filmov
tv
Prim's Algorithm - Minimum Spanning Tree - Min Cost to Connect all Points - Leetcode 1584 - Python
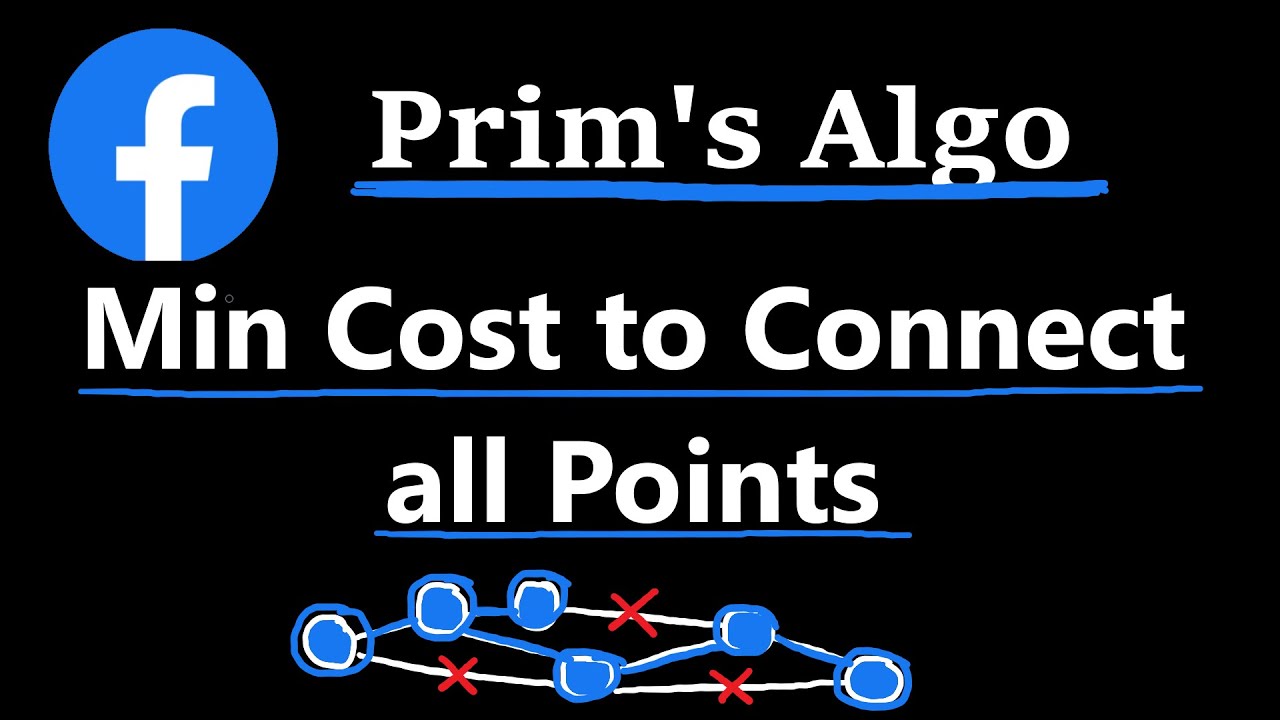
Показать описание
0:00 - Read the problem
3:44 - Drawing Explanation
16:32 - Coding Explanation
leetcode 1584
#prims #algorithm #python
Disclosure: Some of the links above may be affiliate links, from which I may earn a small commission.
3.5 Prims and Kruskals Algorithms - Greedy Method
Prim's Algorithm
Prim's Algorithm - Minimum Spanning Tree - Min Cost to Connect all Points - Leetcode 1584 - Pyt...
L-4.9: Prim's Algorithm for Minimum Cost Spanning Tree | Prims vs Kruskal
Prim's Minimum Spanning Tree Algorithm | Graph Theory
G-45. Prim's Algorithm - Minimum Spanning Tree - C++ and Java
Prim's algorithm | Minimum Spanning tree (MST) | Design & Algorithms | Lec-26 | Bhanu Priya
Proof of Prim's MST algorithm using cut property
PRIM'S ALGORITHM (MINIMUM SPANNING TREE) || GRAPH
Prim's Algorithm: Minimal Spanning Tree
Minimum spanning tree || prims algorithm in data structure || 63 ||Data structures in telugu
Prim's Algorithm | Minimum Spanning Tree | Full Dry Run | INTUITION | Graph Concepts & Qns ...
12. Greedy Algorithms: Minimum Spanning Tree
Networks: Prim's Algorithm for finding Minimum spanning trees
Kruskal's Algorithm
Prim's Algorithm for Minimum Cost Spanning Tree
Prim's Algorithm for minimum spanning trees || GATECSE || DAA
Prim's Algorithm for Minimum Spanning Trees (MST) | Graph Theory
OCR MEI MwA E: Minimum Spanning Trees: 06 Prim’s Algorithm with a Matrix Example 1
Prims Algorithm to Find Minimum Spanning Tree of a Graph | Algorithm with Pseudo Code | Logic First
#2 Prims Algorithm Minimum | How to find Spanning Tree Greedy Search Algorithm by Dr. Mahesh Huddar
Lecture 96: Minimum Spanning Tree || Prim's Algorithm
How does Prim's Algorithm work? #shorts
Prim's Algorithm Part-1 For Minimum Spanning Tree Explained With Solved Example in Hindi
Комментарии