filmov
tv
Simple ESP32 Project programming with Arduino (Complete ESP project) Led Blinking
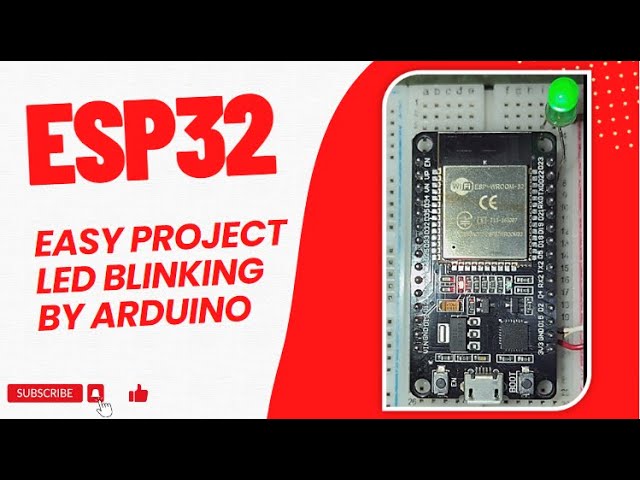
Показать описание
Project Overview:
This project demonstrates how to create a timing-based LED lighting system using an ESP32 microcontroller and the Arduino programming environment. The goal is to develop a smart lighting solution that can automatically control LED lights based on a predefined schedule or timing.
Key Features:
ESP32 Microcontroller: The project utilizes the powerful ESP32 microcontroller, which provides Wi-Fi and Bluetooth connectivity, dual-core processing, and low-power capabilities.
Arduino Programming: The project uses the Arduino IDE and libraries, making it accessible to a wide range of users familiar with the Arduino ecosystem.
Timing-based LED Control: The system allows users to set specific on/off times for the LED lights, enabling automatic and scheduled lighting control.
Customizable Lighting Patterns: The project can be extended to include various lighting patterns, animations, or color effects to enhance the visual appeal of the lighting system.
Optional Sensor Integration: The project can be further expanded to incorporate sensors (e.g., motion, light, or temperature sensors) to enable adaptive lighting based on environmental conditions.
User Interface: The project may include a user interface, either through a mobile app, web interface, or physical buttons/switches, to allow users to easily configure and control the lighting system.
Hardware Requirements:
ESP32 development board (e.g., ESP32-WROOM-32, ESP32-WROVER)
LED strip or individual LED modules
Breadboard and jumper wires
Software Requirements:
Arduino IDE
ESP32 board package for Arduino
Arduino libraries for timing, LED control, and optional sensor integration
Arduino Code:
void setup()
{
pinMode(22, OUTPUT); // Set GPIO22 as digital output pin
}
void loop()
{
digitalWrite(22, HIGH); // Set GPIO22 active high
delay(1000); // delay of one second
digitalWrite(22, LOW); // Set GPIO22 active low
delay(1000); // delay of one second
}
Project Steps:
Set up the development environment by installing the Arduino IDE and configuring it to work with the ESP32 board.
Write the Arduino code to handle the timing-based LED control, including setting up the on/off schedules and managing the LED outputs.
Implement the user interface, either through physical buttons/switches or a web-based/mobile application, to allow users to configure the lighting schedule and settings.
Test the project thoroughly, ensuring the timing-based LED control works as expected and the user interface is intuitive and responsive.
Optimize the code for power efficiency and performance, taking advantage of the ESP32's low-power features.
Package the project and provide detailed instructions for assembly, configuration, and usage.
This comprehensive ESP32 project covers the essential aspects of creating a timing-based LED lighting system, from hardware setup to software implementation and user interface design. It can serve as a valuable learning resource for individuals interested in exploring the capabilities of the ESP32 microcontroller and developing smart home or IoT-based lighting solutions.
This project demonstrates how to create a timing-based LED lighting system using an ESP32 microcontroller and the Arduino programming environment. The goal is to develop a smart lighting solution that can automatically control LED lights based on a predefined schedule or timing.
Key Features:
ESP32 Microcontroller: The project utilizes the powerful ESP32 microcontroller, which provides Wi-Fi and Bluetooth connectivity, dual-core processing, and low-power capabilities.
Arduino Programming: The project uses the Arduino IDE and libraries, making it accessible to a wide range of users familiar with the Arduino ecosystem.
Timing-based LED Control: The system allows users to set specific on/off times for the LED lights, enabling automatic and scheduled lighting control.
Customizable Lighting Patterns: The project can be extended to include various lighting patterns, animations, or color effects to enhance the visual appeal of the lighting system.
Optional Sensor Integration: The project can be further expanded to incorporate sensors (e.g., motion, light, or temperature sensors) to enable adaptive lighting based on environmental conditions.
User Interface: The project may include a user interface, either through a mobile app, web interface, or physical buttons/switches, to allow users to easily configure and control the lighting system.
Hardware Requirements:
ESP32 development board (e.g., ESP32-WROOM-32, ESP32-WROVER)
LED strip or individual LED modules
Breadboard and jumper wires
Software Requirements:
Arduino IDE
ESP32 board package for Arduino
Arduino libraries for timing, LED control, and optional sensor integration
Arduino Code:
void setup()
{
pinMode(22, OUTPUT); // Set GPIO22 as digital output pin
}
void loop()
{
digitalWrite(22, HIGH); // Set GPIO22 active high
delay(1000); // delay of one second
digitalWrite(22, LOW); // Set GPIO22 active low
delay(1000); // delay of one second
}
Project Steps:
Set up the development environment by installing the Arduino IDE and configuring it to work with the ESP32 board.
Write the Arduino code to handle the timing-based LED control, including setting up the on/off schedules and managing the LED outputs.
Implement the user interface, either through physical buttons/switches or a web-based/mobile application, to allow users to configure the lighting schedule and settings.
Test the project thoroughly, ensuring the timing-based LED control works as expected and the user interface is intuitive and responsive.
Optimize the code for power efficiency and performance, taking advantage of the ESP32's low-power features.
Package the project and provide detailed instructions for assembly, configuration, and usage.
This comprehensive ESP32 project covers the essential aspects of creating a timing-based LED lighting system, from hardware setup to software implementation and user interface design. It can serve as a valuable learning resource for individuals interested in exploring the capabilities of the ESP32 microcontroller and developing smart home or IoT-based lighting solutions.