filmov
tv
[Python Programming Basics to Advanced]: Lambda Functions and Sorting Data | Lab 29
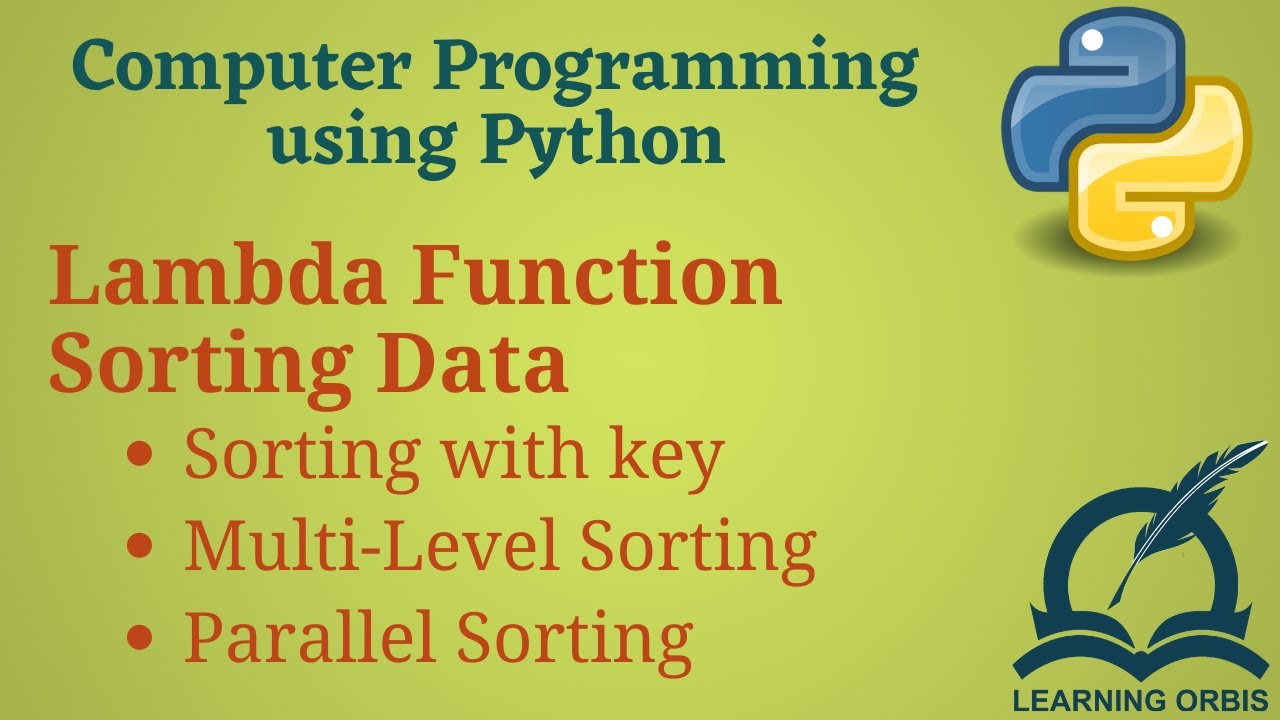
Показать описание
This Python programming playlist is designed to take beginners with zero programming experience to an expert level. The course covers installation, basic syntax, practical scenarios, and efficient logic building. The course material includes PDF handouts, review questions, and covers a wide range of topics, from data types to advanced functions like Lambda and Recursive functions, Generators, and JSON data parsing.
In this lesson we will study about Lambda Functions also known as Anonymous Functions. These are the functions with no names. These are also called as Lambda Expression.
Then we will see the sorting of different data types and will see how we can use the lambda function as key of sorting. We will see multi-level sorting and parallel sorting and will use the concepts on complex dataset.
Link of Movies Task file:
Link of Student Data Task file:
The csv file used in Student Task:
Complete Playlist:
If you have the basic programming knowledge and interested to learn Object-Oriented Programming in Python, check out this playlist:
Lab Manual 29 can be downloaded from here:
Review Question:
1- At 10:10 we saw that we cannot sort a nested list having inner list and tuples together. Now use the argument key efficiently so that inner lists and tuples are sorted on the basis of first element.
2- We have a list of XY points as shown here: p=[(2,1),(3,1),(1,5),(1,0),(4,2)]
Sort the list of points based on their distance from point (5,5)
3- Do review question given at 32:02
4- Here is a list of few numbers: [2,5,-1,4,-2,1,0]
First generate a sorted list based on the magnitude of the number.
Then generate another sorted list in reverse order (from original list) based on magnitude, and if the magnitude is same then positive number should come before negative number.
#PythonProgramming #python #pythontutorial
In this lesson we will study about Lambda Functions also known as Anonymous Functions. These are the functions with no names. These are also called as Lambda Expression.
Then we will see the sorting of different data types and will see how we can use the lambda function as key of sorting. We will see multi-level sorting and parallel sorting and will use the concepts on complex dataset.
Link of Movies Task file:
Link of Student Data Task file:
The csv file used in Student Task:
Complete Playlist:
If you have the basic programming knowledge and interested to learn Object-Oriented Programming in Python, check out this playlist:
Lab Manual 29 can be downloaded from here:
Review Question:
1- At 10:10 we saw that we cannot sort a nested list having inner list and tuples together. Now use the argument key efficiently so that inner lists and tuples are sorted on the basis of first element.
2- We have a list of XY points as shown here: p=[(2,1),(3,1),(1,5),(1,0),(4,2)]
Sort the list of points based on their distance from point (5,5)
3- Do review question given at 32:02
4- Here is a list of few numbers: [2,5,-1,4,-2,1,0]
First generate a sorted list based on the magnitude of the number.
Then generate another sorted list in reverse order (from original list) based on magnitude, and if the magnitude is same then positive number should come before negative number.
#PythonProgramming #python #pythontutorial
Комментарии