filmov
tv
Reverse a Sentence using Stacks | Stack Challenge | C++ Placement Course | Lecture 23.2
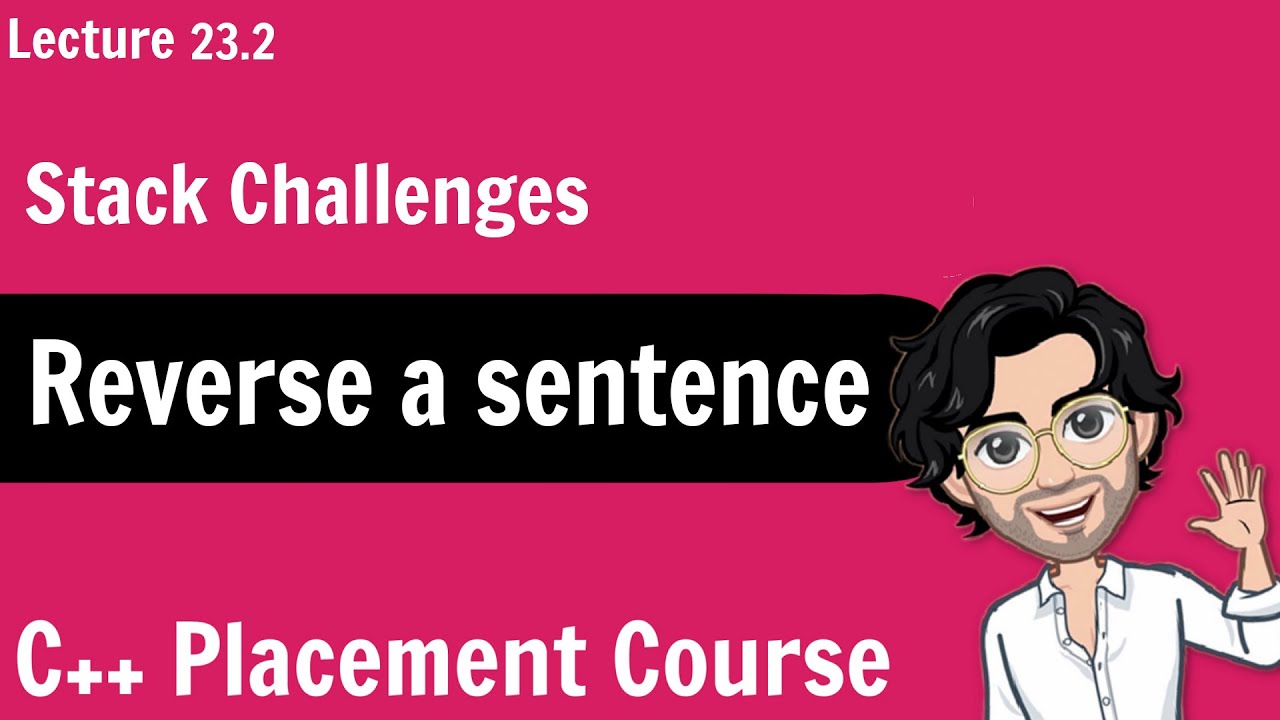
Показать описание
Notes of this Lecture:
Reverse a Sentence using Stacks | Stack Challenge | C++ Placement Course | Lecture 23.2
Stacks (Program 4) – Part 1
Reverse a string or linked list using stack.
Reversing a Word Using Stacks & Queues in Python
Reverse a stack using recursion | Stacks | Love Babbar DSA Sheet | Amazon🔥
Stacks (Program 5)
C_69 C Program to Reverse a String | with strrev() and without strrev() function
Lecture 55: Stack Interview Questions || Placement Series by Love Babbar
17-String Reversal Using Stacks in C# Stack Application
Implement Queue using Stacks - Leetcode 232 - Python
Stacks (Program 4) – Part 2
Stacks (Program 4) – Part 5
Reverse a Single Linked List
2.8 Reverse a Linked List - Iterative Method | Data Structure Tutorials
Basics of Stack | Implementation of Stacks | Reverse a string using Stack | Divyanshu | Geeksforgeek
Check for balanced parentheses using stack
Stacks in Undo and Redo
Stacks (Program 3)
Stack Java Tutorial #65
Infix to Reverse Polish Notation (RPN) using Stacks
Stacks (Program 4) – Part 7
Infix to Postfix using stack
Reverse a Queue using recursion | Stacks & Queues | hindi queue playlist #Princebhai Hello World
Beginner Stacks 2 ways (Tutorial)
Комментарии