filmov
tv
Merge Without Extra Space | 07 July POTD | C++ | Geeks for Geeks Problem of the Day
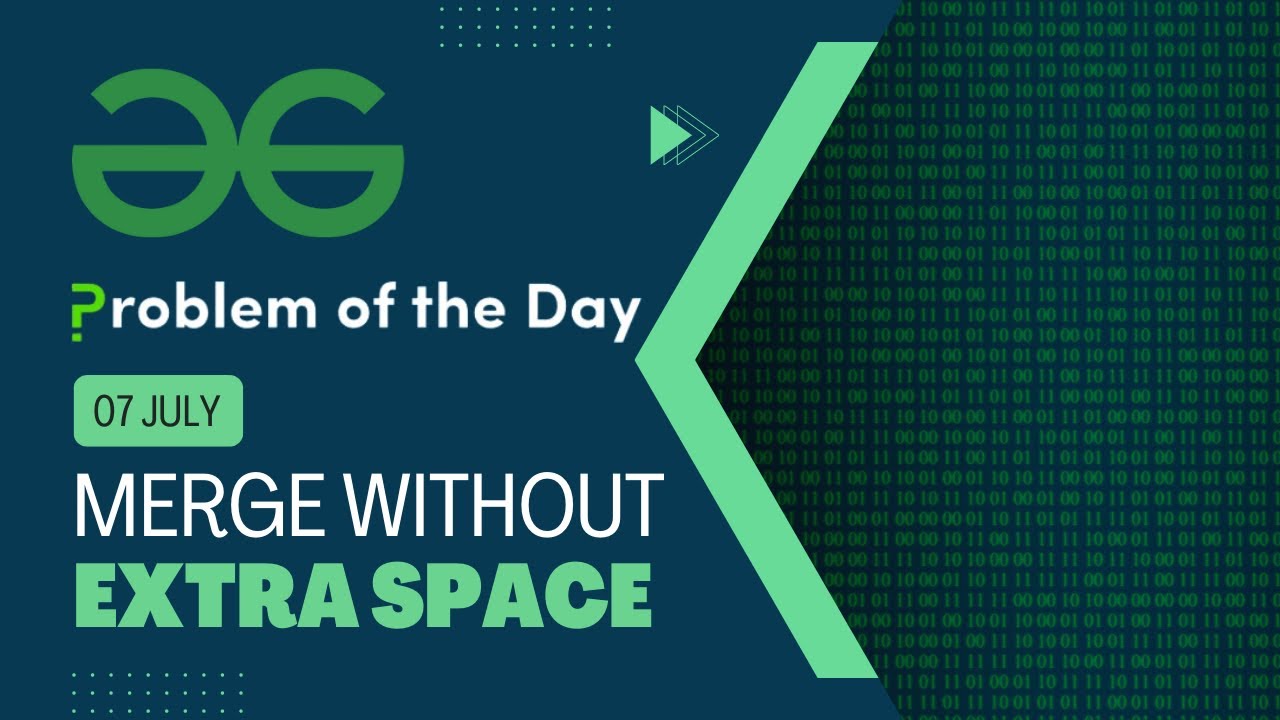
Показать описание
Hi I hope you were able to understand the problem solution. Here are a few links for you to check out.
Subscribe and Keep Coding.
#GFG #POTD #geeksforgeeks #problemoftheday #competetiveprogramming #c++
Subscribe and Keep Coding.
#GFG #POTD #geeksforgeeks #problemoftheday #competetiveprogramming #c++
Merge Sorted Arrays Without Extra Space | 2 Optimal Solution
Merge 2 sorted arrays without extra space
Merge Two Sorted Arrays Without Extra Space
Merge 2 Sorted array without using Extra Space | Love Babbar DSA Sheet Q12 | Arrays
Problem of The Day: 07/07/2023 | Merge Without Extra Space | Abhinav Awasthi
Merge Sorted Array - Leetcode 88 - Python
Merge Without Extra Space | 07 July POTD | C++ | Geeks for Geeks Problem of the Day
Merge two sorted arrays in O(1) space | GFG | C++ and Java | Brute-Better-Optimal
LeetCode 56 - Merge Intervals
Merge Without Extra Space || GFG
Merge Two Sorted Arrays Without Extra Space | Leetcode Problem and Solution
Merge Without Extra Space | easiest solution in hindi | Merge two sorted array | O(1) space
12.Merge two sorted arrays without using extra space | Arrays | GFG Accepted | Very Easy Solution
Merge Two Sorted List without using extra space #python #dsa
Merge Without Extra Space | Must Do Interview Questions | Geeks For Geeks | SDE
Commonly asked Interview Question- Merge Two Sorted Arrays(With and without extra space)
Merge Without Extra Space || GFG SDE Sheet || Problem 52 #coding #sdesheet #gfg #potd
Merge 2 array without extra space || Hard || Geeksforgeeks
Merge Without Extra Space | How to Merge Two Sorted Array Without Using Extra Space|Coder Army Sheet
Merge 2 sorted arrays without using extra space in Python | 450 Question | Love Babbar Sheet
How to merge C and D drive in windows 10/11
Merge without extra space || Geeksforgeeks || DSA cracker sheet
Merge Without Extra Space | in Java | Must-Do Coding Questions | Code With Dinesh
Merge Without Extra Space | Merging two Sorted Arrays | Hindi Explain | GFG | Problem Solving | MLSA
Комментарии