filmov
tv
Decoding Base64 Strings to Bytes in Python
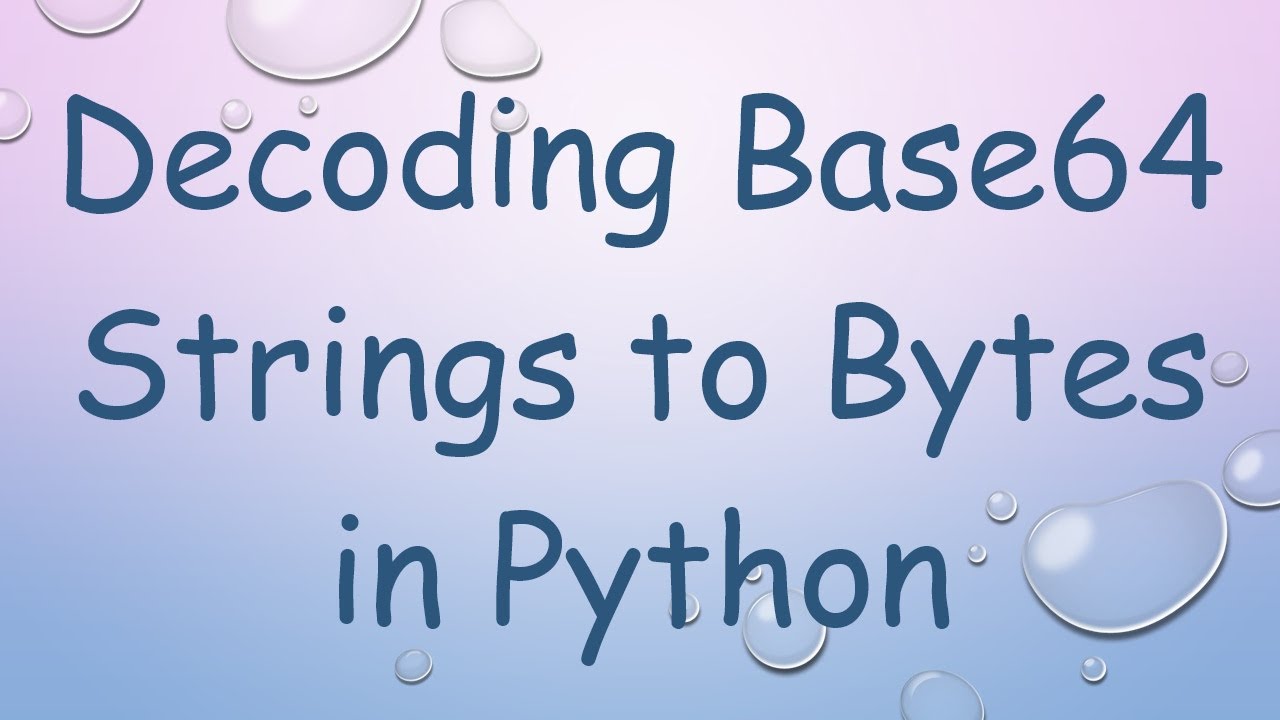
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Learn how to decode Base64 strings to bytes in Python using the base64 module. Understand the process and explore examples for efficient conversion.
---
Base64 encoding is a widely-used technique for converting binary data into a text format, which is ASCII-based and safe for transmission over text protocols, such as email or HTML. In Python, the base64 module provides functions to encode and decode Base64 data. This guide will guide you through the process of decoding Base64 strings into bytes using Python.
Understanding Base64 Encoding
Base64 encoding represents binary data in an ASCII string format by converting it into a set of six-bit characters. This encoding is commonly used for encoding binary data, such as images or files, into a format suitable for text-based protocols.
Decoding Base64 Strings to Bytes
In Python, decoding a Base64 string to bytes involves using the base64 module's b64decode function. Here's a simple example:
[[See Video to Reveal this Text or Code Snippet]]
In this example, the base64_string variable contains the Base64 encoded data, and base64.b64decode() is used to decode it into bytes. The result is printed, revealing the original binary data.
Handling Padding and Errors
It's important to note that Base64 strings must have a length divisible by 4. If the length is not divisible by 4, padding characters ('=') are added to the end of the string. The b64decode function handles this padding automatically.
[[See Video to Reveal this Text or Code Snippet]]
In this example, the string has padding characters, and the b64decode function handles it seamlessly.
Conclusion
Decoding Base64 strings to bytes in Python is a straightforward process using the base64 module. Whether you're working with encoded images, files, or other binary data, understanding how to decode Base64 strings is a valuable skill.
Now that you've learned the basics, you can confidently handle Base64 decoding in your Python projects. If you have any questions or want to explore more advanced use cases, refer to the official Python documentation for the base64 module.
---
Summary: Learn how to decode Base64 strings to bytes in Python using the base64 module. Understand the process and explore examples for efficient conversion.
---
Base64 encoding is a widely-used technique for converting binary data into a text format, which is ASCII-based and safe for transmission over text protocols, such as email or HTML. In Python, the base64 module provides functions to encode and decode Base64 data. This guide will guide you through the process of decoding Base64 strings into bytes using Python.
Understanding Base64 Encoding
Base64 encoding represents binary data in an ASCII string format by converting it into a set of six-bit characters. This encoding is commonly used for encoding binary data, such as images or files, into a format suitable for text-based protocols.
Decoding Base64 Strings to Bytes
In Python, decoding a Base64 string to bytes involves using the base64 module's b64decode function. Here's a simple example:
[[See Video to Reveal this Text or Code Snippet]]
In this example, the base64_string variable contains the Base64 encoded data, and base64.b64decode() is used to decode it into bytes. The result is printed, revealing the original binary data.
Handling Padding and Errors
It's important to note that Base64 strings must have a length divisible by 4. If the length is not divisible by 4, padding characters ('=') are added to the end of the string. The b64decode function handles this padding automatically.
[[See Video to Reveal this Text or Code Snippet]]
In this example, the string has padding characters, and the b64decode function handles it seamlessly.
Conclusion
Decoding Base64 strings to bytes in Python is a straightforward process using the base64 module. Whether you're working with encoded images, files, or other binary data, understanding how to decode Base64 strings is a valuable skill.
Now that you've learned the basics, you can confidently handle Base64 decoding in your Python projects. If you have any questions or want to explore more advanced use cases, refer to the official Python documentation for the base64 module.