filmov
tv
How to Properly Map a List to JSON Objects in TypeScript
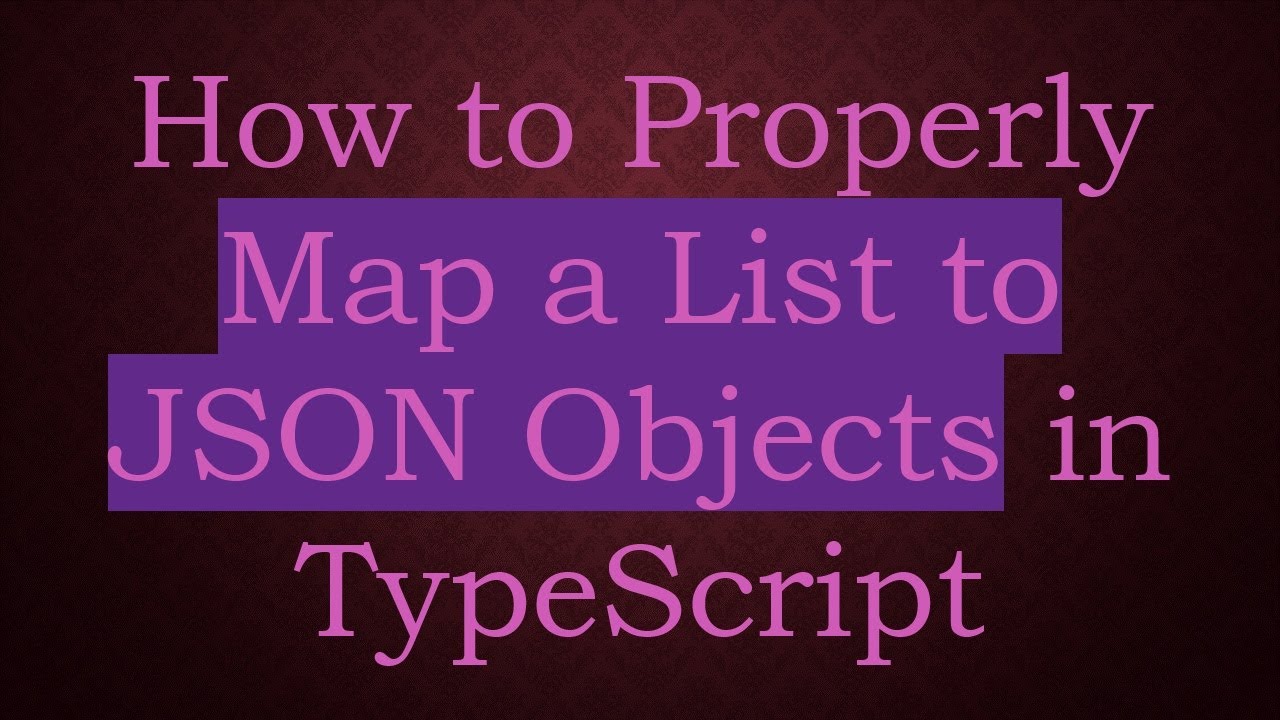
Показать описание
Learn how to convert a list of strings into a list of JSON objects in TypeScript without syntax errors. This guide includes simple explanations and code examples.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How can I map a list to a list of JSON?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mapping a List to JSON Objects in TypeScript: A Simple Guide
In programming, transforming data from one format to another is a common task. One such scenario involves mapping a list of strings to a list of JSON objects in TypeScript. This task can sometimes lead to syntax errors if the code is not written properly. In this guide, we'll start by exploring the problem, followed by a step-by-step explanation of how to achieve this transformation effectively.
The Problem
Suppose you have a list of strings, such as ["a", "b", "c"], and you want to convert it into a list of JSON objects like [{"str":"a"},{"str":"b"},{"str":"c"}]. This is a straightforward task but is often accompanied by syntax errors, especially for those new to TypeScript. A common attempt to do this might look like this:
[[See Video to Reveal this Text or Code Snippet]]
However, when you try running this code, you encounter an error message stating: error TS1005: ';' expected. This can be a frustrating experience, but let's break down the solution clearly.
Analyzing the Error
The error arises because the TypeScript compiler misinterprets the curly braces {} in the mapping function. In JavaScript and TypeScript, curly braces can denote either a block of code (function body) or an object. However, in your case, you want to create an object. To resolve the error, you need to clarify your intention to the compiler.
The Solution: Mapping Correctly
To properly map the list of strings to JSON objects, you need to ensure the syntax indicates you’re creating an object. Here's how to do this correctly:
Correct Mapping Syntax
Instead of using curly braces directly like this:
[[See Video to Reveal this Text or Code Snippet]]
You should wrap the object in parentheses like this:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Solution
Using Parentheses: The key fix here is the parentheses surrounding the object literal. By adding parentheses, you signal to the TypeScript compiler that you want to return an object and not create a function body.
Type Definition: Defining foo with the type ({ str: string })[] ensures that TypeScript understands that you're working with an array of objects which have a property str of type string.
Example in Action
Here's the complete example to clarify how it works:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Transforming a list of strings into a list of JSON objects in TypeScript can be done easily once you understand the correct syntax. By wrapping your object literal in parentheses, you avoid common syntax errors and clearly communicate your intentions to the compiler. The method described above is not only valid TypeScript but also aligns well with JavaScript syntax, making it versatile for your coding needs.
Feel free to try this mapping technique in your TypeScript projects, and you'll find it much smoother than before. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How can I map a list to a list of JSON?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mapping a List to JSON Objects in TypeScript: A Simple Guide
In programming, transforming data from one format to another is a common task. One such scenario involves mapping a list of strings to a list of JSON objects in TypeScript. This task can sometimes lead to syntax errors if the code is not written properly. In this guide, we'll start by exploring the problem, followed by a step-by-step explanation of how to achieve this transformation effectively.
The Problem
Suppose you have a list of strings, such as ["a", "b", "c"], and you want to convert it into a list of JSON objects like [{"str":"a"},{"str":"b"},{"str":"c"}]. This is a straightforward task but is often accompanied by syntax errors, especially for those new to TypeScript. A common attempt to do this might look like this:
[[See Video to Reveal this Text or Code Snippet]]
However, when you try running this code, you encounter an error message stating: error TS1005: ';' expected. This can be a frustrating experience, but let's break down the solution clearly.
Analyzing the Error
The error arises because the TypeScript compiler misinterprets the curly braces {} in the mapping function. In JavaScript and TypeScript, curly braces can denote either a block of code (function body) or an object. However, in your case, you want to create an object. To resolve the error, you need to clarify your intention to the compiler.
The Solution: Mapping Correctly
To properly map the list of strings to JSON objects, you need to ensure the syntax indicates you’re creating an object. Here's how to do this correctly:
Correct Mapping Syntax
Instead of using curly braces directly like this:
[[See Video to Reveal this Text or Code Snippet]]
You should wrap the object in parentheses like this:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Solution
Using Parentheses: The key fix here is the parentheses surrounding the object literal. By adding parentheses, you signal to the TypeScript compiler that you want to return an object and not create a function body.
Type Definition: Defining foo with the type ({ str: string })[] ensures that TypeScript understands that you're working with an array of objects which have a property str of type string.
Example in Action
Here's the complete example to clarify how it works:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Transforming a list of strings into a list of JSON objects in TypeScript can be done easily once you understand the correct syntax. By wrapping your object literal in parentheses, you avoid common syntax errors and clearly communicate your intentions to the compiler. The method described above is not only valid TypeScript but also aligns well with JavaScript syntax, making it versatile for your coding needs.
Feel free to try this mapping technique in your TypeScript projects, and you'll find it much smoother than before. Happy coding!