filmov
tv
Understanding Rust Lifetimes
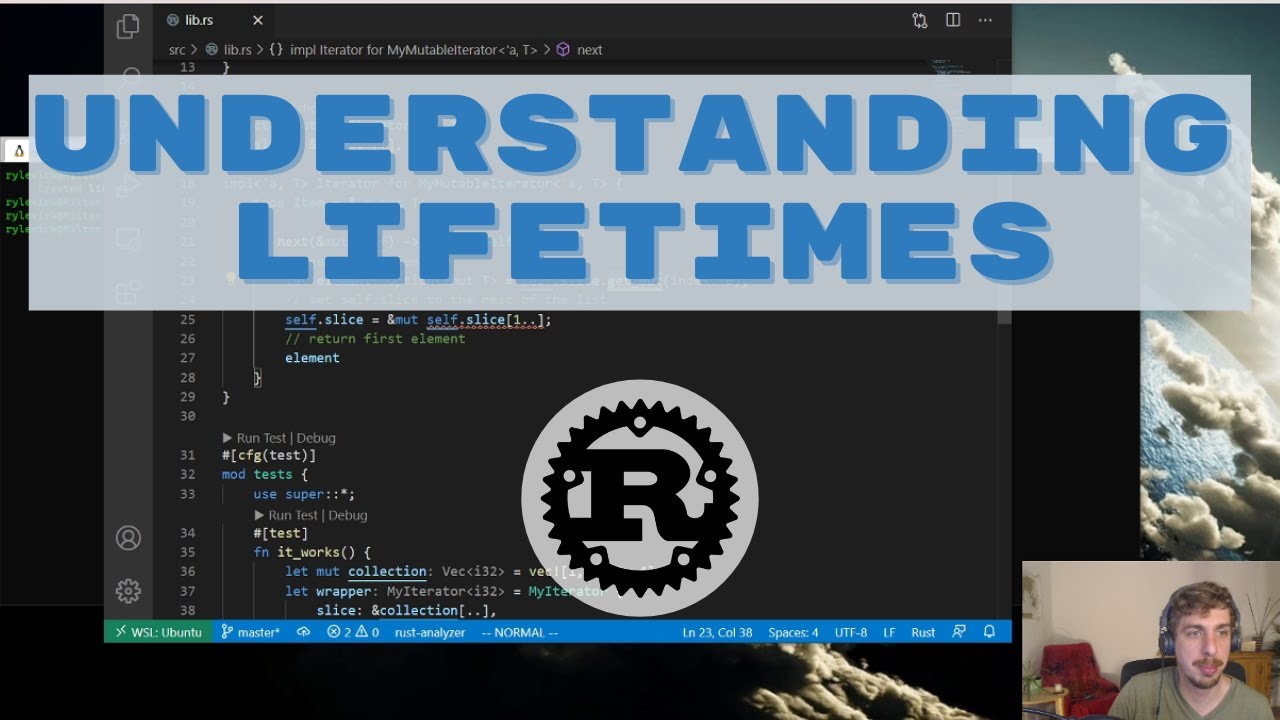
Показать описание
In this stream we attempt to discuss one example that can challenge even long time Rustaceans when it comes to understanding lifetimes. Follow along as we dive deep into lifetimes. If you understand this code, then you're well on your way to understanding almost any Rust related lifetime code out in the wild.
Rust Lifetimes Finally Explained!
Rust's lifetimes made easy
but what is 'a lifetime?
Understanding Rust Lifetimes
Rust Lifetimes explained Tutorial
Rust Demystified 🪄 Simplifying The Toughest Parts
Rust Lifetimes
Crust of Rust: Lifetime Annotations
Rust for TypeScript devs : Borrow Checker
The Rust Survival Guide
Lifetimes in Rust Explained with Examples
Easy Rust 100: Introduction to lifetimes and the 'static lifetime
Demystifying Lifetimes in Rust in 8 Minutes (By A Beginner)
Rust Lifetimes Explained in simple way
Understanding Rust Lifetimes: a visual introduction - Presentation to the Kerala Rustacaeans
Rust: Generics, Traits, Lifetimes
Rust Tutorial - Lifetime Specifiers Explained
Rust's Best Feature - Scope and Lifetimes!
Understanding Lifetimes in Rust
A First Look at Lifetimes in Rust
Why do developers hate Rust?
Rust Lifetimes and How to Use Them
Rust Programming: Lifetimes Demystified - Part 1
Rust lifetimes finally explained
Комментарии