filmov
tv
CppCon 2019: Conor Hoekstra, “Algorithm Intuition (part 2 of 2)”
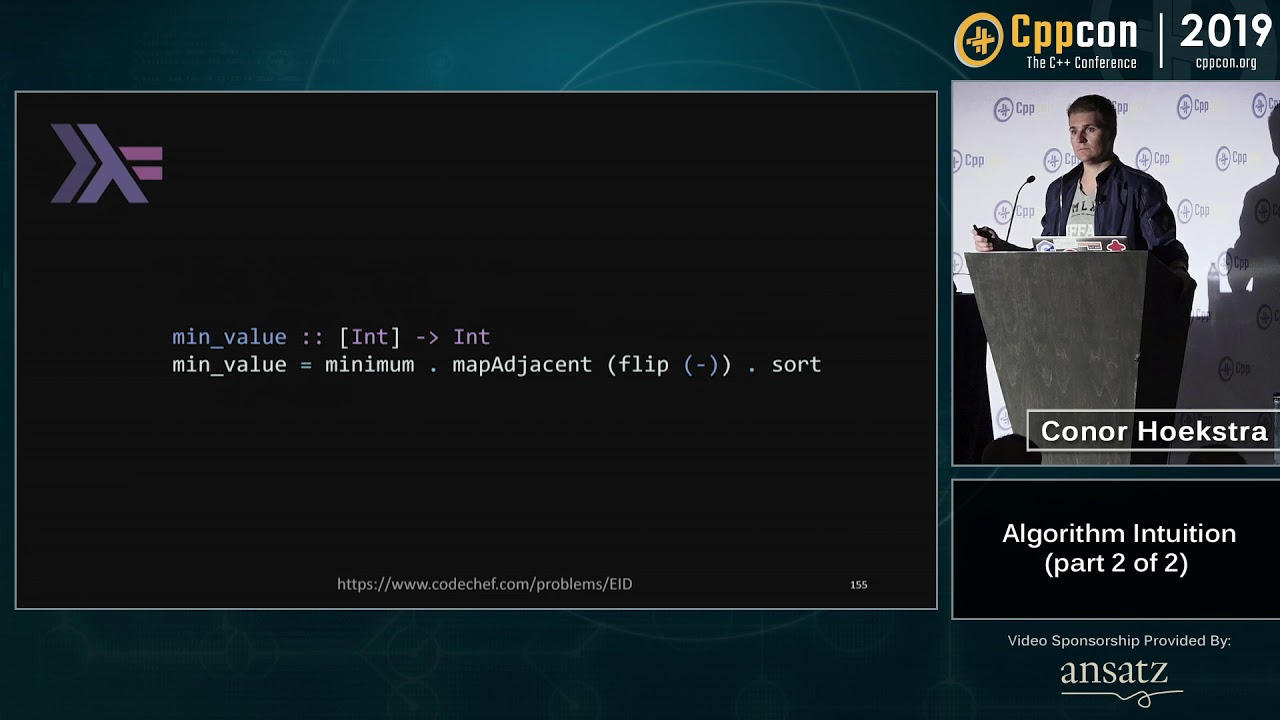
Показать описание
—
—
—
Data structure intuition is something that develops naturally for most software developers. In all languages, we rely heavily on standard containers and collections. Need fast insertion/lookup? Hashmap. Need a sorted data structure that stores unique values? Set. Duplicate values? Multiset. And so on.
However, most software developers don't develop algorithm intuition quite as easily. Algorithms aren't taught as widely as data structures are, and aren't relied on as heavily. This talk aims to introduce some STL algorithms, show how they are commonly used, and show how by developing intuition about them (+ a little help from lambdas), you can unlock their true potential.
—
Conor Hoekstra
Amazon
Software Development Engineer
San Francisco Bay Area
Conor is extremely passionate about programming languages, algorithms and beautiful code. He spent 5 years in Canada working on a large-scale C++ codebase. In 2018, he moved down to Silicon Valley and has spent the last year working for Amazon using C++, Java, Python, Go, Perl and more. He is in the midst of falling in love with Haskell and functional programming. He also has a YouTube channel where he covers solutions to competitive programming problems and more.
—
*-----*
*-----*
—
—
Data structure intuition is something that develops naturally for most software developers. In all languages, we rely heavily on standard containers and collections. Need fast insertion/lookup? Hashmap. Need a sorted data structure that stores unique values? Set. Duplicate values? Multiset. And so on.
However, most software developers don't develop algorithm intuition quite as easily. Algorithms aren't taught as widely as data structures are, and aren't relied on as heavily. This talk aims to introduce some STL algorithms, show how they are commonly used, and show how by developing intuition about them (+ a little help from lambdas), you can unlock their true potential.
—
Conor Hoekstra
Amazon
Software Development Engineer
San Francisco Bay Area
Conor is extremely passionate about programming languages, algorithms and beautiful code. He spent 5 years in Canada working on a large-scale C++ codebase. In 2018, he moved down to Silicon Valley and has spent the last year working for Amazon using C++, Java, Python, Go, Perl and more. He is in the midst of falling in love with Haskell and functional programming. He also has a YouTube channel where he covers solutions to competitive programming problems and more.
—
*-----*
*-----*
Комментарии