filmov
tv
How to Ensure Typescript Properly Detects Overloaded Function Types in Functional Composition
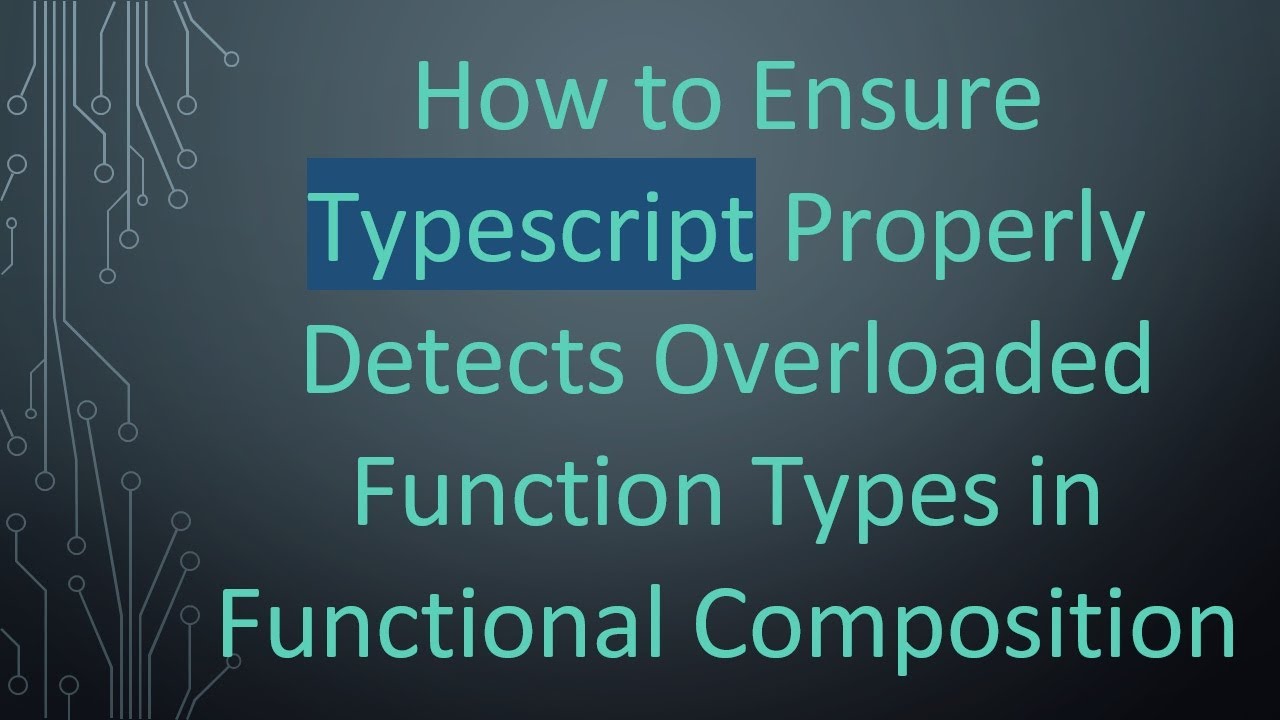
Показать описание
Discover effective strategies to solve `typescript` function overload detection issues in `functional programming` with practical examples.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Is there a way for Typescript to detect correct overloaded function on composition?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Ensure Typescript Properly Detects Overloaded Function Types in Functional Composition
When developing applications in TypeScript, particularly in the realm of functional programming, you may run into a common hurdle: ensuring that TypeScript accurately identifies overloaded function types during composition. For instance, when utilizing overloaded functions, you might expect the compiler to infer parameters correctly. However, it can sometimes opt for a more lenient return type, thus leading to unexpected behaviors and potential errors.
The Problem
Consider the following overloaded function:
[[See Video to Reveal this Text or Code Snippet]]
In this case, you may want to use the asDate function within a higher-order function, like so:
[[See Video to Reveal this Text or Code Snippet]]
You might find that TypeScript infers the return type as Date | null rather than just Date. This happens because TypeScript cannot determine down to the specific overload that applies based on the current context, choosing to return the broader type.
The Solution
Leveraging Function Type Inference
To solve this issue, we want to guide TypeScript so that it can infer the type of the passed function. By fine-tuning our function's type declarations, we can encourage TypeScript to understand the specific overload being used.
Implementing the Changes
Here’s how to modify the apply function:
[[See Video to Reveal this Text or Code Snippet]]
With this change, you allow TypeScript to make an inference based on the type of the function you’re passing in. Now, let’s put everything together:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of Modifications
Type Extension in apply: The change made in apply<F extends (args: any) => any>(fn: F) allows TypeScript to infer the specific overload applied when the function is called.
Revised Overloads for asDate: By properly defining overloads for asDate, you can clarify when it should return Date and when it should return null.
Test Cases: These test cases demonstrate how TypeScript can now correctly infer the return types without confusion.
Conclusion
By enhancing the way we define our function types, TypeScript can more effectively handle overloaded function calls in the context of functional programming. This not only improves type safety within our applications but also enhances developer experience by reducing runtime errors related to type mismatches.
Make sure to implement these strategies in your projects, and enjoy a smoother development experience with TypeScript when dealing with function overloads!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Is there a way for Typescript to detect correct overloaded function on composition?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Ensure Typescript Properly Detects Overloaded Function Types in Functional Composition
When developing applications in TypeScript, particularly in the realm of functional programming, you may run into a common hurdle: ensuring that TypeScript accurately identifies overloaded function types during composition. For instance, when utilizing overloaded functions, you might expect the compiler to infer parameters correctly. However, it can sometimes opt for a more lenient return type, thus leading to unexpected behaviors and potential errors.
The Problem
Consider the following overloaded function:
[[See Video to Reveal this Text or Code Snippet]]
In this case, you may want to use the asDate function within a higher-order function, like so:
[[See Video to Reveal this Text or Code Snippet]]
You might find that TypeScript infers the return type as Date | null rather than just Date. This happens because TypeScript cannot determine down to the specific overload that applies based on the current context, choosing to return the broader type.
The Solution
Leveraging Function Type Inference
To solve this issue, we want to guide TypeScript so that it can infer the type of the passed function. By fine-tuning our function's type declarations, we can encourage TypeScript to understand the specific overload being used.
Implementing the Changes
Here’s how to modify the apply function:
[[See Video to Reveal this Text or Code Snippet]]
With this change, you allow TypeScript to make an inference based on the type of the function you’re passing in. Now, let’s put everything together:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of Modifications
Type Extension in apply: The change made in apply<F extends (args: any) => any>(fn: F) allows TypeScript to infer the specific overload applied when the function is called.
Revised Overloads for asDate: By properly defining overloads for asDate, you can clarify when it should return Date and when it should return null.
Test Cases: These test cases demonstrate how TypeScript can now correctly infer the return types without confusion.
Conclusion
By enhancing the way we define our function types, TypeScript can more effectively handle overloaded function calls in the context of functional programming. This not only improves type safety within our applications but also enhances developer experience by reducing runtime errors related to type mismatches.
Make sure to implement these strategies in your projects, and enjoy a smoother development experience with TypeScript when dealing with function overloads!