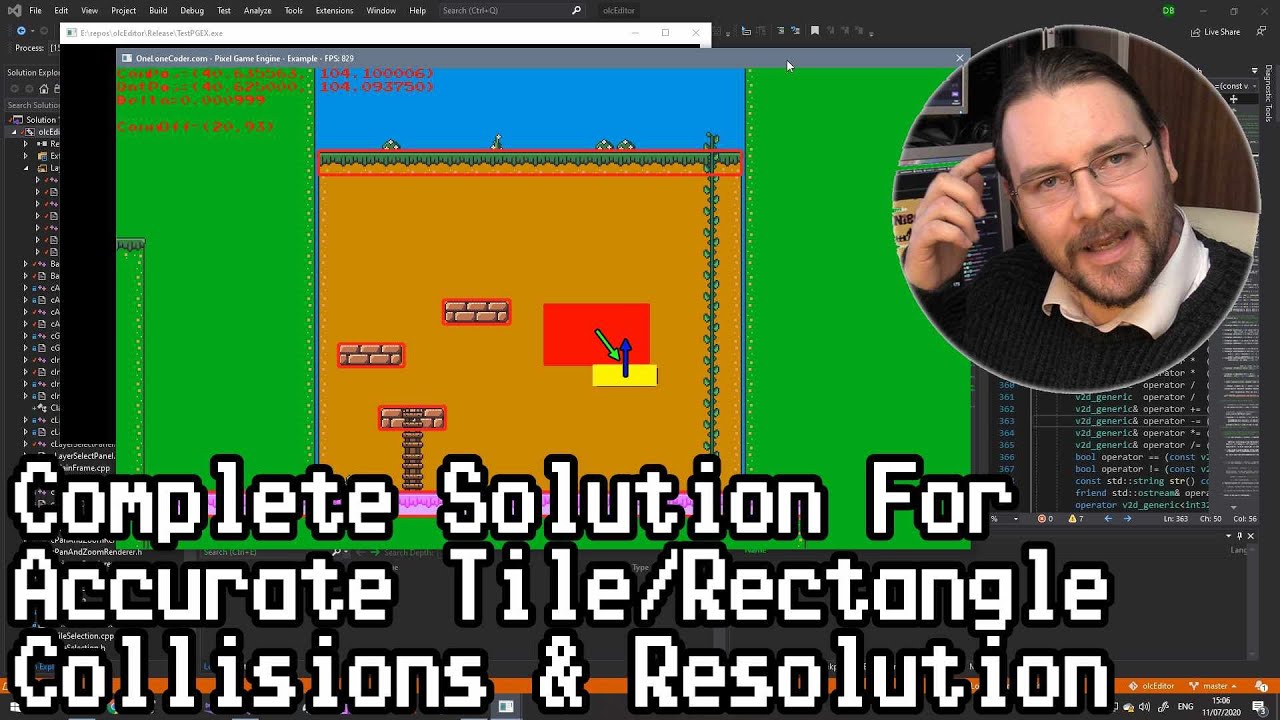
Показать описание
In this video I once and for all solve axis aligned rectangle collision detection, demonstrating algorithms to handle arbitrary size rectangle vs rectangle collisions and collision resolution, applicable to "rectangle soups" or tile map based interactions.
Arbitrary Rectangle Collision Detection & Resolution - Complete!
Collision Detection Guide | Rectangle - Rectangle
How to Code: Rectangular Collision Detection with JavaScript
Collision Detection Between Rectangles in JavaScript
Collision Detection Guide | Circle - Rectangle
Collision Detection (An Overview) (UPDATED!)
Rectangle Collision Demo
Learn Rectangular Collision Detection in Javascript!
How 2D Game Collision Works (Separating Axis Theorem)
Detect if a point is inside a rectangle (pong collision detection)
Arbitrary Geometry Collision Detection
kha tutorial series - episode 048 - collision rectangle
Circle Vs Rectangle Collisions (and TransformedView PGEX)
14. C++ AABB Collision and Movement - Celeste Clone
[Game Algorithms] 01 - Rectangle Collision Normal
2d Rectangle collision detection for JavaScript game development
Collision Detection Between Rotated Rectangles – HTML5 Canvas
COMP4300 - Game Programming - Lecture 08 - Collision Detection
Collisions in Pygame - Beginner Tutorial
Ladder test (Rectangle Collision detection test)
AABB vs AABB Collision Detection | C Game + Engine From Scratch 07
Swept AABB Collision Detection | C Game + Engine From Scratch 08
BSP Trees: The Magic Behind Collision Detection in Quake
Programming Rectangle Intersection/Collision in Processing
Комментарии