filmov
tv
Resolving the TypeError: 'list' object is not callable in Python Functions
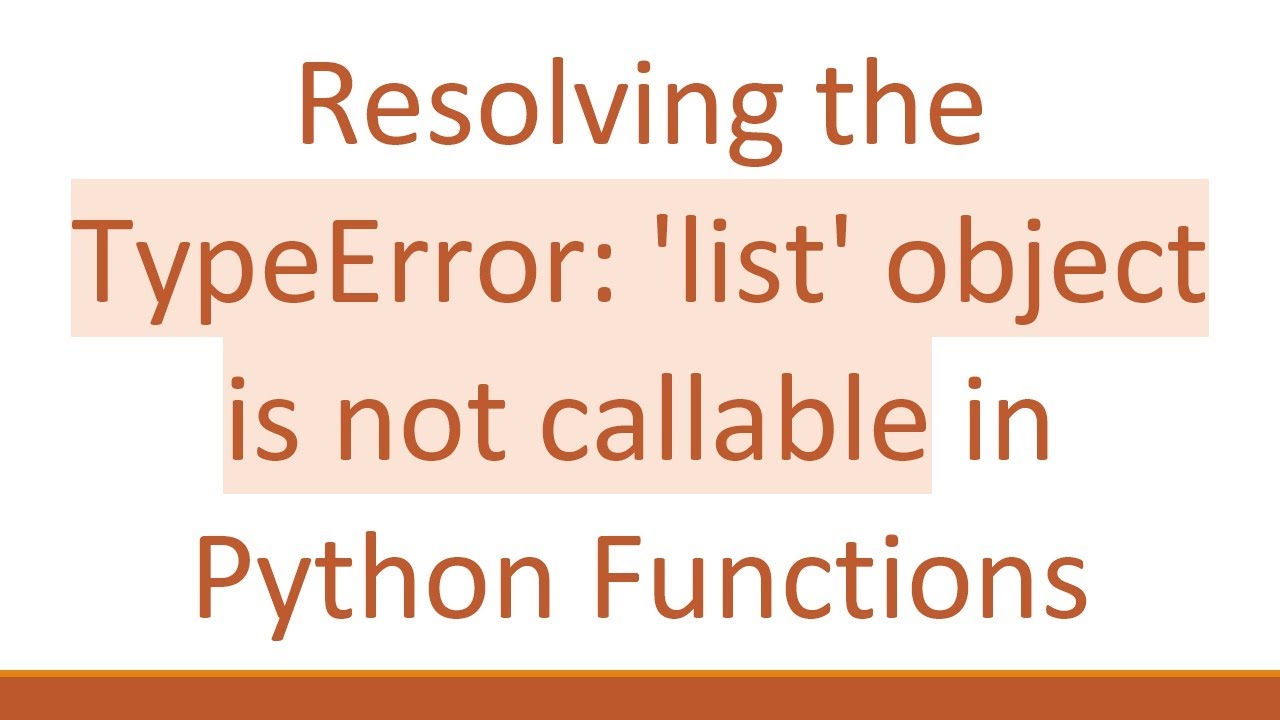
Показать описание
Learn how to solve the common Python error that arises when passing a function to another function, and ensure your algorithms run smoothly.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: list object not callable calling a function whose parameter is also a function with an array
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the TypeError: 'list' object is not callable Error in Python
When working with Python, you might come across various errors, and one of the more notorious ones is the TypeError: 'list' object is not callable. This error typically occurs when you try to invoke a list as if it were a function. A common scenario for this error arises when dealing with function parameters that also have data of similar type, like arrays or lists.
In this guide, we're going to dissect this error, provide clarity on what causes it, and give you a clear, practical solution to prevent it from happening.
The Problem
Let's break down the provided code snippet where the error arises. An index shows where the error occurred:
[[See Video to Reveal this Text or Code Snippet]]
What Happened?
The line timeAlgo(insertionSort(arr)) is problematic and results in insertionSort(arr) running first. This executes the insertionSort function which returns a sorted list—not a function reference. When timeAlgo tries to execute algo(), it fails because algo holds a list instead of a callable function.
The Solution
To fix this issue, we'll adjust the way we handle function and argument passing in our timeAlgo function. Here’s how we can do that step by step:
Step 1: Modify the timeAlgo function
We need to ensure that timeAlgo accepts a function and its arguments separately:
[[See Video to Reveal this Text or Code Snippet]]
In this solution, we accept any additional arguments through *args, which makes the function more flexible.
Step 2: Call timeAlgo with the function and parameters separately
Instead of invoking insertionSort directly, we pass the function and the array separately to timeAlgo:
[[See Video to Reveal this Text or Code Snippet]]
Complete Code Fix
Here's the complete working code after the modifications:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
In summary, the TypeError: 'list' object is not callable can be easily avoided by understanding the difference between invoking functions and passing them as references. By ensuring that we call our functions appropriately, we can avoid such pitfalls.
Next time you run into this issue, remember the key takeaway—as long as you pass the function reference and its arguments separately, you can run your algorithms smoothly without encountering this common error.
Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: list object not callable calling a function whose parameter is also a function with an array
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the TypeError: 'list' object is not callable Error in Python
When working with Python, you might come across various errors, and one of the more notorious ones is the TypeError: 'list' object is not callable. This error typically occurs when you try to invoke a list as if it were a function. A common scenario for this error arises when dealing with function parameters that also have data of similar type, like arrays or lists.
In this guide, we're going to dissect this error, provide clarity on what causes it, and give you a clear, practical solution to prevent it from happening.
The Problem
Let's break down the provided code snippet where the error arises. An index shows where the error occurred:
[[See Video to Reveal this Text or Code Snippet]]
What Happened?
The line timeAlgo(insertionSort(arr)) is problematic and results in insertionSort(arr) running first. This executes the insertionSort function which returns a sorted list—not a function reference. When timeAlgo tries to execute algo(), it fails because algo holds a list instead of a callable function.
The Solution
To fix this issue, we'll adjust the way we handle function and argument passing in our timeAlgo function. Here’s how we can do that step by step:
Step 1: Modify the timeAlgo function
We need to ensure that timeAlgo accepts a function and its arguments separately:
[[See Video to Reveal this Text or Code Snippet]]
In this solution, we accept any additional arguments through *args, which makes the function more flexible.
Step 2: Call timeAlgo with the function and parameters separately
Instead of invoking insertionSort directly, we pass the function and the array separately to timeAlgo:
[[See Video to Reveal this Text or Code Snippet]]
Complete Code Fix
Here's the complete working code after the modifications:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
In summary, the TypeError: 'list' object is not callable can be easily avoided by understanding the difference between invoking functions and passing them as references. By ensuring that we call our functions appropriately, we can avoid such pitfalls.
Next time you run into this issue, remember the key takeaway—as long as you pass the function reference and its arguments separately, you can run your algorithms smoothly without encountering this common error.
Happy coding!