filmov
tv
[Python Programming Basics to Advanced]: Local and Global Variables (LEGB Rule) | Lab 18P-2
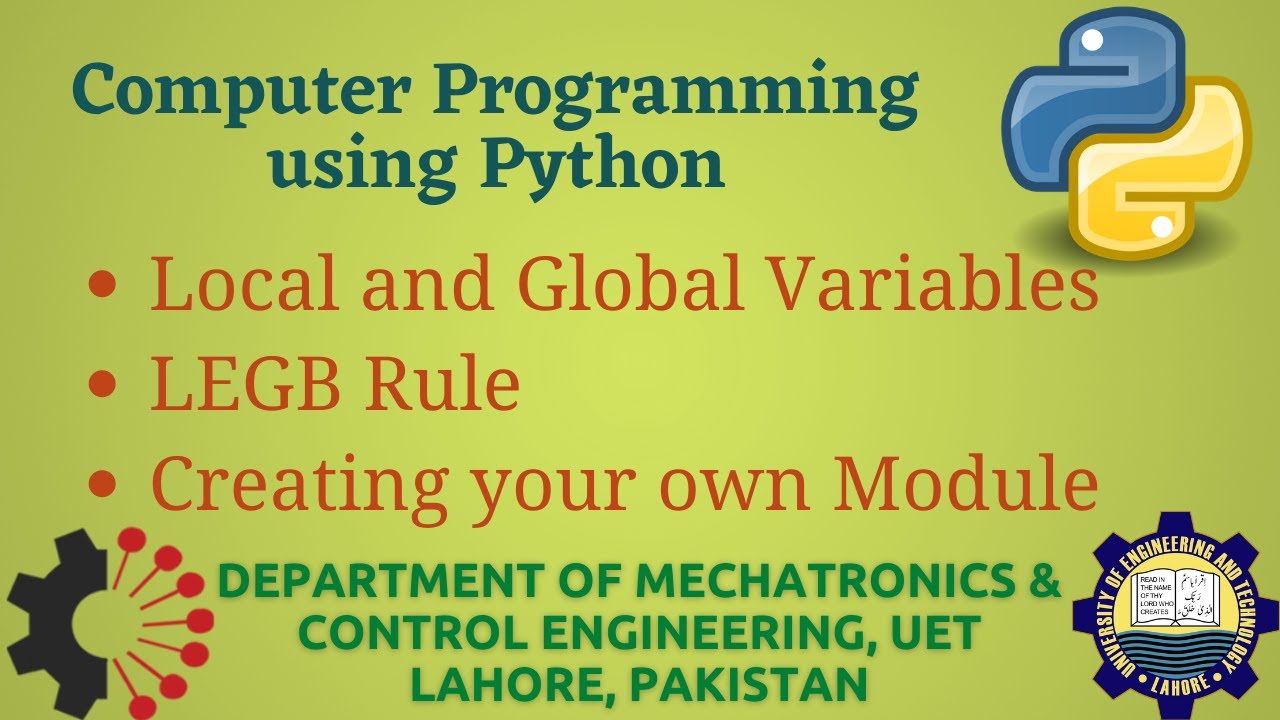
Показать описание
This Python programming playlist is designed to take beginners with zero programming experience to an expert level. The course covers installation, basic syntax, practical scenarios, and efficient logic building. The course material includes PDF handouts, review questions, and covers a wide range of topics, from data types to advanced functions like Lambda and Recursive functions, Generators, and JSON data parsing.
In this lesson we will learn about the Scope of a Variable. Commonly a variables can be Local or Global. But it can also be Enclosing or Built-in. Python follows LEGB rule to pick the variable value.
Moreover, we will learn how to create our own module.
Complete Playlist:
If you have the basic programming knowledge and interested to learn Object-Oriented Programming in Python, check out this playlist:
Lab Manual 18 can be downloaded from here:
Review Questions:
Without running the program, guess the output of this program and explain your answer:
def test1(y):
global x
x=20
a=100*y
b=a+test2()
return b
def test2():
global x
return x/2
## Main Program ##
x=10
print(test1(1))
print(x)
#PythonProgramming #python #pythontutorial
In this lesson we will learn about the Scope of a Variable. Commonly a variables can be Local or Global. But it can also be Enclosing or Built-in. Python follows LEGB rule to pick the variable value.
Moreover, we will learn how to create our own module.
Complete Playlist:
If you have the basic programming knowledge and interested to learn Object-Oriented Programming in Python, check out this playlist:
Lab Manual 18 can be downloaded from here:
Review Questions:
Without running the program, guess the output of this program and explain your answer:
def test1(y):
global x
x=20
a=100*y
b=a+test2()
return b
def test2():
global x
return x/2
## Main Program ##
x=10
print(test1(1))
print(x)
#PythonProgramming #python #pythontutorial
Комментарии