filmov
tv
Solving the no such element Error in Selenium Python: A Guide to Handling Missing Elements
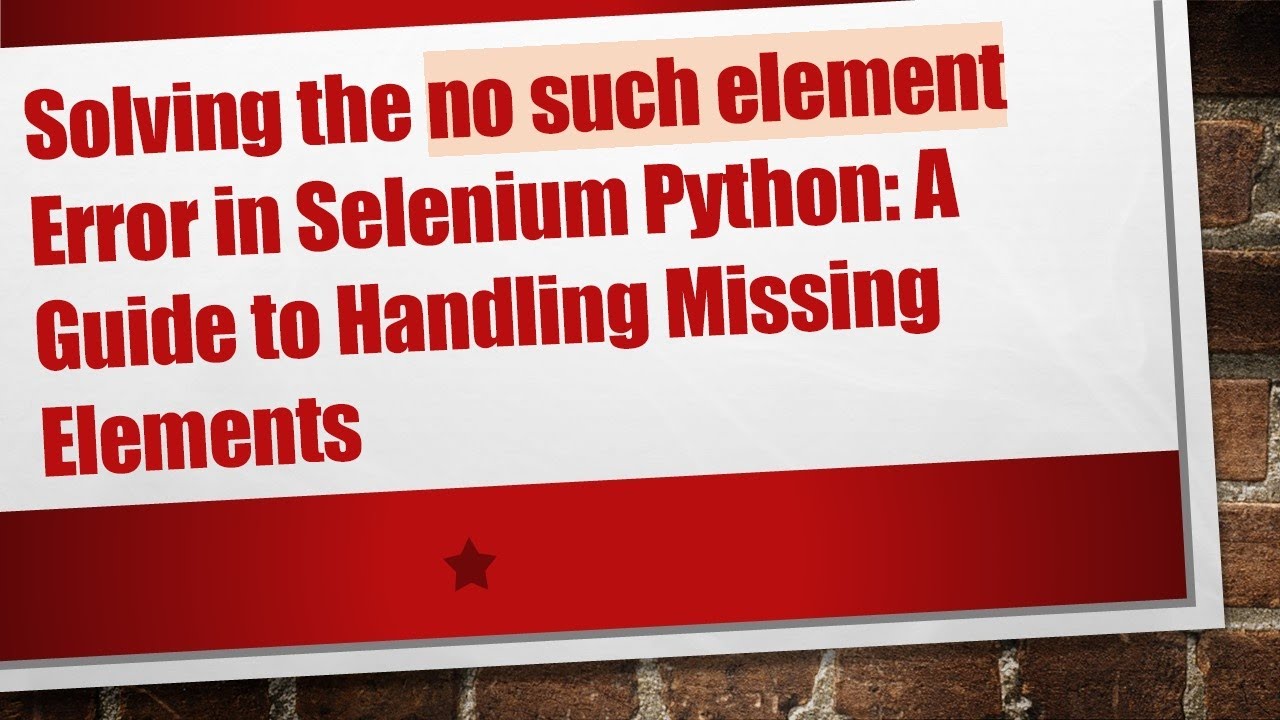
Показать описание
Learn how to effectively handle the `no such element` error in Selenium when trying to locate an element like the “Add to wishlist” button on a webpage. This guide offers simple and practical solutions.
---
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Solving the no such element Error in Selenium Python
When working with Selenium in Python, you may encounter the frustrating error message: no such element: Unable to locate element. This issue often arises when you try to interact with a webpage element that may not be present. For instance, consider a scenario where you are trying to click an "Add to wishlist" button but you receive this error instead. In this guide, we will explain the problem and provide a solution to handle it effectively.
Understanding the Issue
In the provided scenario, the code attempts to find the "Add to wishlist" button using the following line:
[[See Video to Reveal this Text or Code Snippet]]
However, if there are products listed on the webpage that do not have this button available, Selenium will raise a NoSuchElementException. This is likely the source of the error you're experiencing.
Why Does This Happen?
Several factors can lead to this error:
The element is not present in the DOM.
Timing issues where the element has not rendered yet.
Variability in the product list where some products may not have the desired button.
Implementing a Solution
To avoid the error and improve user experience, we can implement simple error handling in our code. Below are the steps to do this effectively.
Step 1: Import the Required Exception
Before you can catch the NoSuchElementException, make sure to import it:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Use a Try-Except Block
Wrap the code that attempts to find and click the wish list button in a try-except block. Here’s how to modify your code:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
Try Block: This is where you attempt to locate and interact with the "Add to wishlist" button.
Except Block: If the button is not found, instead of crashing, your script will print a friendly message indicating that no button is available.
Benefits of Error Handling
User Experience: Provides feedback rather than abruptly ending your script.
Robust Code: Makes your Selenium script more adaptable to changes in the webpage structure.
Conclusion
Handling exceptions in your Selenium automation scripts is crucial for building robust applications. By accounting for the possibility that certain elements may not be present on the page, you can avoid common pitfalls like the no such element error. Implementing the simple solution we discussed can enhance the reliability of your web scraping or automated testing scripts, making your journey through Selenium a lot smoother.
Happy coding, and may your Selenium scripts run more seamlessly from now on!
---
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Solving the no such element Error in Selenium Python
When working with Selenium in Python, you may encounter the frustrating error message: no such element: Unable to locate element. This issue often arises when you try to interact with a webpage element that may not be present. For instance, consider a scenario where you are trying to click an "Add to wishlist" button but you receive this error instead. In this guide, we will explain the problem and provide a solution to handle it effectively.
Understanding the Issue
In the provided scenario, the code attempts to find the "Add to wishlist" button using the following line:
[[See Video to Reveal this Text or Code Snippet]]
However, if there are products listed on the webpage that do not have this button available, Selenium will raise a NoSuchElementException. This is likely the source of the error you're experiencing.
Why Does This Happen?
Several factors can lead to this error:
The element is not present in the DOM.
Timing issues where the element has not rendered yet.
Variability in the product list where some products may not have the desired button.
Implementing a Solution
To avoid the error and improve user experience, we can implement simple error handling in our code. Below are the steps to do this effectively.
Step 1: Import the Required Exception
Before you can catch the NoSuchElementException, make sure to import it:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Use a Try-Except Block
Wrap the code that attempts to find and click the wish list button in a try-except block. Here’s how to modify your code:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
Try Block: This is where you attempt to locate and interact with the "Add to wishlist" button.
Except Block: If the button is not found, instead of crashing, your script will print a friendly message indicating that no button is available.
Benefits of Error Handling
User Experience: Provides feedback rather than abruptly ending your script.
Robust Code: Makes your Selenium script more adaptable to changes in the webpage structure.
Conclusion
Handling exceptions in your Selenium automation scripts is crucial for building robust applications. By accounting for the possibility that certain elements may not be present on the page, you can avoid common pitfalls like the no such element error. Implementing the simple solution we discussed can enhance the reliability of your web scraping or automated testing scripts, making your journey through Selenium a lot smoother.
Happy coding, and may your Selenium scripts run more seamlessly from now on!