filmov
tv
How to Flatten a List of Objects in Python
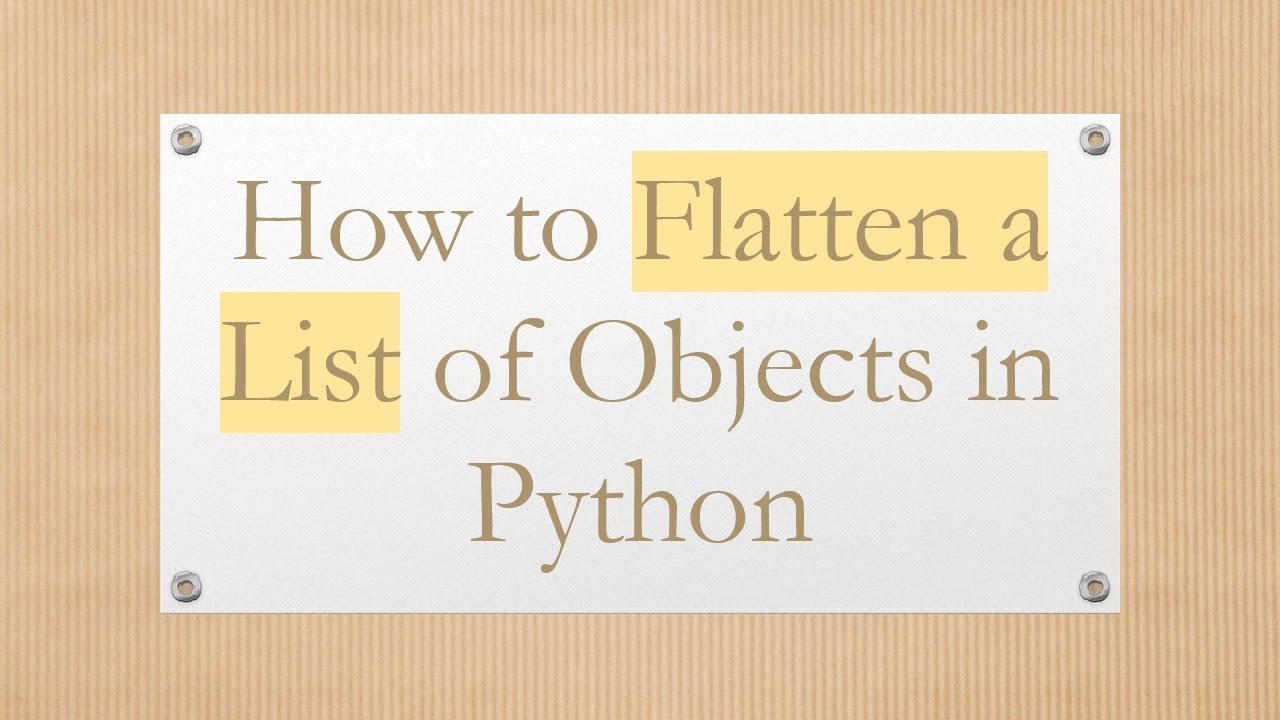
Показать описание
Discover how to easily flatten complex nested lists of objects in Python using a recursive function. Simplify your data structure with our step-by-step instructions!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to flatten a list of objects in python
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Flatten a List of Objects in Python: A Step-by-Step Guide
Dealing with complex data structures in Python can be challenging, especially when it comes to nested lists of objects. Flattening these structures into a simpler form is often necessary for easier data manipulation and processing. If you have been wondering how to flatten a list of objects in Python, this guide is just for you!
Understanding the Problem
Imagine you have a list of dictionaries, each representing a person and their associated details, including nested data. The following is a snippet of such a structure:
[[See Video to Reveal this Text or Code Snippet]]
Your goal is to transform this structure into a flat list, preserving only the relevant fields. For example, the desired output is:
[[See Video to Reveal this Text or Code Snippet]]
The Solution: Using a Recursive Function
To flatten a list of objects, we can use a recursive function. This function will navigate through each object, extract the relevant keys, and handle any nested lists until all data is neatly collected in a flat structure.
Step-by-Step Implementation
Function Definition
Define a function that takes two parameters: a list of objects and a list to accumulate the results.
Convert Single Dict to List
To ensure consistency in handling the input, convert a single dictionary to a list.
Flattening Process
Loop through each object and create a new dictionary with the keys we want to retain. Append this to the flattened list.
Recursion for Nested Lists
Check if the current object contains any lists and call the function recursively on those lists.
Here is how the code looks:
[[See Video to Reveal this Text or Code Snippet]]
Output
After running this code, you will receive an output similar to the following, demonstrating a successful flattening of the nested structure:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Flattening a nested list of objects in Python can simplify data management, making it easier to analyze or export information. By utilizing a recursive function, we navigate through complex structures efficiently. Happy coding, and feel free to modify the function to suit your specific needs!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to flatten a list of objects in python
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Flatten a List of Objects in Python: A Step-by-Step Guide
Dealing with complex data structures in Python can be challenging, especially when it comes to nested lists of objects. Flattening these structures into a simpler form is often necessary for easier data manipulation and processing. If you have been wondering how to flatten a list of objects in Python, this guide is just for you!
Understanding the Problem
Imagine you have a list of dictionaries, each representing a person and their associated details, including nested data. The following is a snippet of such a structure:
[[See Video to Reveal this Text or Code Snippet]]
Your goal is to transform this structure into a flat list, preserving only the relevant fields. For example, the desired output is:
[[See Video to Reveal this Text or Code Snippet]]
The Solution: Using a Recursive Function
To flatten a list of objects, we can use a recursive function. This function will navigate through each object, extract the relevant keys, and handle any nested lists until all data is neatly collected in a flat structure.
Step-by-Step Implementation
Function Definition
Define a function that takes two parameters: a list of objects and a list to accumulate the results.
Convert Single Dict to List
To ensure consistency in handling the input, convert a single dictionary to a list.
Flattening Process
Loop through each object and create a new dictionary with the keys we want to retain. Append this to the flattened list.
Recursion for Nested Lists
Check if the current object contains any lists and call the function recursively on those lists.
Here is how the code looks:
[[See Video to Reveal this Text or Code Snippet]]
Output
After running this code, you will receive an output similar to the following, demonstrating a successful flattening of the nested structure:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Flattening a nested list of objects in Python can simplify data management, making it easier to analyze or export information. By utilizing a recursive function, we navigate through complex structures efficiently. Happy coding, and feel free to modify the function to suit your specific needs!