filmov
tv
This is why you need TypeScript type guards in Angular
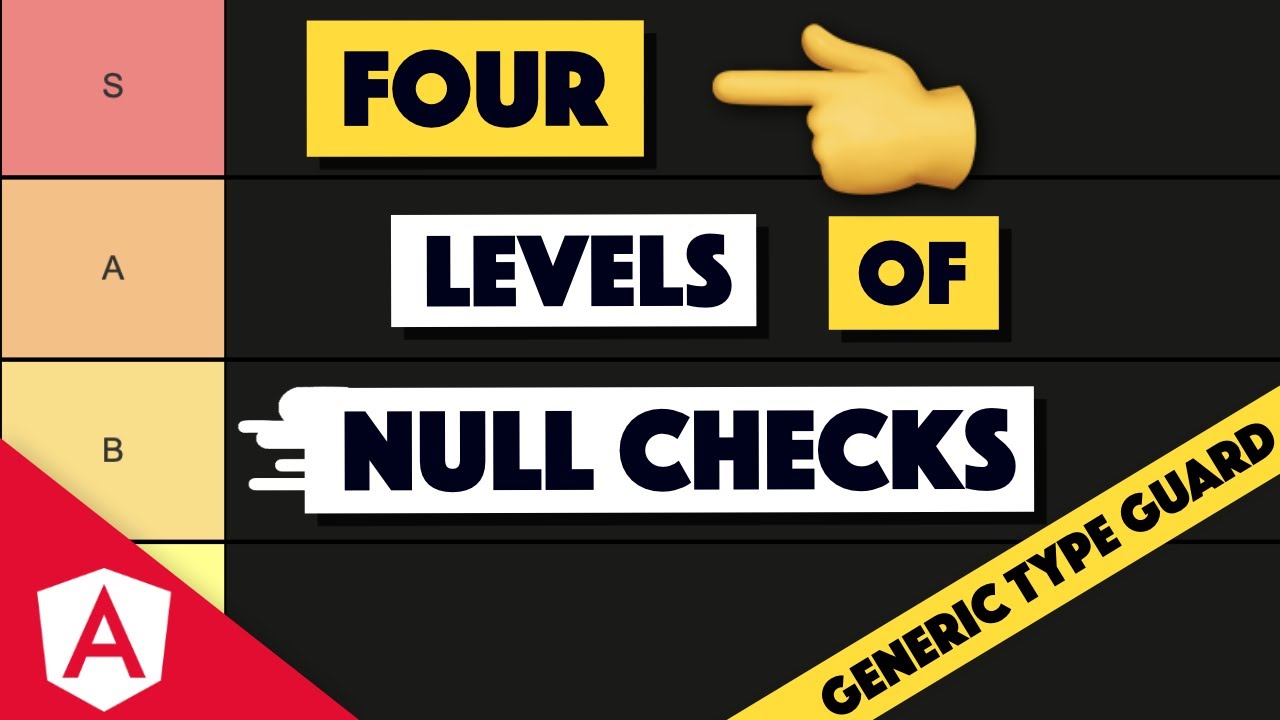
Показать описание
We take a look at four different approaches to dealing with potentially null values in Angular, ending in creating a generic type guard that we can use to assign a type to any object on the condition that it has no null values.
0:00 Introduction
0:15 The problem
1:01 Type assertion
1:45 Manual check
2:31 Type guard
4:23 Generic type guard
#ionic #angular
Jesse Ruben - This Is Why I Need You
What U Need - Sonic Rush [OST]
Ashley Sienna - What You Need (Official Video)
The Weeknd - What You Need (Lyrics)
Ashley Sienna - What You Need (Lyrics)
6 things you probably need to hear
The Weeknd - What You Need
This is why you need a COMPRESSOR
Pray This Psalm When You Need God To Lift You Up | A Blessed Morning Prayer To Start Your Day
What U Need is Remix - Sonic Rush [OST]
This is Why You Need a Property Survey When Buying Real Estate
We Need to Rethink Exercise (Updated Version)
BAYNK - What You Need (ft. NÏKA)
What U Need (Blazy Mix) - Sonic Rush [OST]
LOVE IS ALL YOU NEED - Trailer (Full-HD) - Deutsch / German
Gaming Websites You Need To Know
LeAnn Rimes - I Need You (Lyrics) 'I need you like water like breath like rain'
Why YOU NEED The New Mac Mini (M4)
[2020] Sonic Rush - What U Need [SD's Final Mix]
Attention Is All You Need
15 habits you NEED in 2024 ✧ exit lazy girl era ✧・゚: *✧・゚:*
AMORPHIS - You I Need (Official Music Video)
New rules of travel you need to know
There are four people that you need to forgive #motivation #denzelwashington #inspirationalquotes
Комментарии