filmov
tv
Mastering Custom Event Listeners in JavaScript
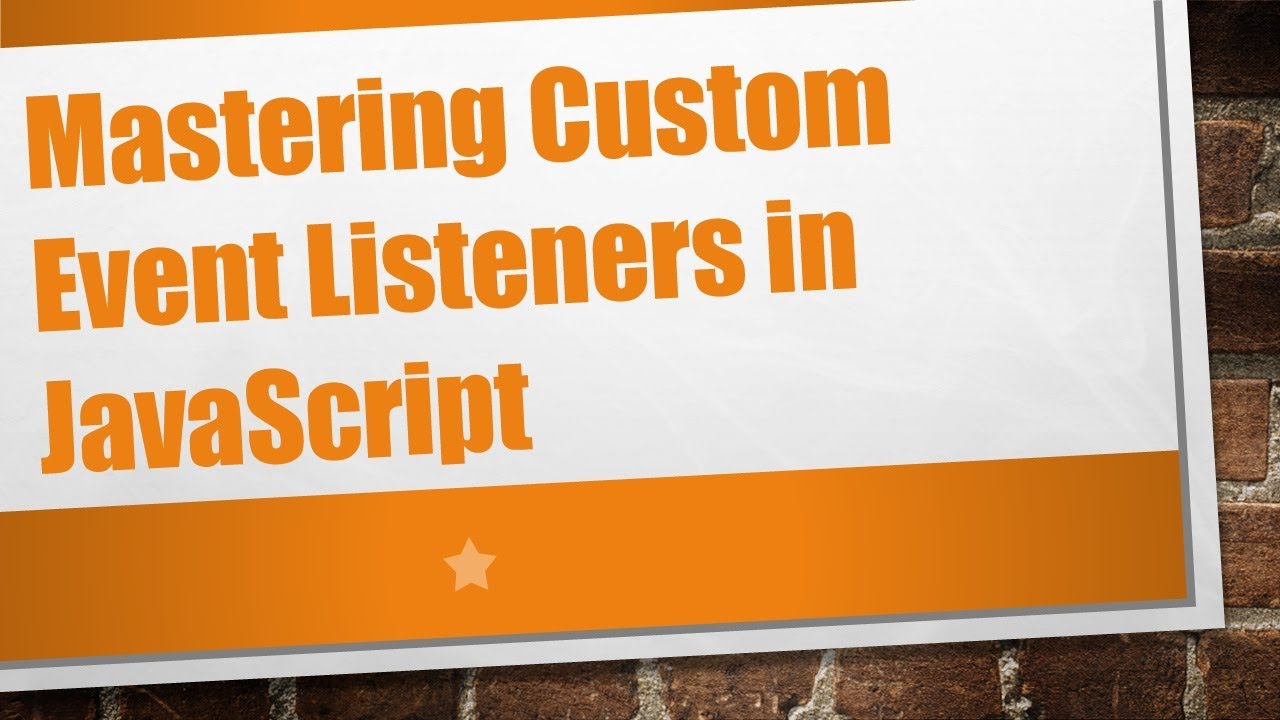
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Learn how to effectively use custom event listeners in JavaScript, including adding custom event handlers and practical examples for seamless integration in your projects.
---
Mastering Custom Event Listeners in JavaScript
Event handling is a crucial part of modern web development, and JavaScript comes with robust built-in capabilities to manage events. However, there are scenarios where standard events might not suffice, and custom event listeners come into play. This guide will walk you through understanding custom event listeners, adding custom event handlers, and provide practical examples to solidify the concepts.
What is a Custom Event Listener in JavaScript?
A custom event listener is an event listener that developers define to handle user-defined or custom events, rather than pre-defined ones such as 'click' or 'hover'. It allows you to create more modular and manageable code by encapsulating specific behaviors that respond to unique conditions or interactions in your application.
Adding Custom Event Handlers
To utilize custom event listeners effectively, you need to know how to define and add custom event handlers. Here is a step-by-step approach:
Create the Custom Event
To create a custom event, you use the CustomEvent constructor. This involves defining an event name and optionally passing some data.
[[See Video to Reveal this Text or Code Snippet]]
Dispatch the Custom Event
An event must be dispatched to be recognized by any event listeners. You can dispatch custom events using the dispatchEvent method on the target element.
[[See Video to Reveal this Text or Code Snippet]]
Add the Custom Event Listener
Finally, add an event listener to handle the dispatched event. This can be done using addEventListener.
[[See Video to Reveal this Text or Code Snippet]]
JavaScript Custom Event Listener Example
Let's put everything together in a complete example:
[[See Video to Reveal this Text or Code Snippet]]
In this example:
A button is created in the HTML to trigger the custom event.
A custom event 'myCustomEvent' is created with some detailed data.
An event listener for 'myCustomEvent' is added to log the custom event details.
On button click, the custom event is dispatched, showing how a custom event can be triggered.
Conclusion
Custom event listeners in JavaScript are a powerful way to create more maintainable and modular code. They allow you to define specific behaviors that better match your application's needs. By understanding how to create, dispatch, and handle custom events, you can enhance the interactivity and functionality of your web applications. Experiment with custom events in your projects to see how they can simplify your event management logic.
---
Summary: Learn how to effectively use custom event listeners in JavaScript, including adding custom event handlers and practical examples for seamless integration in your projects.
---
Mastering Custom Event Listeners in JavaScript
Event handling is a crucial part of modern web development, and JavaScript comes with robust built-in capabilities to manage events. However, there are scenarios where standard events might not suffice, and custom event listeners come into play. This guide will walk you through understanding custom event listeners, adding custom event handlers, and provide practical examples to solidify the concepts.
What is a Custom Event Listener in JavaScript?
A custom event listener is an event listener that developers define to handle user-defined or custom events, rather than pre-defined ones such as 'click' or 'hover'. It allows you to create more modular and manageable code by encapsulating specific behaviors that respond to unique conditions or interactions in your application.
Adding Custom Event Handlers
To utilize custom event listeners effectively, you need to know how to define and add custom event handlers. Here is a step-by-step approach:
Create the Custom Event
To create a custom event, you use the CustomEvent constructor. This involves defining an event name and optionally passing some data.
[[See Video to Reveal this Text or Code Snippet]]
Dispatch the Custom Event
An event must be dispatched to be recognized by any event listeners. You can dispatch custom events using the dispatchEvent method on the target element.
[[See Video to Reveal this Text or Code Snippet]]
Add the Custom Event Listener
Finally, add an event listener to handle the dispatched event. This can be done using addEventListener.
[[See Video to Reveal this Text or Code Snippet]]
JavaScript Custom Event Listener Example
Let's put everything together in a complete example:
[[See Video to Reveal this Text or Code Snippet]]
In this example:
A button is created in the HTML to trigger the custom event.
A custom event 'myCustomEvent' is created with some detailed data.
An event listener for 'myCustomEvent' is added to log the custom event details.
On button click, the custom event is dispatched, showing how a custom event can be triggered.
Conclusion
Custom event listeners in JavaScript are a powerful way to create more maintainable and modular code. They allow you to define specific behaviors that better match your application's needs. By understanding how to create, dispatch, and handle custom events, you can enhance the interactivity and functionality of your web applications. Experiment with custom events in your projects to see how they can simplify your event management logic.