filmov
tv
How to Properly Use a Returned int * Array from C in Python with CFFI
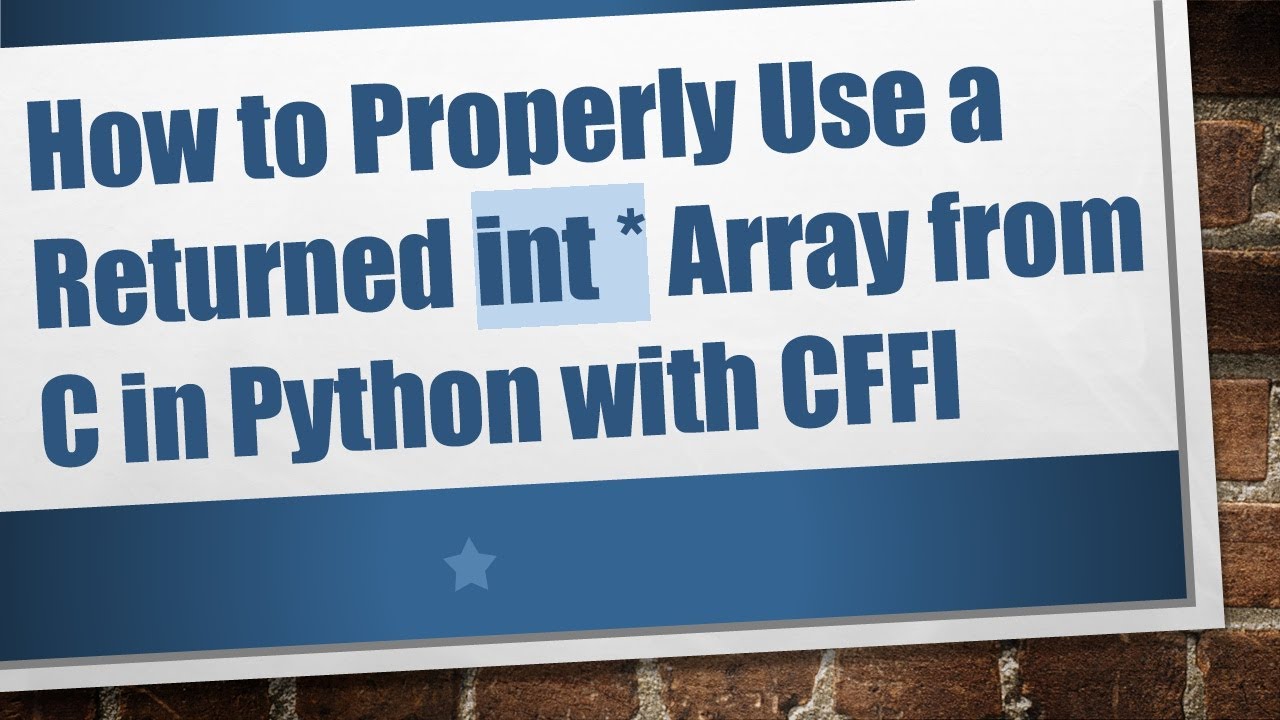
Показать описание
Learn how to efficiently handle arrays returned from C functions in Python using CFFI, including memory management and type casting.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: using an int * (array) return value in python via cffi?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Introduction
When working with C extensions in Python, especially using C Foreign Function Interface (CFFI), handling arrays can often be a source of confusion. One common issue arises when you need to retrieve and use an array of integers returned by a C function. This guide will guide you through the process of returning an int * from C and effectively using it in your Python code.
The Problem
Consider a scenario where you have a C function defined as follows:
[[See Video to Reveal this Text or Code Snippet]]
In Python, you can call this function using:
[[See Video to Reveal this Text or Code Snippet]]
However, the challenge comes when trying to utilize the ret variable, which holds a pointer to an array of integers. The main question here is: How can you convert this pointer into a usable Python array or list?
The Solution
To work with the array returned by your C function using CFFI, you can take several approaches. Below, I outline the basic method as well as some optimizations for handling memory and improving performance.
Accessing the Returned Array
When the C function returns an int *, you can treat it as an array in Python directly. Here’s how you can extract integers from it to create a Python list:
[[See Video to Reveal this Text or Code Snippet]]
This comprehension allows you to iterate over the first five elements of the array and store them in a list.
Memory Management
Since you are using malloc() in the C function, it’s crucial to free the allocated memory once you are done with it to avoid memory leaks. You can do this in Python by adding the following declaration in your CFFI setup:
[[See Video to Reveal this Text or Code Snippet]]
And then, after you have finished using the array:
[[See Video to Reveal this Text or Code Snippet]]
Advanced Techniques
For more advanced usage, there are additional functions in CFFI that can help streamline your code.
Type Casting: You can cast the pointer directly to an array type if you wish to work with it as a fixed-length array:
[[See Video to Reveal this Text or Code Snippet]]
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Handling a C function's returned int * array in Python can be straightforward when using CFFI, provided you keep memory management in mind. With this guide, you can efficiently retrieve integer arrays, manage memory safely, and utilize advanced techniques to enhance your performance. These strategies will not only help avoid confusion but will also make your code cleaner and more efficient.
By understanding these concepts, you can make the most out of the interoperability between C and Python using CFFI, tapping into the performance benefits that C has to offer while enjoying the ease of Python.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: using an int * (array) return value in python via cffi?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Introduction
When working with C extensions in Python, especially using C Foreign Function Interface (CFFI), handling arrays can often be a source of confusion. One common issue arises when you need to retrieve and use an array of integers returned by a C function. This guide will guide you through the process of returning an int * from C and effectively using it in your Python code.
The Problem
Consider a scenario where you have a C function defined as follows:
[[See Video to Reveal this Text or Code Snippet]]
In Python, you can call this function using:
[[See Video to Reveal this Text or Code Snippet]]
However, the challenge comes when trying to utilize the ret variable, which holds a pointer to an array of integers. The main question here is: How can you convert this pointer into a usable Python array or list?
The Solution
To work with the array returned by your C function using CFFI, you can take several approaches. Below, I outline the basic method as well as some optimizations for handling memory and improving performance.
Accessing the Returned Array
When the C function returns an int *, you can treat it as an array in Python directly. Here’s how you can extract integers from it to create a Python list:
[[See Video to Reveal this Text or Code Snippet]]
This comprehension allows you to iterate over the first five elements of the array and store them in a list.
Memory Management
Since you are using malloc() in the C function, it’s crucial to free the allocated memory once you are done with it to avoid memory leaks. You can do this in Python by adding the following declaration in your CFFI setup:
[[See Video to Reveal this Text or Code Snippet]]
And then, after you have finished using the array:
[[See Video to Reveal this Text or Code Snippet]]
Advanced Techniques
For more advanced usage, there are additional functions in CFFI that can help streamline your code.
Type Casting: You can cast the pointer directly to an array type if you wish to work with it as a fixed-length array:
[[See Video to Reveal this Text or Code Snippet]]
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Handling a C function's returned int * array in Python can be straightforward when using CFFI, provided you keep memory management in mind. With this guide, you can efficiently retrieve integer arrays, manage memory safely, and utilize advanced techniques to enhance your performance. These strategies will not only help avoid confusion but will also make your code cleaner and more efficient.
By understanding these concepts, you can make the most out of the interoperability between C and Python using CFFI, tapping into the performance benefits that C has to offer while enjoying the ease of Python.