filmov
tv
Reverse a string or linked list using stack.
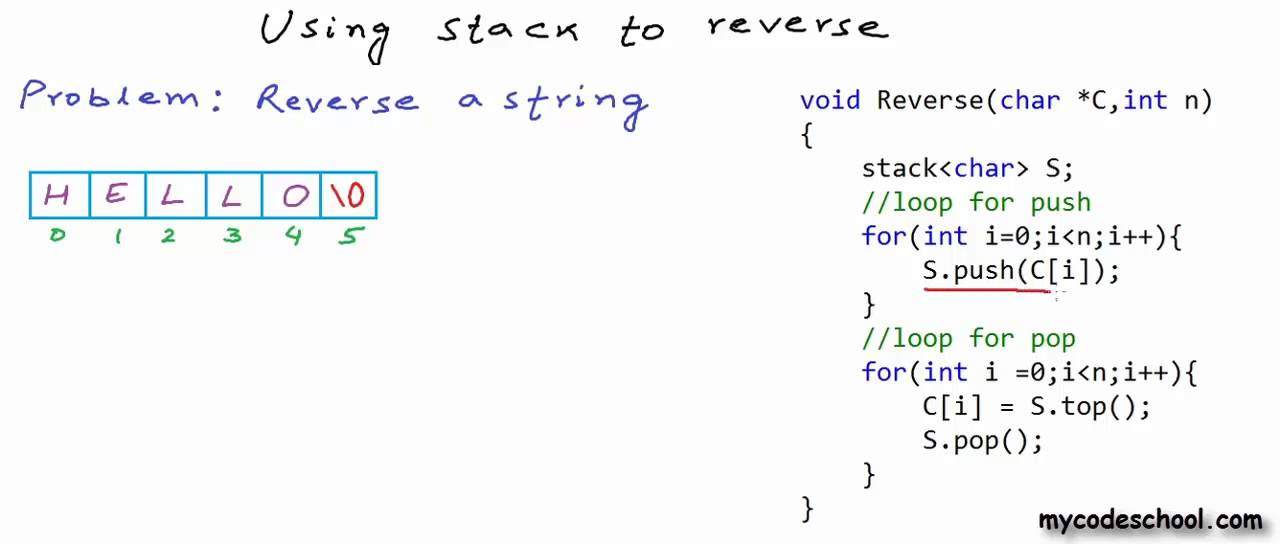
Показать описание
See complete series on data structures here:
In this lesson, we have described how we can reverse a string or linked list using stack. Reversal is a simple application of stack.
To know about implicit stack, see this video:
Reversal of linked list using recursion:
In this lesson, we have described how we can reverse a string or linked list using stack. Reversal is a simple application of stack.
To know about implicit stack, see this video:
Reversal of linked list using recursion:
Reverse a string or linked list using stack.
Reversing a linked list | GeeksforGeeks
Reverse a string or linked list using stack
2.8 Reverse a Linked List - Iterative Method | Data Structure Tutorials
Reverse String using linked list in C
Reverse a string or linked list using stack
Reverse each word in a linked list node | GeeksforGeeks
Reverse String - 3 Ways - Leetcode 344 - Python
Stacks (Program 4) – Part 1
Reverse a Singly Linked List in Java | Leetcode #206 | Data Structures & Algorithms
Reverse a linked list - Iterative method
Reverse the Linked List Visualization | Log2Base2 - The Visual Learning Platform
Reverse a LinkedList using Stack
Reverse a linked list recursively in java
HackerRank Reverse A Linked List Solution Explained - Java
2.13 Reverse a Doubly Linked List | Data Structures & Algorithm Tutorials
Reverse a linked list using recursion
Reverse string in javascript || Javascript reverse string || Reverse string || Javascript tutorial
Reverse A String | C Programming Example
Reverse a string in C/C++ | GeeksforGeeks
JavaScript Challenge: Reversing a String
How to reverse a String using a Stack in Java ? | Animation
Reverse a Stack | C++ Placement Course | Lecture 23.3
Reversing a String with Special Characters
Комментарии