filmov
tv
How to Fix ImportError: cannot import name ... Due to Circular Imports in Python
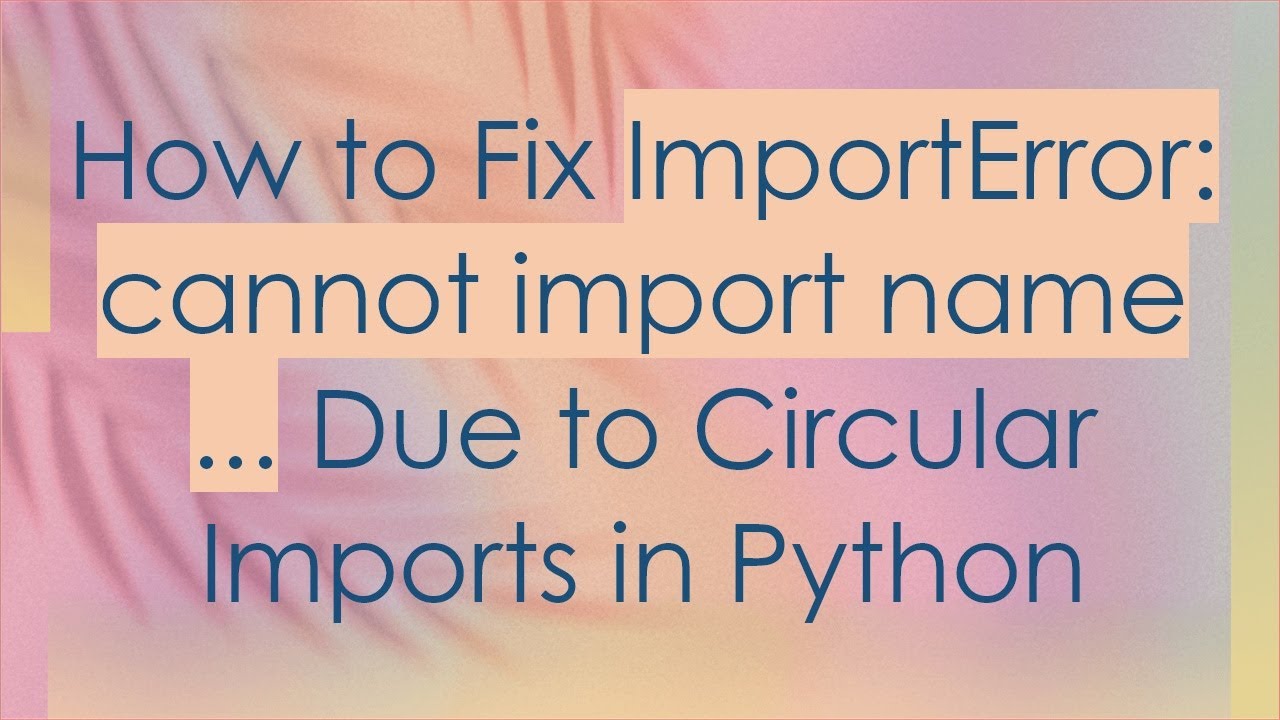
Показать описание
Learn how to tackle the common `ImportError: cannot import name ...` caused by circular imports in Python with practical solutions.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: ImportError: cannot import name ... (most likely due to a circular import)
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding Circular Imports in Python
When working with Python projects, it’s not uncommon to encounter the frustrating ImportError: cannot import name ... (most likely due to a circular import) error. This error usually arises when one module tries to import another that, in turn, imports the first one — creating a loop.
In this guide, we’ll explore the phenomenon of circular imports, using a sample project structure to illustrate the problem, and present effective strategies for resolving these issues.
Problem Overview
Consider a scenario where your project structure resembles the following:
[[See Video to Reveal this Text or Code Snippet]]
For instance, the error message you might see could look something like this:
[[See Video to Reveal this Text or Code Snippet]]
Why Circular Imports Occur
Circular imports happen when two or more modules attempt to import one another. Here’s a simplified explanation of how this can occur:
As a result, Python gets stuck trying to load the modules, leading to an ImportError.
Strategies to Resolve Circular Import Errors
1. Use the typing Library
One common workaround is to use Python’s typing library with the TYPE_CHECKING variable. This allows you to conditionally import modules only when type checking, thus avoiding circular imports at runtime.
Here's how you can implement it:
[[See Video to Reveal this Text or Code Snippet]]
This way, the imports only happen when you are type-checking your code, which can circumvent the circular import issue.
2. Check Module Loading
Another method is to check which modules are already loaded and only attempt to import if they have not been loaded yet. This can help prevent the circular import situation from arising in the first place.
3. Restructure Your Code
Sometimes, the best solution may be to rethink your overall code structure. By refactoring your modules to minimize interdependencies or to create a common module for shared functionalities, you can often avoid circular imports altogether.
Conclusion
In conclusion, encountering ImportError: cannot import name ... (most likely due to a circular import) can be frustrating, but understanding the cause and employing a few strategies can help you resolve the issue. Remember, effective structuring of your modules and careful management of your imports will go a long way in preventing these errors.
By following the outlined methods and maintaining clarity in your project organization, you'll be well-equipped to handle and circumvent circular imports in Python.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: ImportError: cannot import name ... (most likely due to a circular import)
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding Circular Imports in Python
When working with Python projects, it’s not uncommon to encounter the frustrating ImportError: cannot import name ... (most likely due to a circular import) error. This error usually arises when one module tries to import another that, in turn, imports the first one — creating a loop.
In this guide, we’ll explore the phenomenon of circular imports, using a sample project structure to illustrate the problem, and present effective strategies for resolving these issues.
Problem Overview
Consider a scenario where your project structure resembles the following:
[[See Video to Reveal this Text or Code Snippet]]
For instance, the error message you might see could look something like this:
[[See Video to Reveal this Text or Code Snippet]]
Why Circular Imports Occur
Circular imports happen when two or more modules attempt to import one another. Here’s a simplified explanation of how this can occur:
As a result, Python gets stuck trying to load the modules, leading to an ImportError.
Strategies to Resolve Circular Import Errors
1. Use the typing Library
One common workaround is to use Python’s typing library with the TYPE_CHECKING variable. This allows you to conditionally import modules only when type checking, thus avoiding circular imports at runtime.
Here's how you can implement it:
[[See Video to Reveal this Text or Code Snippet]]
This way, the imports only happen when you are type-checking your code, which can circumvent the circular import issue.
2. Check Module Loading
Another method is to check which modules are already loaded and only attempt to import if they have not been loaded yet. This can help prevent the circular import situation from arising in the first place.
3. Restructure Your Code
Sometimes, the best solution may be to rethink your overall code structure. By refactoring your modules to minimize interdependencies or to create a common module for shared functionalities, you can often avoid circular imports altogether.
Conclusion
In conclusion, encountering ImportError: cannot import name ... (most likely due to a circular import) can be frustrating, but understanding the cause and employing a few strategies can help you resolve the issue. Remember, effective structuring of your modules and careful management of your imports will go a long way in preventing these errors.
By following the outlined methods and maintaining clarity in your project organization, you'll be well-equipped to handle and circumvent circular imports in Python.