filmov
tv
Understanding Lifetimes in Rust
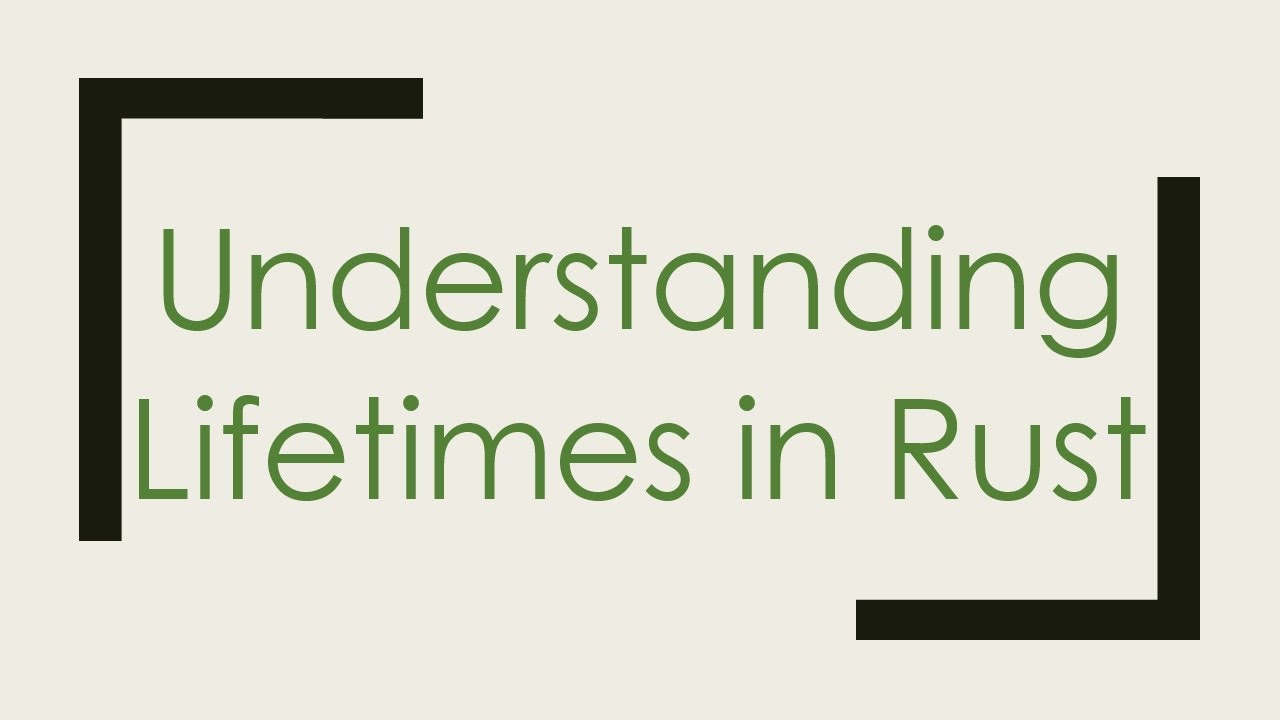
Показать описание
Summary: Dive deep into Rust's lifetimes, understanding their importance in memory safety and how they work to prevent common programming errors.
---
Understanding Lifetimes in Rust: A Comprehensive Guide
Rust is renowned for its memory safety guarantees without a garbage collector. One of the core concepts that enable this feature is the notion of lifetimes. In this guide, we'll delve into what lifetimes are, why they are crucial, and how you can use them effectively in Rust programming.
What Are Lifetimes?
Lifetimes in Rust are a way of describing the scope during which a reference is valid. They are a vital part of Rust's borrowing system, ensuring references do not outlive the data they point to, thereby preventing dangling references, which are a common cause of bugs and security issues in other systems programming languages.
The Importance of Lifetimes
The primary role of lifetimes in Rust is to prevent dangling references. When a reference is used beyond the lifetime of the data it references, it can lead to unpredictable behavior and memory safety violations. Rust's compiler uses lifetime annotations to verify at compile time that all references are valid.
Lifetime Annotations
In many cases, Rust's borrow checker can infer lifetimes on its own. However, there are instances where we need to explicitly annotate them. Lifetime annotations look like regular parameters, except they start with an apostrophe ('). For instance:
[[See Video to Reveal this Text or Code Snippet]]
In the example above, each reference (both input and output) has a lifetime parameter 'a, ensuring the output reference lives at least as long as both input references.
Function Lifetimes
When working with function signatures, lifetime annotations become essential for defining the relationships between the lifetimes of the inputs and the outputs. Without these annotations, the compiler would not be able to determine if such relationships uphold Rust's borrowing rules.
Example:
[[See Video to Reveal this Text or Code Snippet]]
Here, the function foo takes a string slice with lifetime 'a and a number y, then returns a slice that is guaranteed to have the same lifetime 'a.
Structs with Lifetimes
Lifetimes can also be applied to structs. This is useful when structs need to hold references rather than owning the data. Here’s an example:
[[See Video to Reveal this Text or Code Snippet]]
This ImportantExcerpt struct holds a string slice with a specific lifetime 'a.
Lifetimes in Method Definitions
When defining methods for a struct that has lifetime parameters, the same lifetimes must be used in the method signatures.
Example:
[[See Video to Reveal this Text or Code Snippet]]
In this block, methods associated with ImportantExcerpt use the lifetime parameter 'a.
Lifetime Elision
Rust provides a set of lifetime elision rules, where the compiler can infer lifetimes in certain cases, reducing the need for explicit annotations. For example, the following:
[[See Video to Reveal this Text or Code Snippet]]
is automatically understood by the compiler as having the same lifetime for input and output:
[[See Video to Reveal this Text or Code Snippet]]
These elision rules make common patterns simpler and less verbose.
Conclusion
Understanding and correctly using lifetimes in Rust is a crucial skill for ensuring memory safety and optimizing performance. By applying lifetime annotations thoughtfully, you can prevent common pitfalls like dangling references and ensure your programs are both safe and efficient. While lifetimes can initially appear complex, with practice, they become a powerful tool in the Rust developer's arsenal.
We hope this guide has helped demystify lifetimes. With time and practice, using lifetimes effectively will become second nature, making your Rust programs robust and reliable.
---
Understanding Lifetimes in Rust: A Comprehensive Guide
Rust is renowned for its memory safety guarantees without a garbage collector. One of the core concepts that enable this feature is the notion of lifetimes. In this guide, we'll delve into what lifetimes are, why they are crucial, and how you can use them effectively in Rust programming.
What Are Lifetimes?
Lifetimes in Rust are a way of describing the scope during which a reference is valid. They are a vital part of Rust's borrowing system, ensuring references do not outlive the data they point to, thereby preventing dangling references, which are a common cause of bugs and security issues in other systems programming languages.
The Importance of Lifetimes
The primary role of lifetimes in Rust is to prevent dangling references. When a reference is used beyond the lifetime of the data it references, it can lead to unpredictable behavior and memory safety violations. Rust's compiler uses lifetime annotations to verify at compile time that all references are valid.
Lifetime Annotations
In many cases, Rust's borrow checker can infer lifetimes on its own. However, there are instances where we need to explicitly annotate them. Lifetime annotations look like regular parameters, except they start with an apostrophe ('). For instance:
[[See Video to Reveal this Text or Code Snippet]]
In the example above, each reference (both input and output) has a lifetime parameter 'a, ensuring the output reference lives at least as long as both input references.
Function Lifetimes
When working with function signatures, lifetime annotations become essential for defining the relationships between the lifetimes of the inputs and the outputs. Without these annotations, the compiler would not be able to determine if such relationships uphold Rust's borrowing rules.
Example:
[[See Video to Reveal this Text or Code Snippet]]
Here, the function foo takes a string slice with lifetime 'a and a number y, then returns a slice that is guaranteed to have the same lifetime 'a.
Structs with Lifetimes
Lifetimes can also be applied to structs. This is useful when structs need to hold references rather than owning the data. Here’s an example:
[[See Video to Reveal this Text or Code Snippet]]
This ImportantExcerpt struct holds a string slice with a specific lifetime 'a.
Lifetimes in Method Definitions
When defining methods for a struct that has lifetime parameters, the same lifetimes must be used in the method signatures.
Example:
[[See Video to Reveal this Text or Code Snippet]]
In this block, methods associated with ImportantExcerpt use the lifetime parameter 'a.
Lifetime Elision
Rust provides a set of lifetime elision rules, where the compiler can infer lifetimes in certain cases, reducing the need for explicit annotations. For example, the following:
[[See Video to Reveal this Text or Code Snippet]]
is automatically understood by the compiler as having the same lifetime for input and output:
[[See Video to Reveal this Text or Code Snippet]]
These elision rules make common patterns simpler and less verbose.
Conclusion
Understanding and correctly using lifetimes in Rust is a crucial skill for ensuring memory safety and optimizing performance. By applying lifetime annotations thoughtfully, you can prevent common pitfalls like dangling references and ensure your programs are both safe and efficient. While lifetimes can initially appear complex, with practice, they become a powerful tool in the Rust developer's arsenal.
We hope this guide has helped demystify lifetimes. With time and practice, using lifetimes effectively will become second nature, making your Rust programs robust and reliable.