filmov
tv
How to Flatten a List of Lists in Python
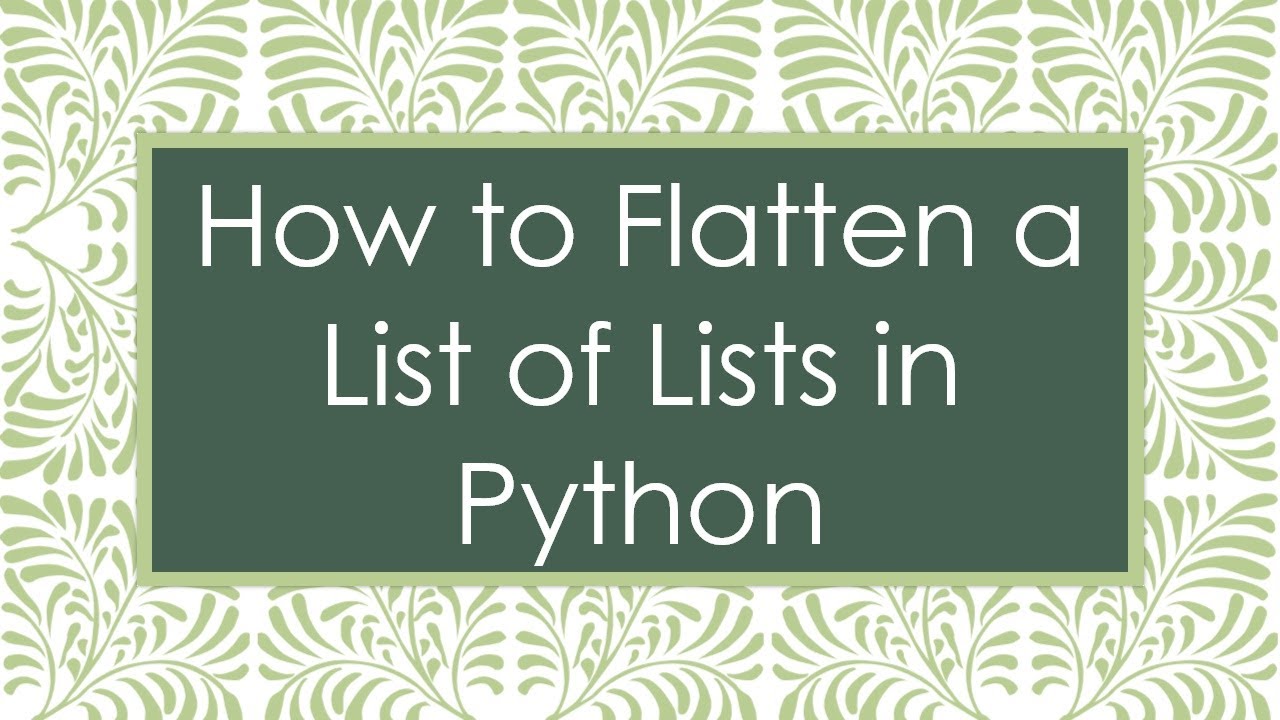
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Learn how to flatten a list of lists into a single flat list in Python using different methods and techniques. Flatten lists effortlessly and efficiently in Python programming.
---
Flattening a list of lists in Python is a common task, especially when dealing with nested data structures. Fortunately, Python offers several ways to achieve this efficiently. Let's explore a few methods:
Using List Comprehension
One of the most concise ways to flatten a list of lists is by using list comprehension:
[[See Video to Reveal this Text or Code Snippet]]
In this code snippet, item represents each individual element in the nested lists, while sublist represents each sublist in the list of lists. The list comprehension iterates over each sublist and appends its elements to the flat_list.
[[See Video to Reveal this Text or Code Snippet]]
Using Nested Loops
A straightforward approach involves using nested loops to iterate through the nested lists:
[[See Video to Reveal this Text or Code Snippet]]
This method iterates through each sublist and each element within the sublists, appending them to the flat_list.
Using Recursive Function
Another approach is to define a recursive function to flatten the list of lists:
[[See Video to Reveal this Text or Code Snippet]]
The flatten_list() function recursively traverses through nested lists, appending individual elements to the flat_list.
Choose the method that best fits your requirements based on readability, performance, and personal preference.
Flattening lists is a fundamental operation in Python programming, and understanding different techniques for achieving it can enhance your coding efficiency and productivity.
---
Summary: Learn how to flatten a list of lists into a single flat list in Python using different methods and techniques. Flatten lists effortlessly and efficiently in Python programming.
---
Flattening a list of lists in Python is a common task, especially when dealing with nested data structures. Fortunately, Python offers several ways to achieve this efficiently. Let's explore a few methods:
Using List Comprehension
One of the most concise ways to flatten a list of lists is by using list comprehension:
[[See Video to Reveal this Text or Code Snippet]]
In this code snippet, item represents each individual element in the nested lists, while sublist represents each sublist in the list of lists. The list comprehension iterates over each sublist and appends its elements to the flat_list.
[[See Video to Reveal this Text or Code Snippet]]
Using Nested Loops
A straightforward approach involves using nested loops to iterate through the nested lists:
[[See Video to Reveal this Text or Code Snippet]]
This method iterates through each sublist and each element within the sublists, appending them to the flat_list.
Using Recursive Function
Another approach is to define a recursive function to flatten the list of lists:
[[See Video to Reveal this Text or Code Snippet]]
The flatten_list() function recursively traverses through nested lists, appending individual elements to the flat_list.
Choose the method that best fits your requirements based on readability, performance, and personal preference.
Flattening lists is a fundamental operation in Python programming, and understanding different techniques for achieving it can enhance your coding efficiency and productivity.