filmov
tv
Check if a binary tree is binary search tree or not
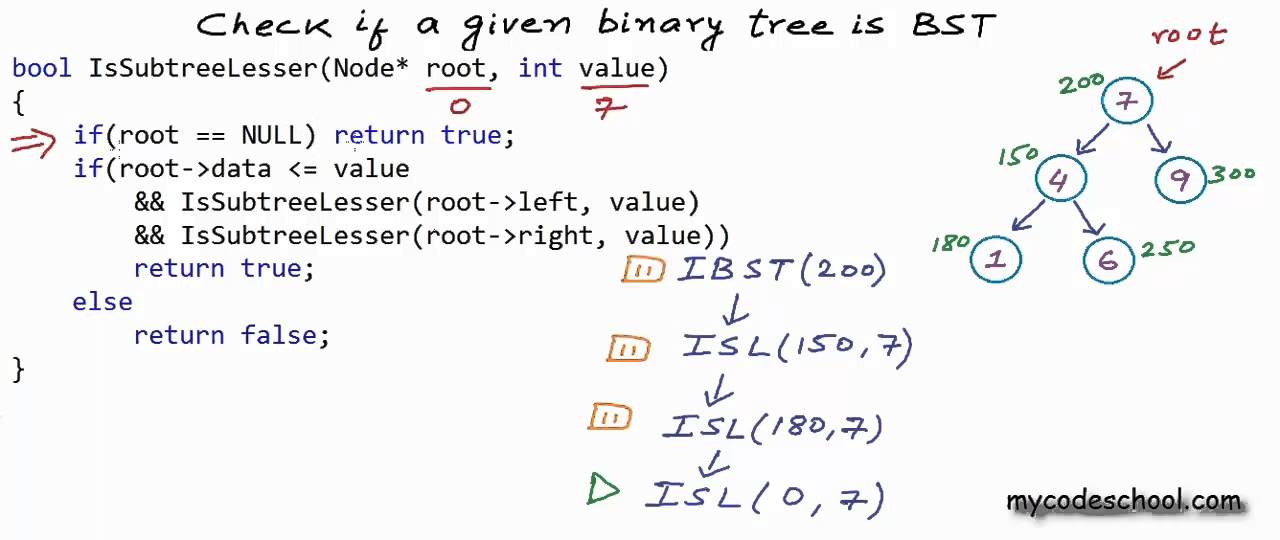
Показать описание
See complete series on data structures here:
In this lesson, we have written a program in C/C++ to verify whether a given binary tree is binary search tree or not.
In this lesson, we have written a program in C/C++ to verify whether a given binary tree is binary search tree or not.
Check if a binary tree is binary search tree or not
Check Completeness of a Binary Tree - Leetcode 958 - Python
L46. Check if a tree is a BST or BT | Validate a BST
Check if Binary Tree is Binary Search Tree
Validate Binary Search Tree - Depth First Search - Leetcode 98
Balanced Binary Tree - Leetcode 110 - Python
Test If A Binary Tree Is Height Balanced ('Balanced Binary Tree' on LeetCode)
Check if two binary trees are identical (Algorithm/code/program)
Build a Web API From Scratch - Principal Software Engineering Manager AMA
Check if a tree is bst or not
How To Validate If A Binary Tree Is A Binary Search Tree implemented in C#
Test If A Binary Tree Is Symmetric ('Symmetric Tree' on Leetcode)
Check if two binary trees are identical (Algorithm/code/program)
Determine if a binary tree is height-balanced | GeeksforGeeks
Check if a binary tree is subtree of another binary tree | Part 1 | GeeksforGeeks
Check If Two Binary Trees Are Equal
BST - 20: Check if given Binary Tree is BST
Checking if a binary tree is a binary search tree or not!
check if a tree is subtree of another tree
Binary Tree - 71: Check if given Binary Tree is BST
Check whether a binary tree is a full binary tree or not | GeeksforGeeks
Check Mirror of Binary Tree
Binary Tree - 37: Check if two Binary Trees are Isomorphic
Binary Trees - Data Structures Explained
Комментарии