filmov
tv
Counting Bits - Dynamic Programming - Leetcode 338 - Python
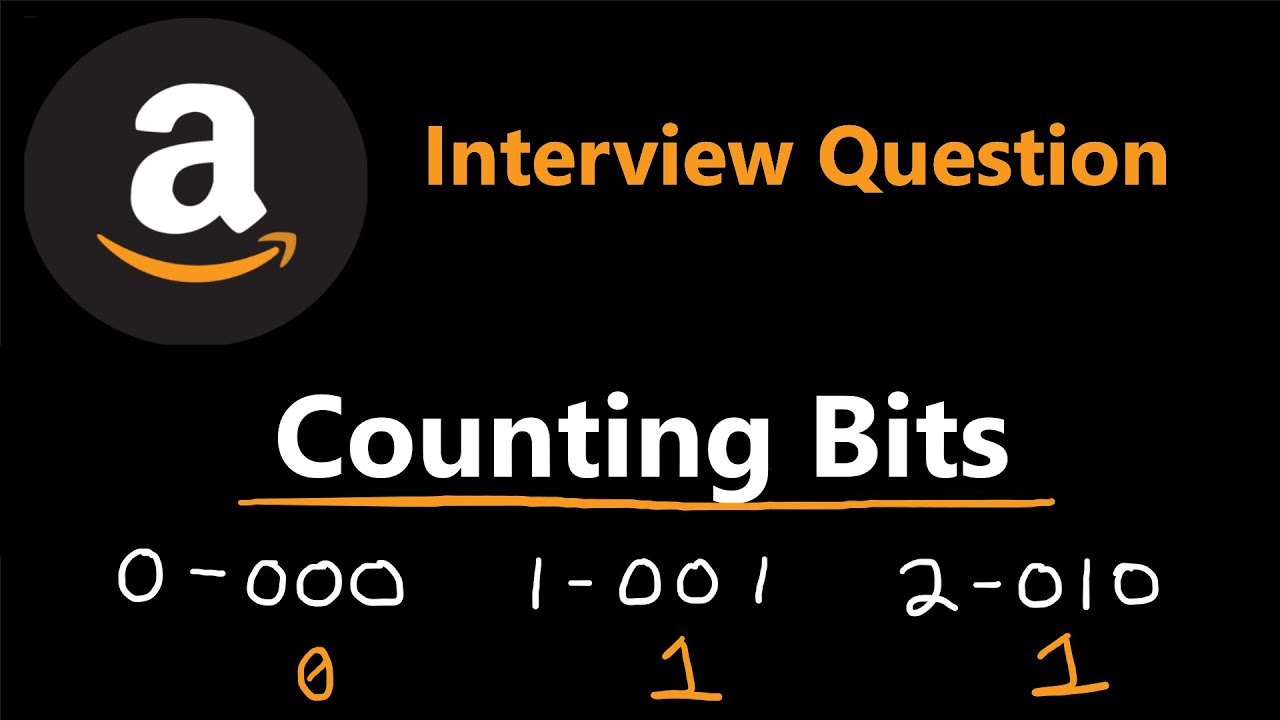
Показать описание
0:00 - Read the problem
4:28 - Drawing Explanation
11:38 - Coding Explanation
leetcode 338
#counting #bits #python
Disclosure: Some of the links above may be affiliate links, from which I may earn a small commission.
Counting Bits - Dynamic Programming - Leetcode 338 - Python
(Remade) Leetcode 338 - Dynamic Programming | Counting Bits
Counting Bits | Leetcode #338
Leetcode Counting Bits | Dynamic Programming | Python
61 - Counting Bits - Dynamic Programming approach 1
Leetcode 338. Counting Bits, Dynamic Programming
(Old) Leetcode 338 - Dynamic Programming | Counting Bits
Leetcode 338. Counting Bits | Динамическое программирование
Solving Counting Bits Problem Using Dynamic Programming
[Animated] LeetCode 338 Counting Bits | Blind 75 | Dynamic Programming
Counting Bits - LeetCode 338 - Python - Dynamic Programming! (Bit Shift Solution)
Counting Bits | Dynamic Programming | Leetcode#338
E007 : Counting Bits using DP | Bit Manipulation | CodeNCode
Bit manipulation - dynamic programming interview question || Counting Bits -: Blind 75: Leetcode 338
338. Counting Bits | LEETCODE EASY | DYNAMIC PROGRAMMING
Leetcode - Counting Bits (Python)
LeetCode in Golang - Counting Bits
Counting Bits | Leetcode | Algorithm explanation by alGOds
Uncover Binary Secrets with Dynamic Programming! | Counting Bits - LeetCode 338 | Live Coding
LeetCode 338: Counting Bits | Python Inbuilt Function v/s Dynamic Programming | Hack Code
LeetCode 338. Counting Bits (Dynamic Programming)
Counting Bits | Leetcode 338 | Dynamic Programming | Bit manipulation | Day-1
Counting Bits - Dynamic Programming - Amazon Interview Question - Python - LeetCode 338
338. Counting Bits - Day 1/31 Leetcode March Challenge
Комментарии