filmov
tv
How to Fix the TypeError: 'list' object is not callable in Python OpenCV Face Detection
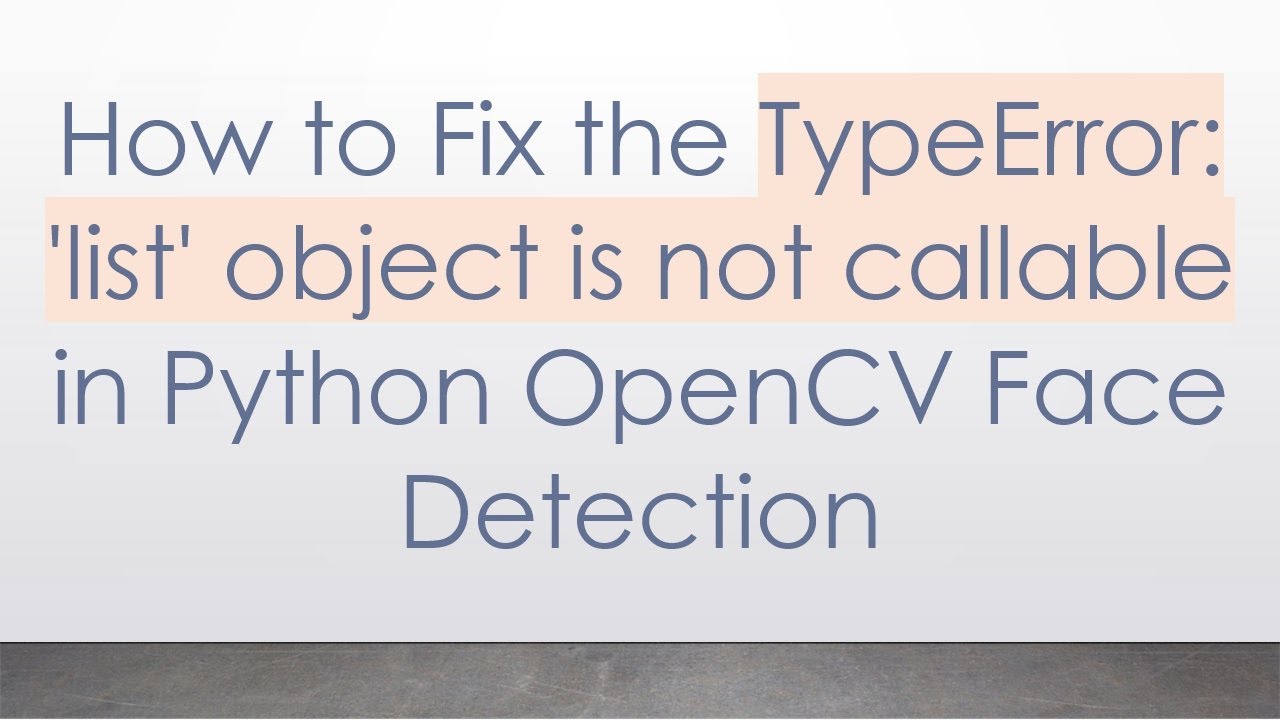
Показать описание
Solve the common Python error "TypeError: 'list' object is not callable" when using OpenCV's face mesh module with our detailed guide and examples.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: TypeError : 'list' object is not callable
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Fix the TypeError: 'list' object is not callable in Python OpenCV Face Detection
If you're working with the face mesh module of OpenCV to detect faces and run into the frustrating issue of TypeError: 'list' object is not callable, you’re not alone. This commonly arises when trying to retrieve data from a list, leading to confusion and halted progress in your code. In this guide, we'll delve into this error, uncover its cause, and walk through a simple solution to get your face detection program running smoothly.
Understanding the Problem
When you attempt to access an item from a list in Python, the syntax can be a bit tricky for beginners. The specific error you are encountering typically indicates that the code is trying to treat a list like a function.
For example:
[[See Video to Reveal this Text or Code Snippet]]
In the line above, the issue lies in the way you're trying to access an element from the landmark_points list. The parentheses () imply that you want to call it as a function, which is not valid since landmark_points is indeed a list.
What Went Wrong?
Here’s the breakdown of the mistake:
Mistaken Syntax: Your code attempts to call landmark_points as if it were a function (landmark_points(1)), which leads to the TypeError.
Accessibility of Elements: Instead, you should be using square brackets [] to access elements directly by their index.
The Solution
Correcting the Code
To fix this error, you should replace landmark_points(1) with landmark_points[1]. However, note that landmark_points[1] refers to the second element in the list, and you may actually want the first element which can be accessed with landmark_points[0].
Here’s how you can rewrite that specific line of code:
[[See Video to Reveal this Text or Code Snippet]]
This change should resolve the error you're facing.
Complete Code Example
Here’s the corrected block of your code that includes the fix:
[[See Video to Reveal this Text or Code Snippet]]
Key Takeaways
Always use square brackets [] to access elements within a list.
Be mindful of index positions, as Python uses zero-based indexing (i.e., the first item is at index 0).
If you're unsure which index to use or how many elements are present in your list, a simple print statement can help clarify.
Conclusion
By understanding how to properly access elements in a list, you can resolve the TypeError: 'list' object is not callable issue effectively. With the adjustments made, your face detection implementation should now work correctly, allowing you to extract and utilize the coordinates of detected landmarks for your projects.
So, get back to coding, and enjoy the process of building applications with OpenCV!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: TypeError : 'list' object is not callable
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Fix the TypeError: 'list' object is not callable in Python OpenCV Face Detection
If you're working with the face mesh module of OpenCV to detect faces and run into the frustrating issue of TypeError: 'list' object is not callable, you’re not alone. This commonly arises when trying to retrieve data from a list, leading to confusion and halted progress in your code. In this guide, we'll delve into this error, uncover its cause, and walk through a simple solution to get your face detection program running smoothly.
Understanding the Problem
When you attempt to access an item from a list in Python, the syntax can be a bit tricky for beginners. The specific error you are encountering typically indicates that the code is trying to treat a list like a function.
For example:
[[See Video to Reveal this Text or Code Snippet]]
In the line above, the issue lies in the way you're trying to access an element from the landmark_points list. The parentheses () imply that you want to call it as a function, which is not valid since landmark_points is indeed a list.
What Went Wrong?
Here’s the breakdown of the mistake:
Mistaken Syntax: Your code attempts to call landmark_points as if it were a function (landmark_points(1)), which leads to the TypeError.
Accessibility of Elements: Instead, you should be using square brackets [] to access elements directly by their index.
The Solution
Correcting the Code
To fix this error, you should replace landmark_points(1) with landmark_points[1]. However, note that landmark_points[1] refers to the second element in the list, and you may actually want the first element which can be accessed with landmark_points[0].
Here’s how you can rewrite that specific line of code:
[[See Video to Reveal this Text or Code Snippet]]
This change should resolve the error you're facing.
Complete Code Example
Here’s the corrected block of your code that includes the fix:
[[See Video to Reveal this Text or Code Snippet]]
Key Takeaways
Always use square brackets [] to access elements within a list.
Be mindful of index positions, as Python uses zero-based indexing (i.e., the first item is at index 0).
If you're unsure which index to use or how many elements are present in your list, a simple print statement can help clarify.
Conclusion
By understanding how to properly access elements in a list, you can resolve the TypeError: 'list' object is not callable issue effectively. With the adjustments made, your face detection implementation should now work correctly, allowing you to extract and utilize the coordinates of detected landmarks for your projects.
So, get back to coding, and enjoy the process of building applications with OpenCV!