filmov
tv
How to Deal with Multiple Return Values in C++
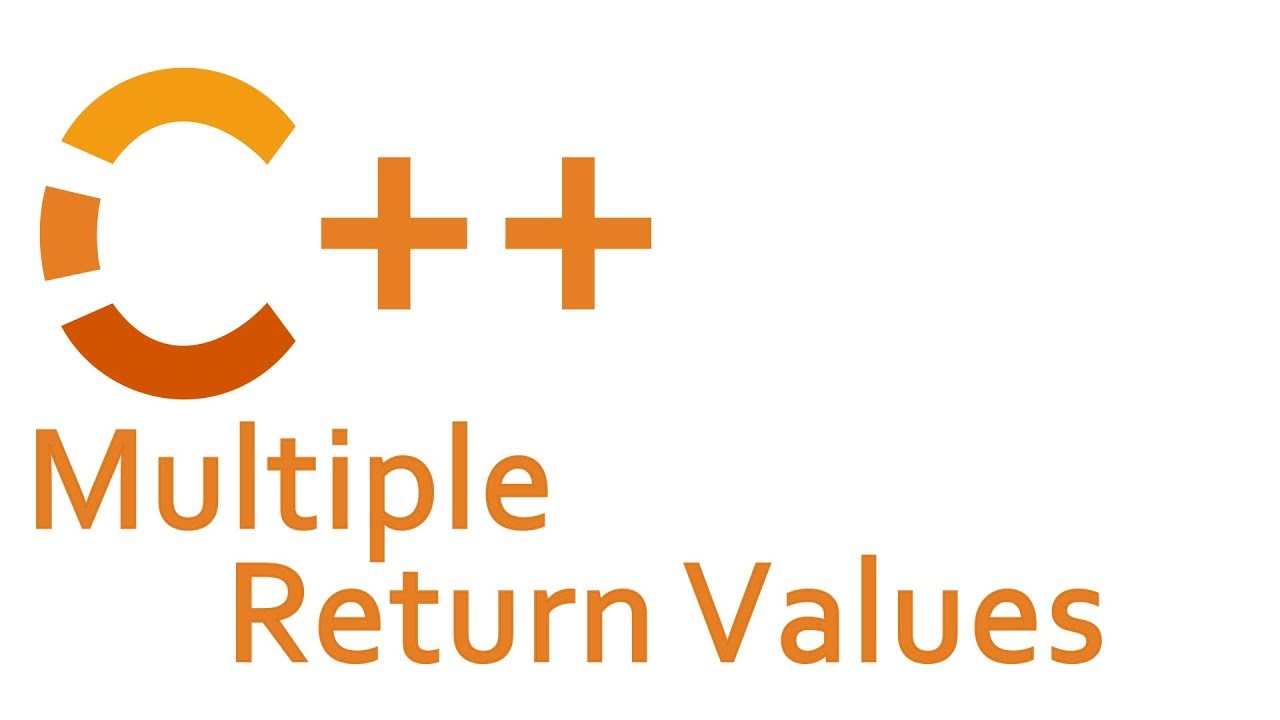
Показать описание
Thank you to the following Patreon supporters:
- Samuel Egger
- Dominic Pace
- Kevin Gregory Agwaze
- Sébastien Bervoets
- Tobias Humig
- Peter Siegmund
Gear I use:
-----------------
How To Deal With Multiple Losses | Simple & Effective Advice
How to Deal with Multiple Return Values in C++
How to Deal with Multiple Focal Points in a Room
How To Deal With Multiple Problems At Once - Jocko Willink
How To Deal With Threatening People in Public - Jocko Willink
How to deal with rejection
DAX Fridays! #183: How to deal with multiple dates in Power BI
How To Deal With Client Revisions
How Do I Deal With Multiple Years Of Unfiled Income Tax Returns And Messy Records?!
How To Deal With Enemies In Life and Toxic Culture
How to Deal with Multiple Header rows in Power Query | PowerBI | Excel | MiTutorials
☯️ How To Deal with Multiple Queen Cells
How to deal with two-faced people
What to do when staff or coworkers undermine you? How to deal with a difficult employee.
How to develop skill | Deal with Multiple Attackers, Aggression and Violence #combatives #kravmaga
How-to move a deal from one pipeline to another in HubSpot.
How to Deal With Concurrency in Python
How to Deal with Relationships? | Sadhguru
COVID-19 and Multimorbidity: How to Deal with Multiple Infectious Diseases in Parallel?
The Four Personality Types and How to Deal with Them
How to Deal With Loving Two Girls at the Same Time
how to deal with friend breakups | detachment, friendship standards, moving on
Algebra - Ch. 0.6: Basic Concepts (9 of 36) How to Deal with Multiple Negative Signs
Multiple static methods inside a single Class | How to deal it?
Комментарии