filmov
tv
Simplifying JavaScript Functions: How to Shorten Probabilities in Your Code
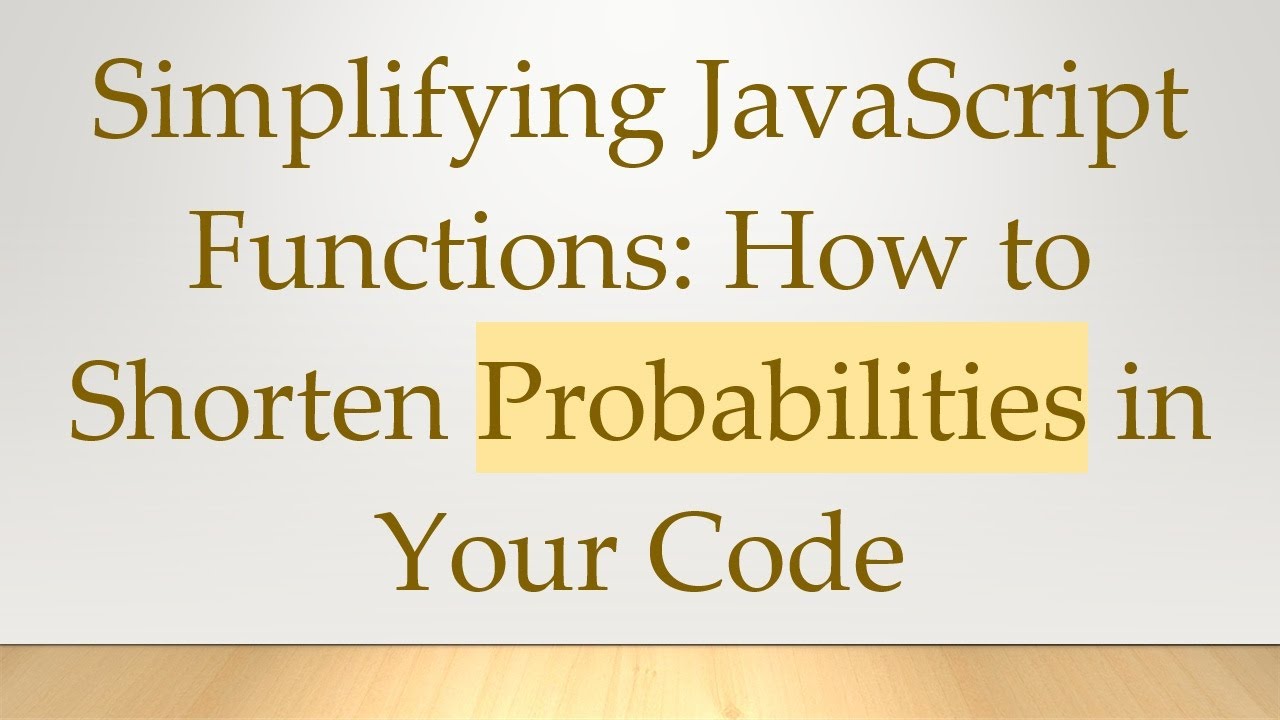
Показать описание
Discover effective ways to simplify JavaScript functions and improve code readability. Learn best practices for handling parameters in a more organized manner.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: how to shorten js probabilities for functions
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Simplifying JavaScript Functions: How to Shorten Probabilities in Your Code
When dealing with JavaScript functions, especially those that require multiple parameters, the code can quickly become complex and confusing. If you've ever found yourself faced with a lengthy function that seems to take more steps than necessary, you're not alone. Today, we'll delve into how to streamline your JavaScript functions, making them not only simpler but also easier to maintain.
The Problem: Complex Function Parameters
Consider the following function that aims to greet a user based on their name, age, and hiring status:
[[See Video to Reveal this Text or Code Snippet]]
In its current form, this function handles parameters in a convoluted way, making it challenging to read and maintain. Each time you manipulate the function's parameters, you're left wondering about their order and types. Additionally, it lacks a straightforward method for sorting or organizing the input.
The Solution: Using Objects and Destructuring
One of the best practices to simplify functions in JavaScript is to pass an object as a parameter instead of individual variables. This way, you can use destructuring to assign default values and improve code clarity. Here’s how you can rewrite the function:
Step 1: Define the Function Using an Object
Instead of using three separate parameters, you can define the function to accept an object. Here’s the modified version:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Calling the Function
With the new structure, you can call the function in various ways, passing objects with the parameters you need. Here are some examples:
[[See Video to Reveal this Text or Code Snippet]]
This method not only cleans up the code but also makes it scalable for future modifications. Adding new features like an email field or a position would be straightforward and wouldn't require drastic changes to the function's parameter structure.
Alternative Approach: Using Arrays and find Method
If you are unable to change the structure to accept an object for some reason, you can also handle the parameters using the spread operator (...) to collect arguments into an array, then use the .find() method to identify their types. Here’s how that looks:
Collecting Parameters into an Array
[[See Video to Reveal this Text or Code Snippet]]
Example Outputs
You can also call this function similarly:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion: Cleaner Code, Better Maintenance
By using object destructuring or collecting parameters into an array, you can simplify the way your JavaScript functions handle inputs. Not only does this make it easier to read and maintain your code, but it also enhances the overall functionality and scalability of your applications. Remember, good coding practices lead to better and cleaner code!
In conclusion, whether you're just starting your programming journey or you're a seasoned developer, considering how you structure your function parameters can make a significant difference in your coding experience. Simplifying your JavaScript functions will save you time and headaches in the long run.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: how to shorten js probabilities for functions
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Simplifying JavaScript Functions: How to Shorten Probabilities in Your Code
When dealing with JavaScript functions, especially those that require multiple parameters, the code can quickly become complex and confusing. If you've ever found yourself faced with a lengthy function that seems to take more steps than necessary, you're not alone. Today, we'll delve into how to streamline your JavaScript functions, making them not only simpler but also easier to maintain.
The Problem: Complex Function Parameters
Consider the following function that aims to greet a user based on their name, age, and hiring status:
[[See Video to Reveal this Text or Code Snippet]]
In its current form, this function handles parameters in a convoluted way, making it challenging to read and maintain. Each time you manipulate the function's parameters, you're left wondering about their order and types. Additionally, it lacks a straightforward method for sorting or organizing the input.
The Solution: Using Objects and Destructuring
One of the best practices to simplify functions in JavaScript is to pass an object as a parameter instead of individual variables. This way, you can use destructuring to assign default values and improve code clarity. Here’s how you can rewrite the function:
Step 1: Define the Function Using an Object
Instead of using three separate parameters, you can define the function to accept an object. Here’s the modified version:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Calling the Function
With the new structure, you can call the function in various ways, passing objects with the parameters you need. Here are some examples:
[[See Video to Reveal this Text or Code Snippet]]
This method not only cleans up the code but also makes it scalable for future modifications. Adding new features like an email field or a position would be straightforward and wouldn't require drastic changes to the function's parameter structure.
Alternative Approach: Using Arrays and find Method
If you are unable to change the structure to accept an object for some reason, you can also handle the parameters using the spread operator (...) to collect arguments into an array, then use the .find() method to identify their types. Here’s how that looks:
Collecting Parameters into an Array
[[See Video to Reveal this Text or Code Snippet]]
Example Outputs
You can also call this function similarly:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion: Cleaner Code, Better Maintenance
By using object destructuring or collecting parameters into an array, you can simplify the way your JavaScript functions handle inputs. Not only does this make it easier to read and maintain your code, but it also enhances the overall functionality and scalability of your applications. Remember, good coding practices lead to better and cleaner code!
In conclusion, whether you're just starting your programming journey or you're a seasoned developer, considering how you structure your function parameters can make a significant difference in your coding experience. Simplifying your JavaScript functions will save you time and headaches in the long run.