filmov
tv
Number of 1 Bits - Leetcode 191 - Python
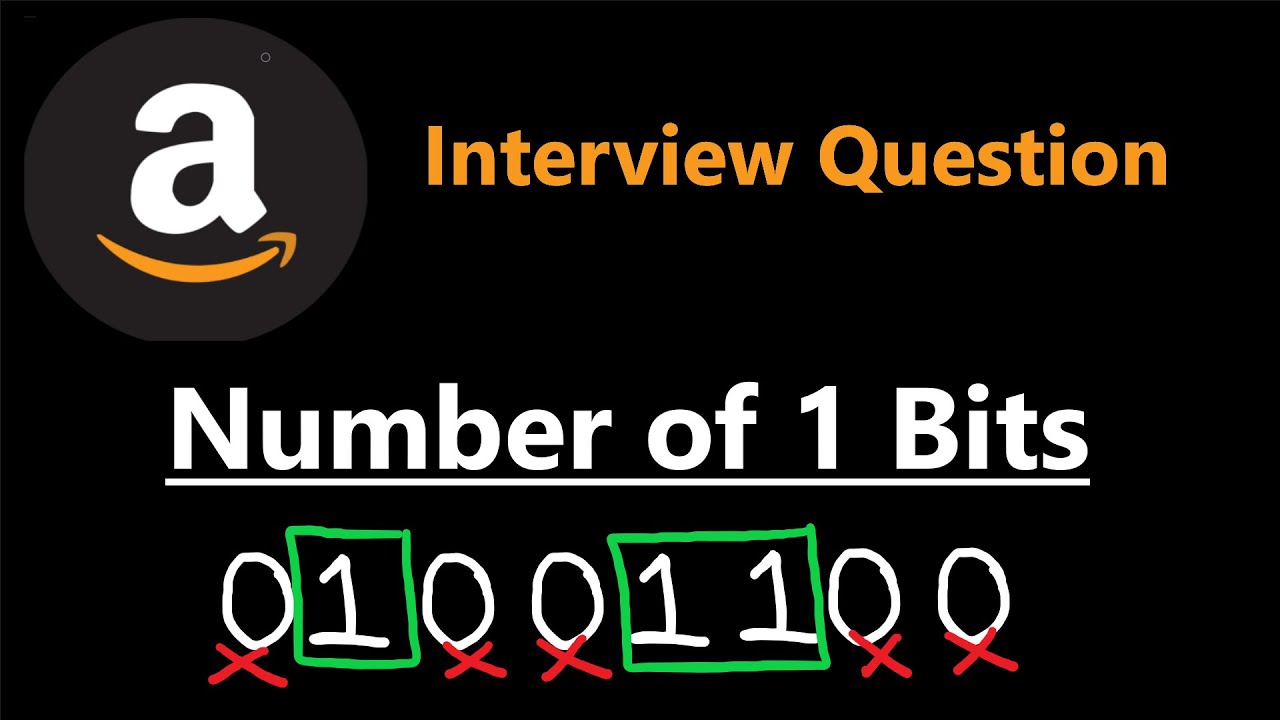
Показать описание
0:00 - Read the problem
1:10 - Explain Solution #1
4:33 - Coding Solution #1
5:40 - Explain Solution #2
11:03 - Coding Solution #2
leetcode 191
#amazon #interview #python
Disclosure: Some of the links above may be affiliate links, from which I may earn a small commission.
Number of 1 Bits - Leetcode 191 - Python
Number of 1 Bits - Leetcode 191 - Bit Manipulation (Python)
Bit Manipulation Series Part 4 | Number of 1 Bits & Trailing Zeroes
Number of 1 Bits | Leetcode | Python
Number of 1 Bits | Live Coding with Explanation | Leetcode - 191
Number of 1 Bits | 4 Approaches | Time Complexity | Apple | Microsoft | Amazon | Leetcode 191
Number of 1 Bits | Live Coding with Explanation | Leetcode - 191
✅ Number of 1 Bits - Simple Interview Solution - LeetCode 191 - Visualized Too
Raspberry Pi Pico W LESSON 88: Understanding PIO State Machine Push, Pull, Put and Get
191. Number of 1 Bits | 2 Ways | O(No of Set Bits)
Counting Bits - Dynamic Programming - Leetcode 338 - Python
Number of 1 Bits : LeetCode Solution Explained with JavaScript
Bit Manipulation || Number of 1 Bits || Leetcode 191 || C++ || Java
Bit Wars - 64 bits, 32 bits, 16 bits, 8 bits, 4 bits, 2 bits, 1 bit
E001 : Number of 1 bits | Bit Manipulation | CodeNCode
Counting Bits | Leetcode #338
Number of 1 Bits - Leetcode 191 - Java | 3 Easy Methods
Number of 1 Bits Problem | Detailed Explanation and Code (Java ) | LeetCode 191
191. Number of 1 Bits
6.0 NUMBER OF 1 BITS(Bit Manipulation)-Interviewbit #bitmanipulaiton#programming
Lec 6.10: Number of 1 Bits | Leetcode Problem | Bit Manipulation | Data Structure and Algorithm
64 bits 128 bits 256 bits 512 bits 1024 bits 2048 bits 4096 bits 8192 16384 bits Greg Heffley
1 bit 2 bits 4 bits 8 bits 16 bits 32 bits 64 bits 128 bits 256 bits 512 bits 1024 bits - TrollFace
LC: 1356 | Sort Integers by the number of 1 Bits
Комментарии