filmov
tv
How to sort an array in C++ for beginners ➡️
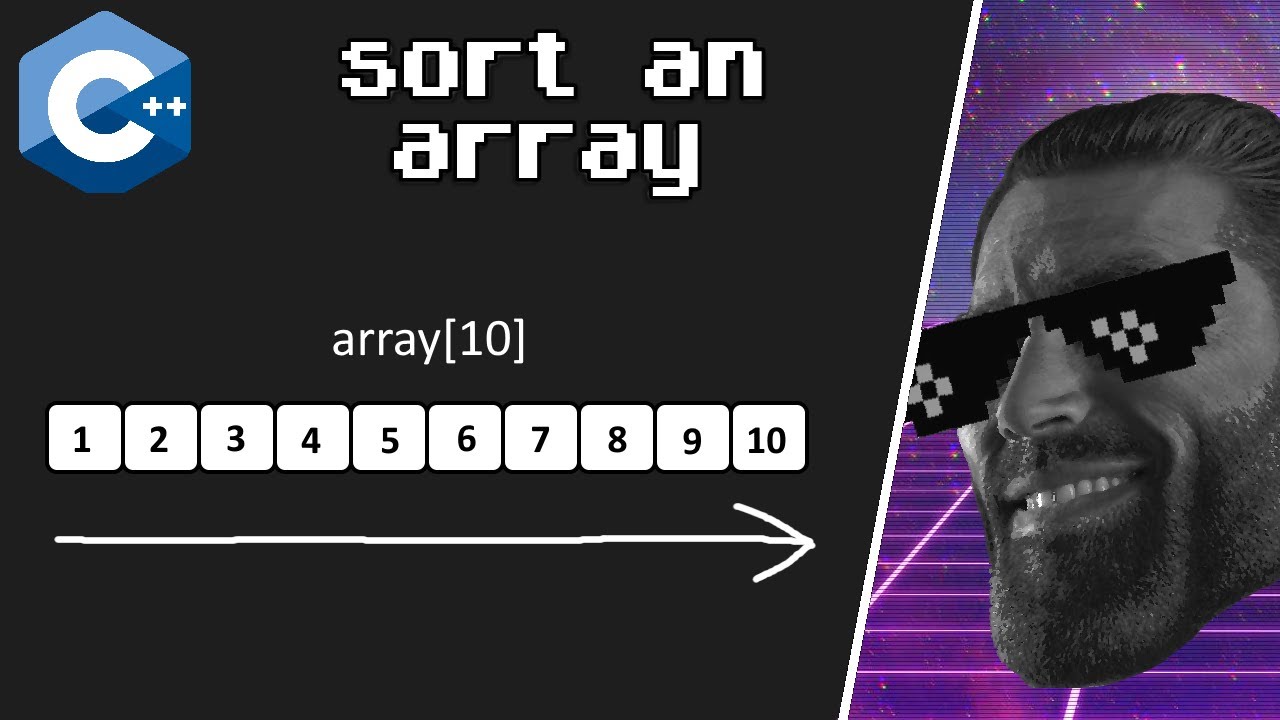
Показать описание
#array #sort
How to sort an array in C++ tutorial example explained
How to sort an array in C++ tutorial example explained
C sort an array 💱
Java Program #17 - Sort an Array of Integers in ascending order
Sort an Array - Leetcode 912 - Python
How to sort an array in C++ for beginners ➡️
How to sort an array using sort() – Swift 5
Algorithms: Sort An Array with Comparator
16.9: Array Functions: sort() - Topics of JavaScript/ES6
sort Array Method | JavaScript Tutorial
#33 How to Sort an Array | Sorting an Array | JavaScript Bangla Tutorial
Sort an Array | Leetcode 912 | codestorywithMIK
8.3.1 Sorting in Arrays | Selection Sort | C++ Placement Course
Learn JavaScript SORTING in 6 minutes! 🗃
Sorting Algorithms Explained Visually
Learn Bubble Sort in 7 minutes 🤿
C40 - Program to arrange numbers in ascending order | sort values in array
Sort an array of 0's 1's & 2's | Intuition of Algo🔥 | C++ Java Python | Brute-Bet...
Sort an Array which contain 1 to N values in O(N) Time Complexity !
Sorting in Array Data Structure- How to Sort an Array in C++ - Ascending Order - Descending Order
JavaScript Problem: Sorting an Array of Objects
Using qsort() To Sort An Array | C Programming Example
10 Sorting Algorithms Easily Explained
Frequently Asked Java Program 22: Sort Elements in Array | Bubble Sort
912. Sort an Array | sorting | Leetcode Daily Challenge | DSA | Hindi
How To Sort Array In Ascending & Descending Order With Javascript
Комментарии