filmov
tv
Resolving the element not interactable Error in C# Selenium WebDriver with Dropdowns
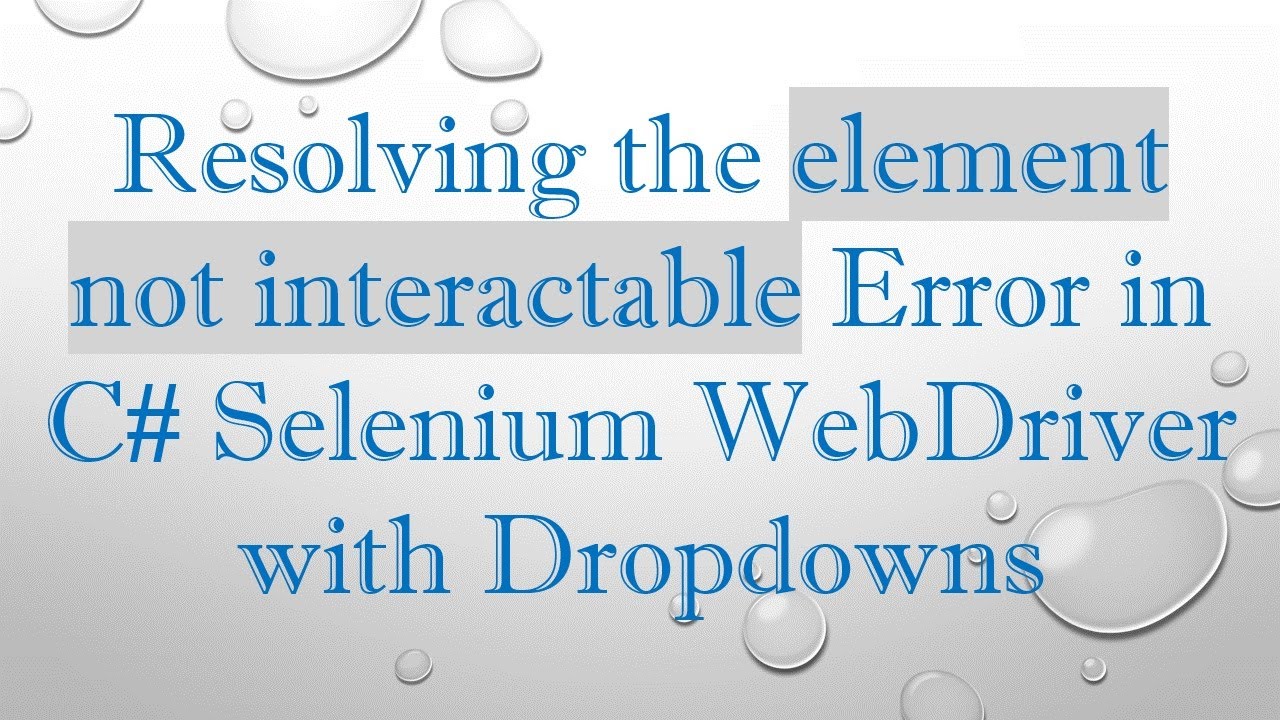
Показать описание
Learn how to fix the `element not interactable` error when handling dropdowns with C# Selenium WebDriver. Follow this detailed guide to implement effective solutions.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: C# selenium webdriver dropdown Error : element not interactable
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Resolving the element not interactable Error in C# Selenium WebDriver with Dropdowns
If you are working with C# and Selenium WebDriver, you may have encountered the frustrating element not interactable error, particularly when trying to interact with dropdown elements in your web application. This issue often arises when the dropdown is not visible or in a state that allows interaction, leading to a failed test execution.
In this guide, we will address the common causes of this error and provide a step-by-step solution to ensure your dropdowns are interactable in your tests.
Understanding the Problem
The element not interactable error typically occurs in scenarios such as:
The element is hidden or not rendered on the page.
The element is covered by another UI component.
The driver is struggling to switch to the relevant frame or context.
In your case, you are working with a complex dropdown structure that uses a combination of HTML select elements and JavaScript for rendering. Because the dropdown is initially set to display: none;, attempting to click on it or select an option directly will throw the error.
Example Code That Leads to the Error
To illustrate, let's look at the code snippet where the issue occurs:
[[See Video to Reveal this Text or Code Snippet]]
As seen above, the attempt to interact with the dropdown directly results in an error.
The Solution
To resolve the element not interactable error, we need to ensure the dropdown is visible before interacting with it. The solution involves executing JavaScript to change the element's display state and then performing the selection. Here's the step-by-step approach:
Step 1: Switch to the Appropriate Frame
Make sure you are switching to the correct iframe where the dropdown resides.
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Locate the Dropdown Element
Next, identify the dropdown element that needs to be interacted with.
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Use JavaScript to Make the Dropdown Displayable
By using JavaScript, change the dropdown's display style from none to block so that it becomes interactable.
[[See Video to Reveal this Text or Code Snippet]]
Step 4: Select the Desired Option
Once the dropdown is visible, you can use the SelectElement class to select the desired option.
[[See Video to Reveal this Text or Code Snippet]]
Complete Revised Code
Here's how the complete working code looks after incorporating the solution:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
With this guide, you should now be able to successfully resolve the element not interactable error when working with dropdowns in C# Selenium WebDriver. Remember to adjust the visibility of elements via JavaScript when necessary and ensure that your test waits for elements to be interactable.
Using these strategies will not only make your tests more resilient but will also enhance your overall automation skills.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: C# selenium webdriver dropdown Error : element not interactable
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Resolving the element not interactable Error in C# Selenium WebDriver with Dropdowns
If you are working with C# and Selenium WebDriver, you may have encountered the frustrating element not interactable error, particularly when trying to interact with dropdown elements in your web application. This issue often arises when the dropdown is not visible or in a state that allows interaction, leading to a failed test execution.
In this guide, we will address the common causes of this error and provide a step-by-step solution to ensure your dropdowns are interactable in your tests.
Understanding the Problem
The element not interactable error typically occurs in scenarios such as:
The element is hidden or not rendered on the page.
The element is covered by another UI component.
The driver is struggling to switch to the relevant frame or context.
In your case, you are working with a complex dropdown structure that uses a combination of HTML select elements and JavaScript for rendering. Because the dropdown is initially set to display: none;, attempting to click on it or select an option directly will throw the error.
Example Code That Leads to the Error
To illustrate, let's look at the code snippet where the issue occurs:
[[See Video to Reveal this Text or Code Snippet]]
As seen above, the attempt to interact with the dropdown directly results in an error.
The Solution
To resolve the element not interactable error, we need to ensure the dropdown is visible before interacting with it. The solution involves executing JavaScript to change the element's display state and then performing the selection. Here's the step-by-step approach:
Step 1: Switch to the Appropriate Frame
Make sure you are switching to the correct iframe where the dropdown resides.
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Locate the Dropdown Element
Next, identify the dropdown element that needs to be interacted with.
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Use JavaScript to Make the Dropdown Displayable
By using JavaScript, change the dropdown's display style from none to block so that it becomes interactable.
[[See Video to Reveal this Text or Code Snippet]]
Step 4: Select the Desired Option
Once the dropdown is visible, you can use the SelectElement class to select the desired option.
[[See Video to Reveal this Text or Code Snippet]]
Complete Revised Code
Here's how the complete working code looks after incorporating the solution:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
With this guide, you should now be able to successfully resolve the element not interactable error when working with dropdowns in C# Selenium WebDriver. Remember to adjust the visibility of elements via JavaScript when necessary and ensure that your test waits for elements to be interactable.
Using these strategies will not only make your tests more resilient but will also enhance your overall automation skills.