filmov
tv
Simplifying JavaScript Functions with try/catch/finally for Cleaner Code
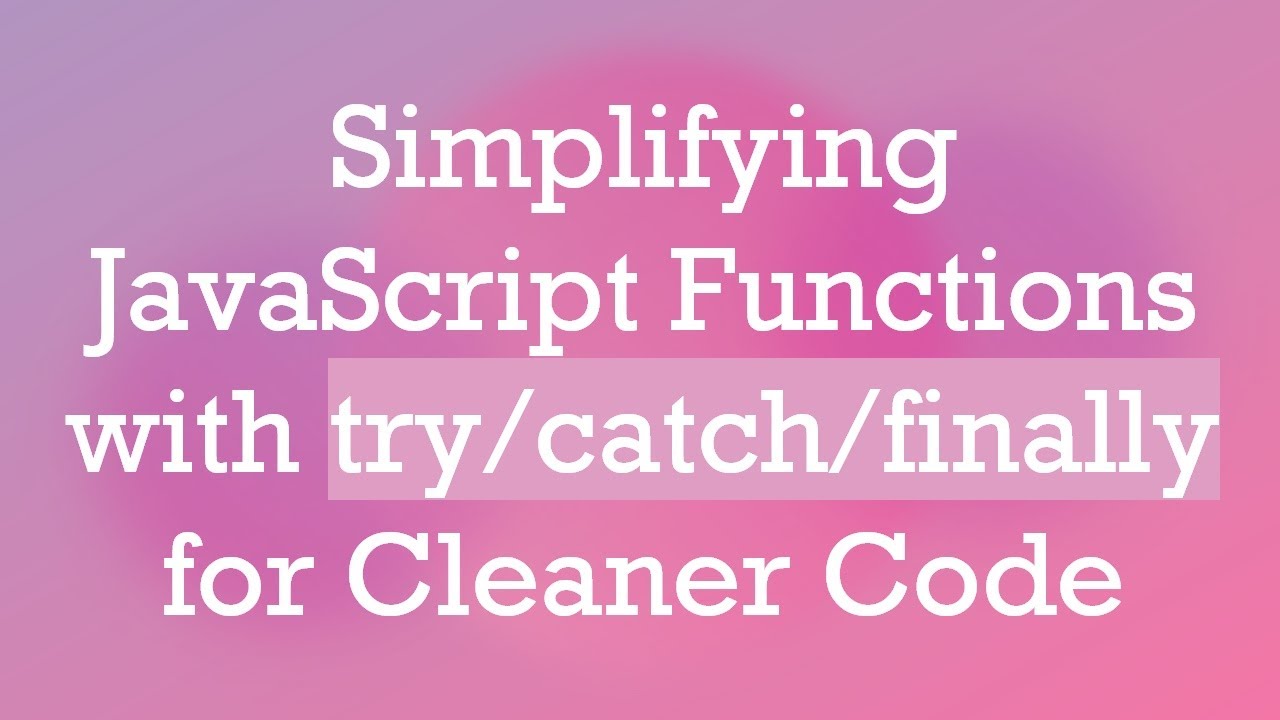
Показать описание
Learn how to streamline your JavaScript functions using `try/catch/finally` to avoid repetitive code and ensure certain processes always run.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: way to always call a function without repeating code inside a function with guard clauses
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Simplifying JavaScript Functions with try/catch/finally for Cleaner Code
In JavaScript development, writing clean and efficient code is essential. However, when dealing with multiple conditional checks within functions, it can lead to repeated code and decreased readability. A common query among developers is how to simplify such functions while ensuring that specific actions execute regardless of the conditions. This is where the try/catch/finally statement comes into play.
The Problem with Conditional Code
Consider a function that checks multiple conditions and executes different blocks of code based on those conditions. This often leads to repetitive code if multiple conditions require the same concluding action to occur. Here is an example of such a scenario:
[[See Video to Reveal this Text or Code Snippet]]
In this instance, you can see that the doSomething() function may need to be executed in various places, leading to code duplication and potential errors if changes are needed later.
The Solution: Using try/catch/finally
The try/catch/finally statement in JavaScript helps manage exceptions and ensure certain blocks of code run no matter what. By wrapping your function logic in a try block and placing the final action in a finally block, you can simplify your code significantly.
Step-by-Step Explanation
Define the Function: Create a self-executing function inside the try block to maintain scope and execute conditional statements.
Implement Conditions: Inside the function, use your condition checks without worrying about repetitive calls to the concluding function.
Final Action: Place the function that needs to be executed regardless of the conditions inside the finally block.
Example Code
Here’s how the revised function looks using try/catch/finally:
[[See Video to Reveal this Text or Code Snippet]]
Key Benefits
Reduced Code Duplication: The doSomething() function is executed only once, regardless of the conditions met.
Improved Readability: The functionality of your code is clearer, making it easier to understand and maintain.
Error Handling: The try/catch block allows you to handle potential errors more gracefully.
Conclusion
By utilizing the try/catch/finally structure in JavaScript, you not only simplify your code but also enhance its maintainability. This approach helps avoid redundancy and declutters functions that would otherwise require complex conditional checks. Embracing this method can significantly improve your coding practices and lead to cleaner, more efficient JavaScript applications.
By following these steps, you can take full advantage of modern JavaScript functionalities, making your development process smoother and more productive.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: way to always call a function without repeating code inside a function with guard clauses
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Simplifying JavaScript Functions with try/catch/finally for Cleaner Code
In JavaScript development, writing clean and efficient code is essential. However, when dealing with multiple conditional checks within functions, it can lead to repeated code and decreased readability. A common query among developers is how to simplify such functions while ensuring that specific actions execute regardless of the conditions. This is where the try/catch/finally statement comes into play.
The Problem with Conditional Code
Consider a function that checks multiple conditions and executes different blocks of code based on those conditions. This often leads to repetitive code if multiple conditions require the same concluding action to occur. Here is an example of such a scenario:
[[See Video to Reveal this Text or Code Snippet]]
In this instance, you can see that the doSomething() function may need to be executed in various places, leading to code duplication and potential errors if changes are needed later.
The Solution: Using try/catch/finally
The try/catch/finally statement in JavaScript helps manage exceptions and ensure certain blocks of code run no matter what. By wrapping your function logic in a try block and placing the final action in a finally block, you can simplify your code significantly.
Step-by-Step Explanation
Define the Function: Create a self-executing function inside the try block to maintain scope and execute conditional statements.
Implement Conditions: Inside the function, use your condition checks without worrying about repetitive calls to the concluding function.
Final Action: Place the function that needs to be executed regardless of the conditions inside the finally block.
Example Code
Here’s how the revised function looks using try/catch/finally:
[[See Video to Reveal this Text or Code Snippet]]
Key Benefits
Reduced Code Duplication: The doSomething() function is executed only once, regardless of the conditions met.
Improved Readability: The functionality of your code is clearer, making it easier to understand and maintain.
Error Handling: The try/catch block allows you to handle potential errors more gracefully.
Conclusion
By utilizing the try/catch/finally structure in JavaScript, you not only simplify your code but also enhance its maintainability. This approach helps avoid redundancy and declutters functions that would otherwise require complex conditional checks. Embracing this method can significantly improve your coding practices and lead to cleaner, more efficient JavaScript applications.
By following these steps, you can take full advantage of modern JavaScript functionalities, making your development process smoother and more productive.