filmov
tv
python tutorial for beginners #3: variables and datatype in python
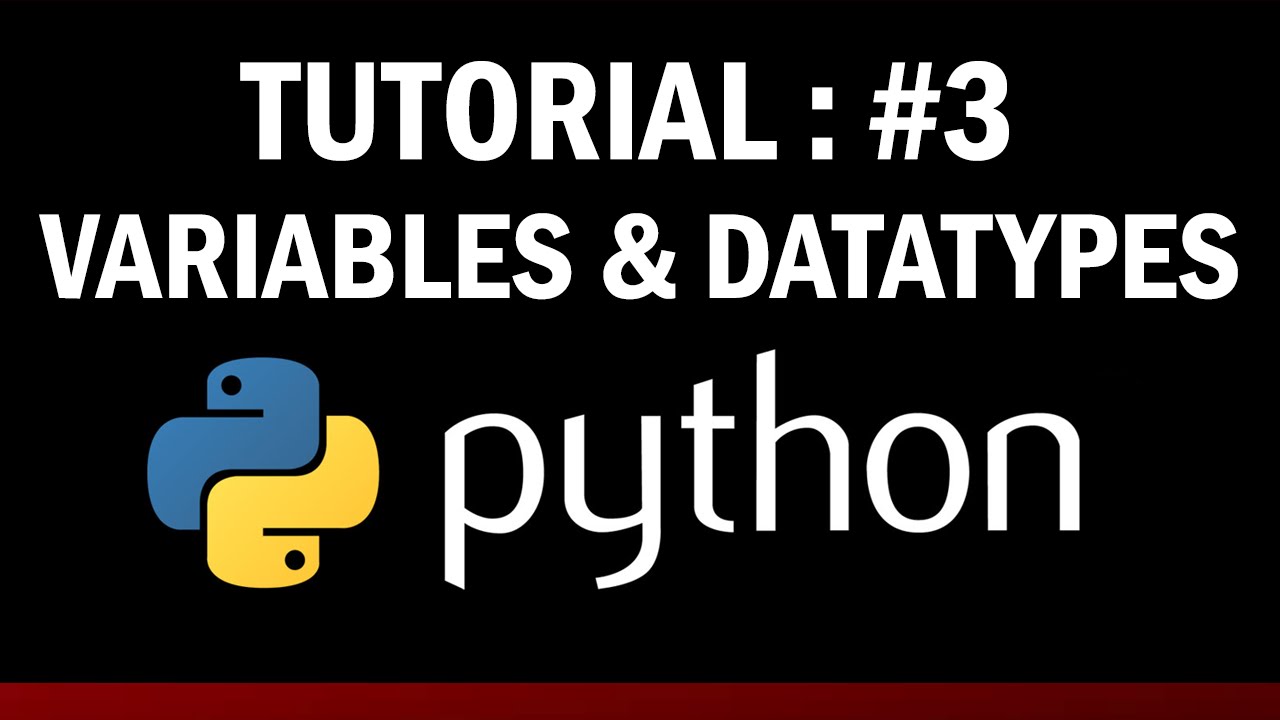
Показать описание
python tutorial for beginners #3: variables and datatype in python
A variable is a name in source code that is associated with an area in memory that you can use to store data, which is then called upon throughout the code.
We create a variable by writing the name of the variable we want followed by an equal’s sign, which is followed by the value we want to store in the variable.
The data can be one of many types, including:
Integer, that Stores whole numbers
hello_int = 21
Float data type: Stores decimal numbers
hello_float = 1.3
Boolean data type can have a value of True or False
hello_bool = True
String data type stores a collection of characters. “Hello
World” is a string
hello_str = “Hello World!”
As well as these main data types, there are sequence types
List data type contains a collection of data in a specific order
hello_list = [“Hello,”, “this”, “is”, “list”]
You could also create the same list in the following way
hello_list = list()
The first line creates an empty list and the following lines use the append function of the list type to add elements to the list.
It can be useful when working with dynamic data such as user input.
Tuple data type, contains a collection immutable data in a specific order.
hello_tuple = (1991, 77)
The data stored in a tuple is immutable because you aren’t able to change values of individual elements in a tuple. However, you can do so in a list.
It will also be useful to know about Python’s dictionary type.
A dictionary is a mapped data type. It stores data in key-value pairs.
hello_dict = { “first_name” : “Safaa”,
“last_name” : “Alaa”,
“eye_colour” : “Brown” }
This means that you access values stored in the dictionary using that value’s corresponding key,
print(hello_dict[“first_name”] + “ “ + hello_dict[“last_name”] + “ has “ + hello_dict[“eye_colour”] + “ eyes.”)
Which is different to how you would do it with a list.
In a list, you would access an element of the list using that element’s index, index it just a number representing the element’s position in the list).
Indexes in Python are zero-based, which means the first element has an index of 0.
print(hello_list[3])
We’ll start by changing the value of the last string in our hello_list and add an exclamation mark to the end.
hello_list[3] += “!”
print(hello_list[3])
In tuple, we can’t change the value of those elements like we just did with the list
print(hello_tuple[0])
Let’s create a sentence using the data in our hello_dict
A tidier way of doing this would be to use Python’s string formatter
print(“{0} {1} has {2} eyes.”.format(hello_dict[“fi rst_name”],
hello_dict[“last_name”],
hello_dict[“eye_colour”]))
“Any text in a Python file that follows a # character will be ignored”
Subscribe for more:
----------------------------------------------------------------------------
SWE.Safaa Al-Hayali - saf3al2a
A variable is a name in source code that is associated with an area in memory that you can use to store data, which is then called upon throughout the code.
We create a variable by writing the name of the variable we want followed by an equal’s sign, which is followed by the value we want to store in the variable.
The data can be one of many types, including:
Integer, that Stores whole numbers
hello_int = 21
Float data type: Stores decimal numbers
hello_float = 1.3
Boolean data type can have a value of True or False
hello_bool = True
String data type stores a collection of characters. “Hello
World” is a string
hello_str = “Hello World!”
As well as these main data types, there are sequence types
List data type contains a collection of data in a specific order
hello_list = [“Hello,”, “this”, “is”, “list”]
You could also create the same list in the following way
hello_list = list()
The first line creates an empty list and the following lines use the append function of the list type to add elements to the list.
It can be useful when working with dynamic data such as user input.
Tuple data type, contains a collection immutable data in a specific order.
hello_tuple = (1991, 77)
The data stored in a tuple is immutable because you aren’t able to change values of individual elements in a tuple. However, you can do so in a list.
It will also be useful to know about Python’s dictionary type.
A dictionary is a mapped data type. It stores data in key-value pairs.
hello_dict = { “first_name” : “Safaa”,
“last_name” : “Alaa”,
“eye_colour” : “Brown” }
This means that you access values stored in the dictionary using that value’s corresponding key,
print(hello_dict[“first_name”] + “ “ + hello_dict[“last_name”] + “ has “ + hello_dict[“eye_colour”] + “ eyes.”)
Which is different to how you would do it with a list.
In a list, you would access an element of the list using that element’s index, index it just a number representing the element’s position in the list).
Indexes in Python are zero-based, which means the first element has an index of 0.
print(hello_list[3])
We’ll start by changing the value of the last string in our hello_list and add an exclamation mark to the end.
hello_list[3] += “!”
print(hello_list[3])
In tuple, we can’t change the value of those elements like we just did with the list
print(hello_tuple[0])
Let’s create a sentence using the data in our hello_dict
A tidier way of doing this would be to use Python’s string formatter
print(“{0} {1} has {2} eyes.”.format(hello_dict[“fi rst_name”],
hello_dict[“last_name”],
hello_dict[“eye_colour”]))
“Any text in a Python file that follows a # character will be ignored”
Subscribe for more:
----------------------------------------------------------------------------
SWE.Safaa Al-Hayali - saf3al2a